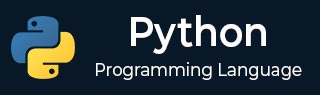
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 合并线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python math.atan2() 方法
Python 的 math.atan2() 方法返回 atan(y / x) 的值(以弧度表示)。换句话说,此方法将笛卡尔坐标对 (x, y) 转换为极坐标对 (r, θ) 并返回 θ 值。
此方法仅接受浮点值作为参数;如果传递给此方法的值不是浮点值,则会引发 TypeError。
注意 - 此函数无法直接访问,因此我们需要导入 math 模块,然后才能使用 math 静态对象调用此函数。
语法
以下是 Python math.atan2() 方法的语法:
math.atan2(y, x)
参数
x, y - 必须是浮点类型的数值。
返回值
此方法返回 atan(y / x) 的值(以弧度表示)。
示例
以下示例演示了 Python math.atan2() 方法的使用。在这里,我们创建了两个包含两个浮点值的 对象,并将它们作为参数传递给此方法。获得的返回值必须以弧度表示。
import math # Create two objects of floating-point numbers x = 0.6 y = 1.2 # Calculate the atan(y/x) value theta = math.atan2(y, x) # Print the theta value print("The theta value is calculated as:", theta)
运行上述程序时,会产生以下结果:
The theta value is calculated as: 1.1071487177940904
示例
如果我们将负值作为参数传递给此方法,则将返回这些矩形坐标所包围的角度。
import math # Create two objects of floating-point numbers x = -1.6 y = -3.5 # Calculate the atan(y/x) value theta = math.atan2(y, x) # Print the theta value print("The theta value is calculated as:", theta)
如果我们编译并运行
The theta value is calculated as: -1.999574354240913
示例
在下面的示例中,我们创建了两个列表对象 x、y。使用循环语句,我们试图从列表中找到对应 x 和 y 值的 θ 值。
import math # Create two lists of floating-point numbers x = [1, 2, 3, 4] y = [5, 6, 7, 8] # Calculate the atan(y/x) value of all objects in the list for n in range(0, len(x)): theta = math.atan2(y[n], x[n]) print("The theta value of", y[n], "and", x[n], "is calculated as:", theta)
编译并运行上面的程序后,输出显示为:
The theta value of 5 and 1 is calculated as: 1.373400766945016 The theta value of 6 and 2 is calculated as: 1.2490457723982544 The theta value of 7 and 3 is calculated as: 1.1659045405098132 The theta value of 8 and 4 is calculated as: 1.1071487177940904
示例
如果直接将上面的列表作为参数传递给该方法,则会引发 TypeError。因此,我们使用循环语句访问其中的浮点对象。
import math # Create two lists of floating-point numbers x = [1, 2, 3, 4] y = [5, 6, 7, 8] # Calculate the atan(y/x) value of all objects in the list theta = math.atan2(y, x) print("The theta value is calculated as:", theta)
如果我们编译并运行给定的程序,则会引发如下 TypeError:
Traceback (most recent call last): File "main.py", line 8, in <module> theta = math.atan2(y, x) TypeError: must be real number, not list
python_maths.htm
广告