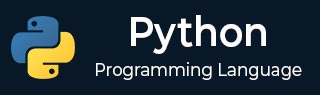
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python collections.Counter
Python Counter 是一个容器,用于保存对象的计数。它用于统计迭代对象中存在的项目数量。计数可以是任何整数值,包括零或负数。
Counter 是字典的子类。它以键值对的形式表示数据。它继承了字典的所有方法和属性。它允许执行算术和集合运算。它可以与任何实现迭代协议的迭代对象一起使用。
语法
以下是 Python Counter 的语法:
class collections.Counter([iterable-or-mapping])
参数
此数据类型接受迭代对象作为参数。
返回值
此数据类型返回计数器对象。
Counter 的初始化
Counter 使用迭代对象作为输入值进行初始化。以下是初始化 Counter 的几种不同方法:
- 使用一系列项目
- 使用包含键和计数的字典
- 使用将字符串名称映射到计数的关键字参数
示例
在下面的示例中,我们以不同的方式初始化了 Counter:
from collections import Counter # With sequence of items print(Counter(['x','x','z','x','y','z','x','x','z','x'])) # with dictionary print(Counter({'y':3, 'z':5, 'x':2})) # with keyword arguments print(Counter(z=3, x=5, y=2))
以下是上述代码的输出:
Counter({'x': 6, 'z': 3, 'y': 1}) Counter({'z': 5, 'y': 3, 'x': 2}) Counter({'x': 5, 'z': 3, 'y': 2})
示例
以下是 Python 中 Counter 的基本示例:
from collections import Counter # Create a list tuple1 = ('Python', 'Java', 'Python', 'C++', 'Python', 'Java') # Count distinct elements and print Counter a object print(Counter(tuple1))
以下是上述代码的输出:
Counter({'Python': 3, 'Java': 2, 'C++': 1})
Counter 值
我们还可以使用 keys()、values() 和 items() 方法访问计数器的所有键、值和项目。
示例
在这里,我们定义了 Counter 并找到了它的键值、值和项目:
from collections import Counter #defined Counter my_counter = Counter('xyzmnoxyzm') #Finding key values print(my_counter.keys()) #Finding values print(my_counter.values()) #Finding items print(my_counter.items())
以下是上述代码的输出:
dict_keys(['x', 'y', 'z', 'm', 'n', 'o']) dict_values([2, 2, 2, 2, 1, 1]) dict_items([('x', 2), ('y', 2), ('z', 2), ('m', 2), ('n', 1), ('o', 1)])
Counter 中的方法
以下是 Counter() 类中定义的不同方法:
方法 | 功能 |
---|---|
update() | 用于将元素或迭代对象添加到现有的 Counter 中 |
total() | 计算计数的总和 |
most_common() | 返回 n 个最常见元素及其计数的列表,从最常见到最不常见。如果省略 n 或为 None,most_common() 将返回计数器中的所有元素 |
elements() | 返回一个迭代器,该迭代器重复每个元素与其计数一样多的次数。元素按照首次遇到的顺序返回。 |
subtract() | 从迭代对象或另一个映射(或计数器)中减去元素。 |
Python Counter.update() 方法
Counter 类中的 update() 方法用于向计数器添加新元素。
示例
在这里,我们创建了一个空计数器,并使用 update() 函数添加了元素,Counter 返回具有相应计数的元素:
from collections import Counter #empty counter var1 = Counter() #updated with elements var1.update([2,4,6,2,4,6,2,6]) print(var1) var1.update([2, 6, 4]) print(var1)
以下是上述代码的输出:
Counter({2: 3, 6: 3, 4: 2}) Counter({2: 4, 6: 4, 4: 3})
Python Counter.subtract() 方法
Counter 类中的 subtract() 方法用于在两个计数器之间执行减法运算。两个计数器的减法结果可以是 零 或 负值:
示例
在这里,我们定义了两个计数器,c1 和 c2,并将 c2 从 c1 中减去:
from collections import Counter #defined counters c1 = Counter(A=4, B=3, C=10) c2 = Counter(A=10, B=3, C=4) #subtraction of c2 from cl c1.subtract(c2) print(c1)
以下是上述代码的输出:
Counter({'C': 6, 'B': 0, 'A': -6})
Python Counter.total() 方法
Counter 类中的 total() 方法用于计算计数器中所有元素计数的总和。
示例
在这里,我们定义了一个列表并将其转换为 Counter,然后找到所有元素计数的总和:
from collections import Counter list1 = ['a', 'b', 'c', 'a', 'b', 'c', 'a'] count = Counter(list1).total() print("Total elements of the list :", count)
以下是上述代码的输出:
Total elements of the list : 7
Python Counter.most_common() 方法
Python 的 Counter 类中的 `most_common()` 方法用于返回计数器中最常见的 n 个元素的列表。它按元素计数从高到低的顺序返回元素,从最常见到最不常见。如果未指定 n 值,则将返回计数器中的所有元素。
示例
以下是 Counter 的 `most_common()` 方法示例:
from collections import Counter tup1 = (2,56,13,4,2,10,13,2, ) count = Counter(tup1).most_common(2) print("Most common elements of the tuple :", count)
以下是上述代码的输出:
Most common elements of the tuple : [(2, 3), (13, 2)]