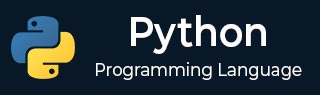
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python collections.ChainMap
在 Python 中,ChainMap 类用于将多个字典或映射转换为单个单元。如果未指定任何映射,则提供单个空字典,以便新的链始终至少具有一个映射。
ChainMap() 类在 Collections 模块中定义。它通常比创建新字典快得多。
语法
以下是 Python ChainMap() 的语法:
collections.ChainMap(iterable1, iterable2, iterable3)
参数
此数据类型接受迭代器作为参数。
返回值
此数据类型返回一个新的 ChainMap 对象,该对象映射多个可迭代对象。
示例
以下是 Python ChainMap() 的基本示例:
# Python program to demonstrate # ChainMap from collections import ChainMap d1 = {'one': 1, 'two': 2} d2 = {'three': 3, 'four': 4} d3 = {'five': 5, 'six': 6} # Defining the chainmap c = ChainMap(d1, d2, d3) print(c)
以下是上述代码的输出:
ChainMap({'one': 1, 'two': 2}, {'three': 3, 'four': 4}, {'five': 5, 'six': 6})
使用 ChainMap 模拟嵌套作用域
ChainMap() 类可用于模拟嵌套作用域,在模板中很有用。它允许我们将多个字典或映射组合成一个单元,因此我们可以像它们是一个一样在所有字典中搜索元素。ChainMap 会按优先级顺序检查每个字典,并在找到匹配项后立即停止,而不是单独搜索每个字典。
示例
在以下示例中,ChainMap() 与嵌套作用域一起使用:
from collections import ChainMap default_settings = {'theme': 'dark', 'font': 'Arial', 'size': 12} user_settings = {'theme': 'light', 'size': 14} # ChainMap gives priority to the first dictionary (user_settings) settings = ChainMap(user_settings, default_settings) print(settings['theme']) print(settings['font'])
以下是上述代码的输出:
light Arial
ChainMap 中的基础映射
在 ChainMap 类中,基础映射(字典)存储为列表。此列表可通过 maps 属性访问,该属性允许您直接查看或修改 ChainMap 管理的字典。
示例
这是一个在 ChainMap() 中使用基础映射的示例:
from collections import ChainMap # Two dictionaries dict1 = {'a': 1, 'b': 2} dict2 = {'b': 3, 'c': 4} # Create a ChainMap combined = ChainMap(dict1, dict2) print(combined['b']) # Accessing the maps attribute print(combined.maps) # Updating the order of dictionaries in the ChainMap combined.maps = [dict2, dict1] print(combined['b'])
以下是上述代码的输出:
2 [{'a': 1, 'b': 2}, {'b': 3, 'c': 4}] 3
使用 ChainMap 合并多个列表
ChainMap() 还可以用于将多个列表转换为单个单元。
示例
以下是使用 ChainMap() 与列表的示例:
from collections import ChainMap list1 = ['a','b','c','d'] list2 = [1, 2, 3, 4] list3 = ['one','two','three'] chain_list = ChainMap(list1, list2, list3) print(chain_list)
以下是上述代码的输出:
ChainMap(['a', 'b', 'c', 'd'], [1, 2, 3, 4], ['one', 'two', 'three'])
ChainMap 中的访问操作
我们可以使用 keys() 函数访问 ChainMap 中所有字典的所有键。values() 函数返回 ChainMap 中所有字典的所有值。maps() 函数用于显示 ChainMap 中所有字典的键及其对应值。
示例
# keys(), values() and maps # importing collections for ChainMap operations import collections # initializing dictionaries dic1 = { 'Program1' : 'Python', 'Program2' : 'HTML' } dic2 = { 'Program3' : ' Java', 'Program4' : 'C' } # initializing ChainMap chain = collections.ChainMap(dic1, dic2) # printing chainMap using maps print ("All the ChainMap contents are : ") print (chain.maps) # printing keys using keys() print ("All keys of ChainMap are : ") print (list(chain.keys())) # printing keys using keys() print ("All values of ChainMap are : ") print (list(chain.values()))
以下是上述代码的输出:
All the ChainMap contents are : [{'Program1': 'Python', 'Program2': 'HTML'}, {'Program3': ' Java', 'Program4': 'C'}] All keys of ChainMap are : ['Program3', 'Program4', 'Program1', 'Program2'] All values of ChainMap are : [' Java', 'C', 'Python', 'HTML']
ChainMap 中的方法
以下是 ChainMap() 类中定义的方法:
方法 | 功能 |
---|---|
new_child() | 在 ChainMap 的开头添加一个新的字典或迭代器 |
reversed() | 反转 ChainMap 中字典或迭代器的相对顺序 |
Python ChainMap.new_child() 方法
new_child() 方法用于在 ChainMap() 的开头添加一个新的字典或迭代器。
示例
在以下示例中,我们使用 new_child() 向 ChainMap() 添加了一个新的字典:
from collections import ChainMap list1 = ['a','b','c','d'] list2 = [1, 2, 3, 4] list3 = ['one','two','three'] chain_list = ChainMap(list1, list2) print(chain_list) print(chain_list.new_child(list3))
以下是上述代码的输出:
ChainMap(['a', 'b', 'c', 'd'], [1, 2, 3, 4]) ChainMap(['one', 'two', 'three'], ['a', 'b', 'c', 'd'], [1, 2, 3, 4])
Python ChainMap.reversed() 方法
reversed() 方法用于反转 ChainMap 中的字典或迭代器。
示例
以下是 ChainMap 中 reversed() 方法的示例:
from collections import ChainMap # Define multiple lists list1 = ['a', 'b', 'c', 'd'] list2 = [1, 2, 3] list3 = ['one', 'two'] # Create a ChainMap with the first two lists chain_list = ChainMap(list1, list2) # Print the initial ChainMap print("Original ChainMap -", chain_list) # Reverse the order of maps in the original ChainMap (note: reversed() does not modify the list) reversed_maps = list(reversed(chain_list.maps)) # This creates a reversed list # Print the reversed order of maps (for demonstration) print("Reversed maps -", reversed_maps)
以下是上述代码的输出:
Original ChainMap - ChainMap(['a', 'b', 'c', 'd'], [1, 2, 3]) Reversed maps - [[1, 2, 3], ['a', 'b', 'c', 'd']] Original maps after reversal attempt: [['a', 'b', 'c', 'd'], [1, 2, 3]]