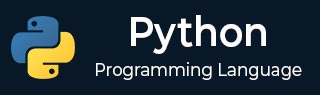
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 结束线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python 和关键词
Python 的 and 关键词是逻辑运算符之一。当两个条件都为真时,结果为 True。它区分大小写。当我们提供一个操作数时,它将导致 SyntaxError。
and 关键词可以用于条件语句、循环、函数中来检查条件是 True 还是 False。它不能直接用于两个数值之间,因为它会将两个值都视为 True 值。
用法
以下是 Python and 关键词的用法:
condition1 and condition2
这里,condition1 和 condition2 可以是任何数值条件。
让我们假设 A 和 B 是操作数,当 A 和 B 都为 True 时,结果为 True。如果任何一个操作数,A 或 B 为 False,结果为 False。以下是 and 关键词的真值表:
A | B | A and B |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
示例
这是一个 Python and 关键词的基本示例:
condition1=True condition2=True result_1=condition1 and condition2 print("The Result Of ",condition1,"And",condition2,":",result_1) operand3=1 operand4=0 result_2=operand3 and operand4 print("The Result Of ",operand3,"And",operand4,":",result_2)
输出
以下是上述代码的输出:
The Result Of True And True : True The Result Of 1 And 0 : 0
在 if-else 语句中使用 and 关键词
and 关键词可以用于 if-else 块中来检查条件结果是否为 True。如果两个给定条件的结果都为 True,则执行 if 块,否则执行 else 块:
示例
让我们通过以下示例来了解在 if-else 中使用 and 关键词:
var1=54 var2=24 var3=12 if var1 > var2 and var1 < var3: print("Both The Conditions Are True") else: print("One Of the Condition is True")
输出
以下是上述代码的输出:
One Of the Condition is True
在循环中使用 and 关键词
and 关键词也用于循环中来检查给定的条件语句,如果条件结果为 True,则执行该块。
示例
在下面的示例中,我们在 while 循环中使用 and 关键词:
list1=[] x=2 while x<25 and True: if x%2==0: list1.append(x) x=x+1 print("We have appended the list1 with the even number below 25 :",list1)
输出
以下是上述代码的输出:
We have appended the list1 with the even number below 25 : [2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24]
在函数中使用 and 关键词
and关键字也用于函数中。如果给定的条件满足,则返回True,否则返回False。
示例
这是一个在函数中使用and关键字的示例:
def num(x): if x>5 and x<100: return True else: return False var1=57 result_1=num(var1) print(var1,"is between",5,"and",100,"True/False :",result_1) var2=600 result_2=num(var2) print(var2,"is between",5,"and",100,"True/False :",result_2)
输出
以下是上述代码的输出:
57 is between 5 and 100 True/False : True 600 is between 5 and 100 True/False : False
在数值中使用and关键字
我们不能使用数值作为and关键字的操作数。它会将两个操作数都视为True,并返回第二个操作数。如果任何一个操作数为零,则结果为零。
示例
这是一个在数值之间使用and关键字的示例:
var1=60 var2=100 result_1= var1 and var2 print("The Result of Two numeric values",var1,"and",var2,":",result_1) var3=0 var4=18 result_2= var3 and var4 print("The Result of Two numeric values",var3,"and",var4,":",result_2)
输出
以下是上述代码的输出:
The Result of Two numeric values 60 and 100 : 100 The Result of Two numeric values 0 and 18 : 0
python_keywords.htm
广告