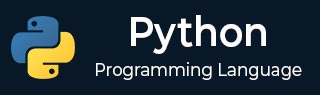
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 连接线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python else 关键字
在 Python 中,else 关键字用于条件语句。只有当 if 条件为 False 时,才会执行 else 代码块。此关键字在语法上依赖于 if 关键字。如果我们在没有 if 语句的情况下使用 else 关键字,我们将得到一个 SyntaxError。
语法
以下是 Python else 关键字的语法:
if condition: statement1 statement2 else: statement3 statement4
示例
以下是 Python else 关键字的基本示例:
if False: print("Hello") else: print("Hello world")
输出
以下是上述代码的输出:
Hello world
在函数中使用 else 关键字
else 关键字也可以用于函数中以检查条件语句。
示例
这里,我们定义了一个名为 fun1 的函数来检查数字是否为 正数:
def fun1(num): if num<0: return False else: return True x=9 print(x,"is a positive number :",fun1(x)) y=-4 print(y,"is a positive number :",fun1(y))
输出
以下是上述代码的输出:
9 is a positive number : True -4 is a positive number : False
在循环中使用 else 关键字
else 关键字用于基于条件语句的 循环。
示例
让我们尝试理解循环中的 else 关键字:
x=[1,2,3,4] for i in x: if i%2==0: print(i,"is a even number in the list") else: print(i,"is not a even number in the list")
输出
以下是上述代码的输出:
1 is not a even number in the list 2 is a even number in the list 3 is not a even number in the list 4 is a even number in the list
在没有 if 语句的情况下使用 else 关键字
else 关键字依赖于 if 条件。如果我们在没有 if 条件的情况下使用 else 代码块,我们将得到一个 SyntaxError。
示例
else: print("Hello")
输出
以下是上述代码的输出:
File "E:\pgms\Keywords\else.py", line 28 else: ^^^^ SyntaxError: invalid syntax
将 else 关键字与 elif 一起使用
当有多个条件语句要检查时,我们可以使用 elif。如果所有给定的条件都为 False,则将执行 else 代码块。
示例
这里,是 else 与 elif 关键字一起使用的示例:
if False: print("Welcome") elif False: print("To") elif False: print("the") else: print("Welcome to Tutorials Point")
输出
以下是上述代码的输出:
Welcome to Tutorials Point
将 else 与 try & except 块一起使用
我们还可以将 else 关键字与 try 和 except 块一起使用。在这种情况下,只有当 try 代码块不引发任何错误时,才会执行 else 代码块。
示例
让我们尝试执行 else 与 try 和 except 块一起使用:
x = 5 try: x < 10 print("This statement is executed") except: print("Something went wrong") else: print("This statement is executed only if try block is executed without raising any errors")
输出
以下是上述代码的输出:
This statement is executed This statement is executed only if try block is executed without raising any errors
嵌套 else
当在一个 else 代码块内有多个 else 时,称为 嵌套 else。
示例
这里,是嵌套 else 的示例:
if False: print("This is not executed") else: if False: print("Hello World") else: print("This statement is executed")
输出
以下是上述代码的输出:
This statement is executed
python_keywords.htm
广告