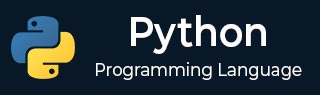
- Python 基础
- Python - 首页
- Python - 概览
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python math.degrees() 方法
Python 的 math.degrees() 方法用于将弧度转换为角度。通常,角度用三种单位来测量:转数、弧度和角度。然而,在三角学中,测量角度的常用方法是用弧度或角度。因此,能够将这些度量单位从一个转换为另一个变得很重要。
degrees() 方法接收一个弧度值作为参数,并将其转换为相应角度。
注意 - 此函数无法直接访问,因此我们需要导入 math 模块,然后我们需要使用 math 静态对象来调用此函数。
语法
以下是 Python math.degrees() 方法的语法:
math.degrees(x)
参数
x - 必须是数值。
返回值
此方法返回角度的度数值。
示例
以下示例显示了 Python math.degrees() 方法的用法。在这里,我们将以弧度为单位的角度“pi”作为参数传递给此方法。预期将其转换为相应的角度。
import math # Take the angle in radians x = 3.14 y = -3.14 # Convert it into degrees using math.degrees() function deg_x = math.degrees(x) deg_y = math.degrees(y) # Display the degrees print("The degrees value of x is:", deg_x) print("The degrees value of y is:", deg_y)
当我们运行上述程序时,它会产生以下结果:
The degrees value of x is: 179.9087476710785 The degrees value of y is: -179.9087476710785
示例
但是,如果我们将无效数字(或 NaN)作为参数传递给该方法,则返回值也将无效(或 NaN)。
import math # Take the nan value in float x = float("nan") # Convert it into degrees using math.degrees() function deg = math.degrees(x) # Display the degrees print("The degrees value of x is:", deg)
如果我们编译并运行给定的程序,则输出将显示如下:
The degrees value of x is: nan
示例
此方法仅接受浮点值作为其参数。如果将类似于数字序列的对象作为其参数传递,则该方法会引发 TypeError。
在以下示例中,我们创建一个包含一系列弧度角的列表对象。然后我们将此列表作为参数传递给此方法。即使列表包含以弧度为单位的角度,该方法也会返回 TypeError。
import math # Take the angle list in radians x = [1, 2, 3, 4, 5] # Convert it into degrees using math.degrees() function deg = math.degrees(x) # Display the degrees print("The degrees value of the list is:", deg)
Traceback (most recent call last): File "main.py", line 7, indeg = math.degrees(x) TypeError: must be real number, not list
示例
为了使用此方法将序列中的对象转换为度数,我们必须每次调用此方法时都将序列中的每个值作为参数传递。因此,我们可以使用循环语句来迭代所述可迭代对象。
import math # Take the angle list in radians x = [1, 2, 3, 4, 5] for n in range (0, len(x)): # Convert it into degrees using math.degrees() function deg = math.degrees(x[n]) # Display the degrees print("The degree value of", x[n], "is:", deg)
编译并运行上述程序,以产生以下结果:
The degree value of 1 is: 57.29577951308232 The degree value of 2 is: 114.59155902616465 The degree value of 3 is: 171.88733853924697 The degree value of 4 is: 229.1831180523293 The degree value of 5 is: 286.4788975654116
python_maths.htm
广告