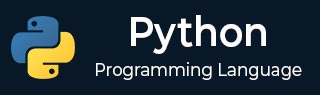
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - match-case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 文件重命名和删除
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python collections.defaultdict
Python 的 `defaultdict()` 是一个容器,类似于 `字典`。它位于 `collections` 模块中。它是字典类的子类,返回一个 `字典` 对象。
字典和 defaultdict 的功能相似,唯一的区别是 defaultdict 不会引发 `KeyError`。它为不存在的键提供一个默认值。
语法
以下是 Python `defaultdict()` 类的语法:
defaultdict(default_factory)
参数
以下是此类接受的参数:
- `default_factory`:这是一个函数,它为定义的字典返回一个默认值。如果未传递此参数,则函数会引发 KeyError。
返回值
此类返回一个 `
示例
以下是 Python `defaultdict()` 类的基本示例:
from collections import defaultdict def default(): return 'Key not found' dic1 = defaultdict(default) dic1[1] = 'one' dic1[2] = 'two' dic1[3] = 'Three' print(dic1) print(dic1[5])
以上代码的输出如下:
defaultdict(<function default at 0x000002040ACC8A40>, {1: 'one', 2: 'two', 3: 'Three'}) Key not found
使用带 `__missing__()` 方法的 defaultdict()
`__missing__()` 方法用于定义作为 defaultdict() 参数传递的 default_factory 的行为。如果 `default_factory` 为 `None`,则此方法会生成一个 `KeyError`。
示例
以下是带有 `__missing__()` 方法的 defaultdict() 示例:
from collections import defaultdict def default(): return 'Key not found' #defined defaultdict dic1 = defaultdict(default) dic1[1] = 'Python' dic1[2] = 'Java' dic1[3] = 'C++' print(dic1) #__missing__() function var1 = dic1.__missing__(1) print(var1)
以上代码的输出如下:
defaultdict(<function default at 0x000001F92A5F8A40>, {1: 'Python', 2: 'Java', 3: 'C++'}) Key not found
列表作为 default_factory
在 defaultdict() 中,我们可以将 `列表` 作为 default_factory 传递。当我们尝试查找字典中不存在的键的值时,它将返回一个空列表。
示例
在这里,我们定义了一个元组列表,每个元组包含两个值,键和值。我们将列表作为参数传递给 defaultdict() 函数并将项目追加到字典中:
# Python program to demonstrate # defaultdict from collections import defaultdict s = [('Python', 90), ('Java', 85), ('Python', 75), ('C++', 80), ('Java', 120)] dict1 = defaultdict(list) for k, v in s: dict1[k].append(v) sorted(dict1.items()) print(dict1) print("Key is not present in the dictionary :",dict1['html'])
以上代码的输出如下:
defaultdict(<class 'list'>, {'Python': [90, 75], 'Java': [85, 120], 'C++': [80]}) []
整数作为 default_factory
在 defaultdict() 中,当我们可以将 `int` 作为参数传递时,它使函数可计数。当我们尝试查找字典中不存在的键时,它将返回 `零`。
示例
在这里,我们将字符追加到字典中,当我们找到字典中不存在的键值时,结果为 `0`:
from collections import defaultdict var1 = 'Hello' dict1 = defaultdict(int) for k in var1: dict1[k] += 1 sorted(dict1.items()) print(dict1) #Value of key which is not found in dictionary print("Key Value :", dict1['k'])
以上代码的输出如下:
defaultdict(<class 'int'>, {'H': 1, 'e': 1, 'l': 2, 'o': 1}) Key Value : 0
python_modules.htm
广告