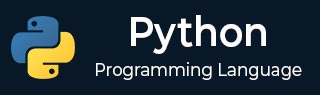
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 对列表进行排序
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 对数组进行排序
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python os.chmod() 方法
Python os.chmod() 方法将路径的模式更改为指定的数字模式。模式可以取以下值之一或它们的按位或组合 -
stat.S_ISUID - 设置用户 ID 以执行。
stat.S_ISGID - 设置组 ID 以执行。
stat.S_ENFMT - 强制记录锁定。
stat.S_ISVTX - 执行后保存文本图像。
stat.S_IREAD - 由所有者读取。
stat.S_IWRITE - 由所有者写入。
stat.S_IEXEC - 由所有者执行。
stat.S_IRWXU - 由所有者读取、写入和执行。
stat.S_IRUSR - 由所有者读取。
stat.S_IWUSR - 由所有者写入。
stat.S_IXUSR - 由所有者执行。
stat.S_IRWXG - 由组读取、写入和执行。
stat.S_IRGRP - 由组读取。
stat.S_IWGRP - 由组写入。
stat.S_IXGRP - 由组执行。
stat.S_IRWXO - 由其他人读取、写入和执行。
stat.S_IROTH - 由其他人读取。
stat.S_IWOTH - 由其他人写入。
stat.S_IXOTH - 由其他人执行。
语法
以下是Python os.chmod() 方法的语法 -
os.chmod(path, mode);
参数
path - 这是要设置模式的路径。
mode - 这可以取上述值之一或它们的按位或组合。
返回值
此方法不返回值。
示例 1
以下示例显示了 Python os.chmod() 方法的用法。在这里,我们首先将文件设置为仅由组执行,方法是将 stat.S_IXGRP 作为模式参数传递给该方法。然后,我们将 stat.S_IWOTH 作为模式参数传递给该方法。这指定只有其他人才能写入该文件。
import os, sys, stat # Assuming /tmp/foo.txt exists, Set a file execute by the group. os.chmod("/tmp/foo.txt", stat.S_IXGRP) # Set a file write by others. os.chmod("/tmp/foo.txt", stat.S_IWOTH) print ("Changed mode successfully!!")
当我们运行上述程序时,它会产生以下结果 -
Changed mode successfully!!
示例 2
在这里,我们首先将文件设置为仅由组写入。这是通过将 stat.S_IWGRP 作为模式参数传递给该方法来完成的。然后,我们将 stat.S_IXGRP 作为模式参数传递给该方法。这指定只有组才能执行该文件。
# importing the libraries import os import sys import stat # Setting the given file written by the group. os.chmod("Code.txt", stat.S_IWGRP) print("This file can only be written by the group") # Setting the given file executed by the group. os.chmod("Code.txt", stat.S_IXGRP) print("Now the file can only be executed by the group.")
在执行上述代码时,我们得到以下输出 -
This file can only be written by the group Now the file can only be executed by the group.
示例 3
在这里,当我们使用 os.chmod() 方法时,我们在设置权限之前编写了 0o。它表示八进制整数。文件权限设置为 755,这意味着所有者可以读取、写入和搜索;其他人和组只能在文件中搜索。
import os file = "code.txt" os.chmod(file, 0o755) stat = os.stat(file) mode = oct(stat.st_mode)[-4:] print('The mode is:',mode)
以下是上述代码的输出 -
The mode is: 0666