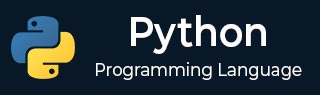
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 代码块
- Python - try-finally 代码块
- Python - 抛出异常
- Python - 异常链接
- Python - 嵌套 try 代码块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
使用 Python 开发的实时世界时间应用程序
实时世界时间项目是一个 GUI 应用程序,使用基本的 Python 和名为 Tkinter 的库进行编码。该选项提供了一个输入大陆和国家以显示当前本地时间并在地图上打开 Google 地图显示该国家/地区位置的功能。
所需库
首先,确保已安装以下库:
- **Tkinter** - 它与 Python 一起提供,用于定义和设计图形用户界面应用程序。
- **pytz** - 此库用于时区管理。
- **webbrowser** - 此库用于打开 URL 的网页浏览器。
安装
可以使用 PIP 或通过直接命令提示符进行安装。以下是安装所需库的代码语句。
要安装 tkinter,请使用以下命令:
pip install tkinter
要安装 pytz,请使用以下命令:
pip install pytz
您可以从命令提示符下官方文档获取 webbrowser 模块,如下所示:
python -m webbrowser -t "https://pythonlang.cn"
创建实时世界时间应用程序的步骤
步骤 1:导入必要的库
使用以下代码语句导入所需的库:
- 导入 datetime 用于处理日期和时间。
- 导入 pytz 用于时区转换。
- 导入 tkinter 和 ttk 用于 GUI 元素。
- 导入 webbrowser 以打开 URL。
步骤 2:初始化 Tkinter 窗口
要**初始化 tkinter 窗口**:
- 使用 Tk() 创建一个窗口。
- 设置窗口的标题和大小。
步骤 3:定义函数
以下是用于在项目中完成不同任务的函数:
- **open_map()** - 此函数根据所选国家/地区打开 Google 地图。
- **update_map_and_time()** - 此函数更新显示的时间并打开 Google 地图。
- **update_countries(event)** - 此函数在选择大洲时更新国家/地区列表。
步骤 4:创建下拉菜单
您必须创建以下下拉菜单:
- 选择大陆。
- 国家/地区选择,根据大陆填充。
步骤 5:显示当前时间
要显示当前时间,请使用标签显示所选国家/地区的本地时间。
步骤 6:将函数绑定到事件
为以下内容绑定函数:
- 大陆下拉菜单以更新国家/地区列表。
- 国家/地区下拉菜单以更新时间和地图。
步骤 7:运行应用程序
使用 root.mainloop() 启动 Tkinter 事件循环。
创建实时世界时间应用程序的代码
from datetime import datetime import pytz from tkinter import * from tkinter import ttk import webbrowser root = Tk() root.title("OPEN World") root.geometry("700x400") # Function to open Google Maps with the selected location def open_map(): map_url = f"https://www.google.com/maps/search/?api=1&query={country_var.get().replace(' ', '+')}" webbrowser.open(map_url) # Function to update the map and time display def update_map_and_time(): continent = continent_var.get() country = country_var.get() if continent == 'Asia': if country == 'India': timezone = 'Asia/Kolkata' elif country == 'China': timezone = 'Asia/Shanghai' elif country == 'Japan': timezone = 'Asia/Tokyo' elif country == 'Pakistan': timezone = 'Asia/Karachi' elif country == 'Bangladesh': timezone = 'Asia/Dhaka' else: return elif continent == 'Australia': timezone = 'Australia/Victoria' elif continent == 'Africa': if country == 'Nigeria': timezone = 'Africa/Lagos' elif country == 'Algeria': timezone = 'Africa/Algiers' else: return elif continent == 'America': if country == 'USA (West)': timezone = 'America/Los_Angeles' elif country == 'Argentina': timezone = 'America/Argentina/Buenos_Aires' elif country == 'Canada': timezone = 'America/Toronto' elif country == 'Brazil': timezone = 'America/Sao_Paulo' else: return elif continent == 'Europe': if country == 'UK': timezone = 'Europe/London' elif country == 'Portugal': timezone = 'Europe/Lisbon' elif country == 'Italy': timezone = 'Europe/Rome' elif country == 'Spain': timezone = 'Europe/Madrid' else: return # Update the time home = pytz.timezone(timezone) local_time = datetime.now(home) current_time = local_time.strftime("%H:%M:%S") clock_label.config(text=current_time) # Open Google Maps in the web browser open_map() # Function to update the country options based on selected continent def update_countries(event): continent = continent_var.get() if continent == 'Asia': countries = ['India', 'China', 'Japan', 'Pakistan', 'Bangladesh'] elif continent == 'Australia': countries = ['Australia'] elif continent == 'Africa': countries = ['Nigeria', 'Algeria'] elif continent == 'America': countries = ['USA (West)', 'Argentina', 'Canada', 'Brazil'] elif continent == 'Europe': countries = ['UK', 'Portugal', 'Italy', 'Spain'] else: countries = [] country_menu['values'] = countries country_var.set('') # Reset country selection # Continent Selection continent_var = StringVar() continent_var.set('Asia') # Default value continent_label = Label(root, text="Select Continent:", font=("Arial", 14)) continent_label.pack(pady=10) continent_menu = ttk.Combobox(root, textvariable=continent_var, font=("Arial", 12), state="readonly", width=20) continent_menu['values'] = ['Asia', 'Australia', 'Africa', 'America', 'Europe'] continent_menu.pack(pady=10) continent_menu.bind('<<ComboboxSelected>>', update_countries) # Country Selection country_var = StringVar() country_label = Label(root, text="Select Country:", font=("Arial", 14)) country_label.pack(pady=10) country_menu = ttk.Combobox(root, textvariable=country_var, font=("Arial", 12), state="readonly", width=20) country_menu.pack(pady=10) country_menu.bind('<<ComboboxSelected>>', lambda event: update_map_and_time()) # Time Display clock_label = Label(root, font=("Arial", 25, "bold")) clock_label.pack(pady=20) root.mainloop()
输出
**GUI 窗口** - 将出现一个标题为“OPEN World”的窗口。
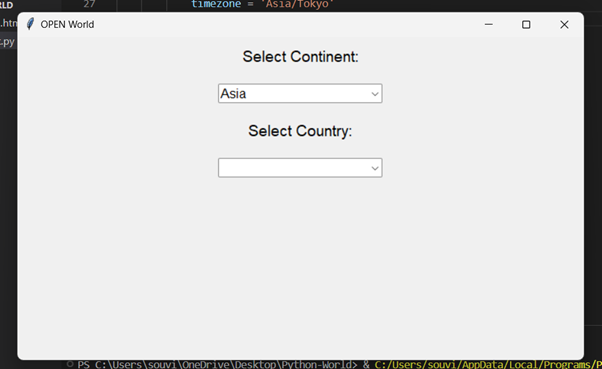
**大陆选择** - 用户从列表中选择一个大陆
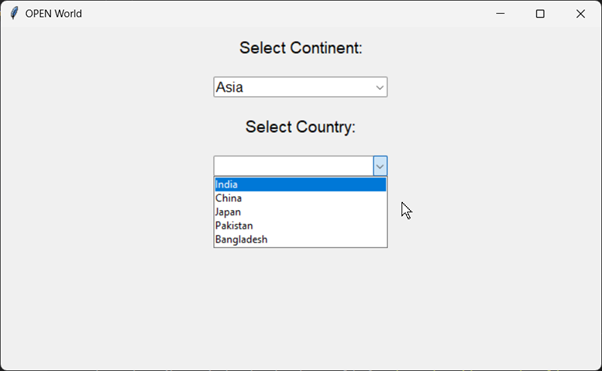
我们点击亚洲大陆,您可以显示四个国家/地区,现在我们点击印度
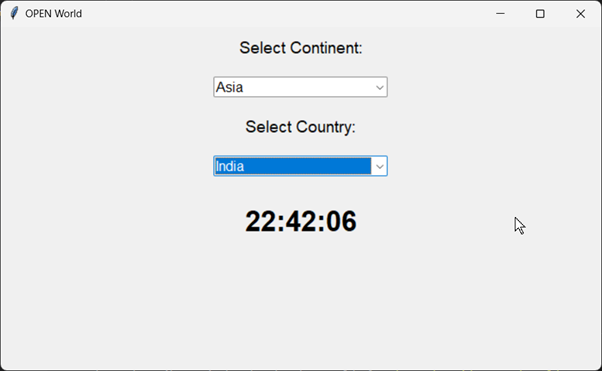
它显示印度的实时时间,并且还会自动为 Google 中的此位置打开网络浏览器
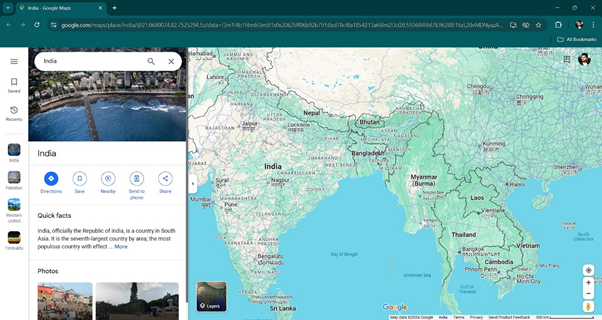
现在我们点击欧洲的葡萄牙。
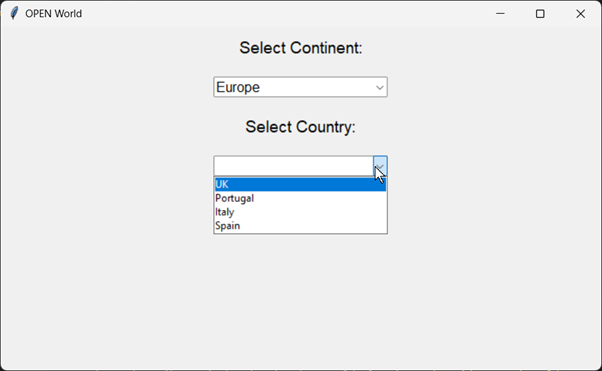
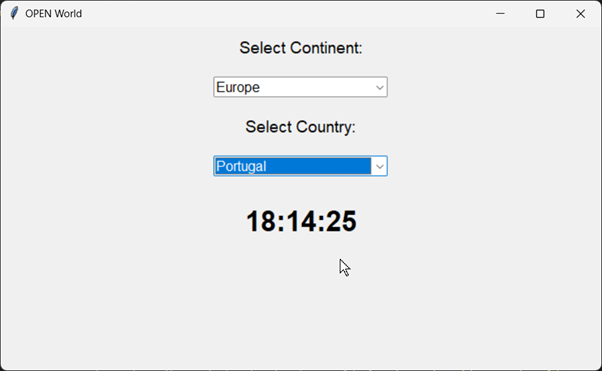
它也会打开谷歌地图。
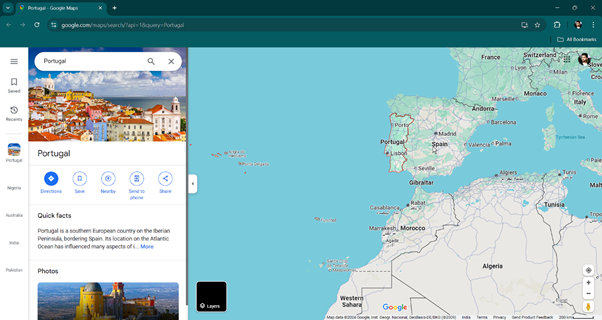
**时间显示** - 显示所选国家/地区的当前本地时间。
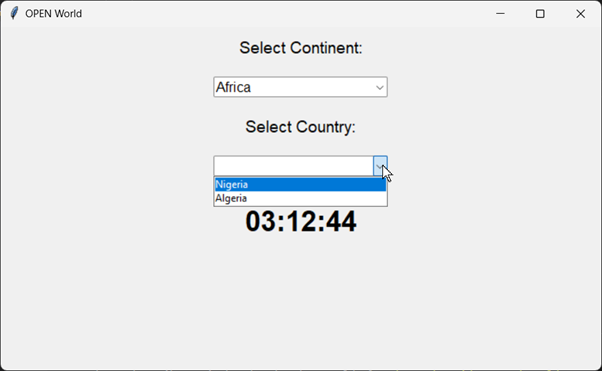
**Google 地图** - 所选国家/地区的位置在 Google 地图中打开
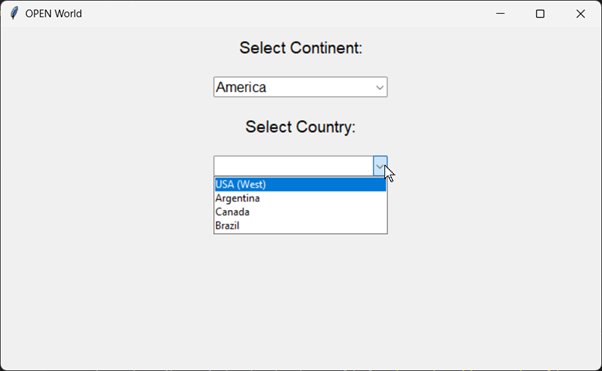
如果您想要更多国家/地区,可以在代码中添加您的国家/地区。
python_reference.htm
广告