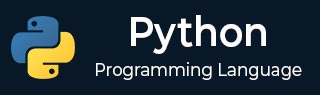
- Python基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python控制语句
- Python - 控制流
- Python - 决策
- Python - if语句
- Python - if else
- Python - 嵌套if
- Python - match-case语句
- Python - 循环
- Python - for循环
- Python - for-else循环
- Python - while循环
- Python - break语句
- Python - continue语句
- Python - pass语句
- Python - 嵌套循环
- Python函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS文件/目录方法
- Python - OS路径方法
- 面向对象编程
- Python - OOPs概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except块
- Python - try-finally块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套try块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 合并线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL处理
- Python - 泛型
- Python库
- NumPy教程
- Pandas教程
- SciPy教程
- Matplotlib教程
- Django教程
- OpenCV教程
- Python杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI编程
- Python - XML处理
- Python - GUI编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类的元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python实用资源
- Python - 问答
- Python - 在线测试
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 资源
- Python - 讨论
- Python编译器
- NumPy编译器
- Matplotlib编译器
- SciPy编译器
使用Python的猜数字游戏
猜数字是一款经典的破译密码游戏,玩家需要在一定次数的尝试内猜出秘密代码。在本文中,我们将探讨如何使用不同的方法(从基本到高级)在Python中实现猜数字游戏。我们将介绍每种方法中使用的逻辑、代码和概念解释。
方法1:基本实现
在猜数字游戏的基本实现中,计算机随机生成一个秘密代码,玩家必须猜出该代码。计算机在每次猜测后提供反馈,指示有多少位数字正确且位置正确(表示为“bulls”),以及有多少位数字正确但位置错误(表示为“cows”)。
示例
以下是基本实现代码:
import random # Function to generate a random 4-digit code def generate_code(): return [random.randint(0, 9) for _ in range(4)] # Function to compare the guess with the code def check_guess(code, guess): bulls = sum([1 for i in range(4) if code[i] == guess[i]]) cows = sum([1 for i in range(4) if guess[i] in code and code[i] != guess[i]]) return bulls, cows # Main game loop def mastermind_basic(): code = generate_code() attempts = 10 print("Welcome to Mastermind!") print("Guess the 4-digit code. You have 10 attempts.") while attempts > 0: guess = list(map(int, input("Enter your guess: ").strip())) bulls, cows = check_guess(code, guess) print(f"Bulls: {bulls}, Cows: {cows}") if bulls == 4: print("Congratulations! You've cracked the code!") break attempts -= 1 if attempts == 0: print(f"Game Over! The code was: {''.join(map(str, code))}") # Run the basic game mastermind_basic()
输出
Welcome to Mastermind! Guess the 4-digit code. You have 10 attempts. Enter your guess: 1234 Bulls: 1, Cows: 2 Enter your guess: 5678 Bulls: 0, Cows: 1 Enter your guess: 9101 Bulls: 2, Cows: 0 ... Congratulations! You've cracked the code!
解释
- “generate_code”函数使用random模块生成一个随机的4位数字代码。
- “check_guess”函数比较玩家的猜测与秘密代码,并返回bulls和cows的数量。
- 主游戏循环运行10次尝试,玩家输入他们的猜测,程序提供反馈。
方法2:带有输入验证的高级实现
在这个高级版本中,我们添加输入验证以确保玩家输入有效的4位数字。我们还将引入一个函数来检查代码中是否包含唯一数字,使游戏更具挑战性。
示例
以下是高级实现代码:
import random # Function to generate a random 4-digit code with unique digits def generate_unique_code(): digits = list(range(10)) random.shuffle(digits) return digits[:4] # Function to validate user input def validate_input(user_input): return user_input.isdigit() and len(user_input) == 4 # Function to compare the guess with the code def check_guess(code, guess): bulls = sum([1 for i in range(4) if code[i] == guess[i]]) cows = sum([1 for i in range(4) if guess[i] in code and code[i] != guess[i]]) return bulls, cows # Main game loop def mastermind_advanced(): code = generate_unique_code() attempts = 10 print("Welcome to Mastermind!") print("Guess the 4-digit code with unique digits. You have 10 attempts.") while attempts > 0: guess = input("Enter your guess: ").strip() if not validate_input(guess): print("Invalid input! Please enter a 4-digit number.") continue guess = list(map(int, guess)) bulls, cows = check_guess(code, guess) print(f"Bulls: {bulls}, Cows: {cows}") if bulls == 4: print("Congratulations! You've cracked the code!") break attempts -= 1 if attempts == 0: print(f"Game Over! The code was: {''.join(map(str, code))}") # Run the advanced game mastermind_advanced()
输出
Welcome to Mastermind! Guess the 4-digit code with unique digits. You have 10 attempts. Enter your guess: 1123 Invalid input! Please enter a 4-digit number. Enter your guess: 3456 Bulls: 0, Cows: 2 Enter your guess: 7890 Bulls: 1, Cows: 1 ... Congratulations! You've cracked the code!
解释
- “generate_unique_code”函数通过对数字列表进行洗牌并选择前四个数字来生成一个包含唯一数字的4位数字代码。
- “validate_input”函数检查玩家的输入是否为4位数字。
- 游戏循环现在包括输入验证,如果输入无效,则提示玩家重新输入猜测。
结论
猜数字游戏是练习Python编程的一种有趣且具有挑战性的方式。在本文中,我们探讨了两种不同的游戏实现方法,从基本版本到具有输入验证和唯一数字的更高级版本。这些示例为进一步增强提供了坚实的基础,例如添加图形用户界面(GUI)或实现AI来玩游戏。
python_projects_from_basic_to_advanced.htm
广告