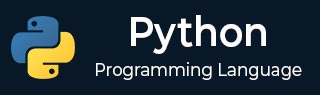
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - match-case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOP 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 终止线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - pyperclip 模块
在 Python 中,pyperclip 模块用于各种与剪贴板相关的操作,例如剪切、复制和粘贴。它非常适合需要在应用程序、脚本或涉及文本操作的多个进程之间传输数据的情况。
在本教程中,我们将学习 **pyperclip** 模块的安装、其用途以及如何通过示例使用它。
什么是 pyperclip 模块?为什么需要它?
**pyperclip** 模块对于自动化涉及剪贴板的文本处理非常重要。您可以将数据从脚本复制到剪贴板,然后轻松地粘贴到任何文档、浏览器或任何应用程序中。同样,您无需手动复制您已放入剪贴板的内容并将其粘贴到脚本中。
pyperclip 模块的用例包括:
- 自动将脚本结果复制到其他应用程序。
- 自动化需要重复手动复制粘贴的任务。
- 创建用于操作文本的工具或实用程序。
使用 PIP 安装 pyperclip 模块
您可以通过 pip 安装 pyperclip 模块,以下是安装命令:
pip install pyperclip
将文本复制到剪贴板
要将特定文本复制到剪贴板,可以使用 pyperclip.copy() 方法。此函数接受字符串作为输入,并将其直接传输到系统的剪贴板。复制后,文本可用于粘贴到任何支持剪贴板操作的应用程序中。
示例
此示例演示如何将文本复制到剪贴板:
import pyperclip as pc def transfer_to_buffer(info): """Transfers the given info to the system's clipboard.""" pc.copy(info) print("Data has been transferred to the clipboard!") # The message to be copied to the clipboard buffer_data = "Clipboard operation successful!" # Executing the transfer transfer_to_buffer(buffer_data)
用于复制和粘贴文本的模块化函数
您可以将剪贴板功能包装在模块化函数中,以使操作更有条理和可重用。我们可以使用函数快速将文本复制到剪贴板或使用 **pyperclip.paste()** 从剪贴板中检索它。
示例
此示例演示了上述概念:
import pyperclip as pc def fetch_from_buffer(): """Fetches and returns data from the system's clipboard.""" return pc.paste() # Retrieve data from clipboard retrieved_data = fetch_from_buffer() print("Data fetched from clipboard:", retrieved_data)
使用模块化函数复制和粘贴文本
这两种方法都重载了行为,其中包括一个工作流程,可以快速将某些内容复制到剪贴板,然后在其他代码或文档中获取该内容。
示例
import pyperclip as pc def transfer_to_buffer(data): # Transfers the given data to the clipboard. pc.copy(data) print("Data has been transferred to the clipboard!") def retrieve_from_buffer(): # Fetches and returns the current content of the clipboard. return pc.paste() # Data to transfer to the clipboard data_for_transfer = "This data was transferred using the buffer." # Transfer the data to the clipboard transfer_to_buffer(data_for_transfer) # Retrieve the data from the clipboard buffer_content = retrieve_from_buffer() # Display the retrieved data from the clipboard print(f"Data fetched from clipboard: {buffer_content}")
创建 .txt 文件并将文本从剪贴板粘贴到文件中
在这里,我们将创建一个程序,该程序能够将剪贴板的内容保存到 .txt 文件中。我们将使用写入模式 ('w') 的 **open()** 函数来创建或覆盖文本文件,并将检索到的剪贴板内容写入该文件。此功能用于保存剪贴板内容以供将来参考或记录。
示例
import pyperclip as pc def transfer_to_buffer(data): """Transfers the given data to the clipboard.""" pc.copy(data) print("Data has been transferred to the clipboard!") def retrieve_from_buffer(): """Fetches and returns the current content of the clipboard.""" return pc.paste() def save_data_to_file(file_name, data): """Saves the provided data to a file.""" with open(file_name, 'w') as file: file.write(data) print(f"Data saved to {file_name}.") # Text to copy to the clipboard data_for_transfer = "This data was transferred using the buffer." # Copy the text to the clipboard transfer_to_buffer(data_for_transfer) # Retrieve the text from the clipboard buffer_content = retrieve_from_buffer() # Display the text retrieved from the clipboard print(f"Data fetched from clipboard: {buffer_content}") # Save the retrieved text to a .txt file file_name = 'output.txt' save_data_to_file(file_name, buffer_content)
输出
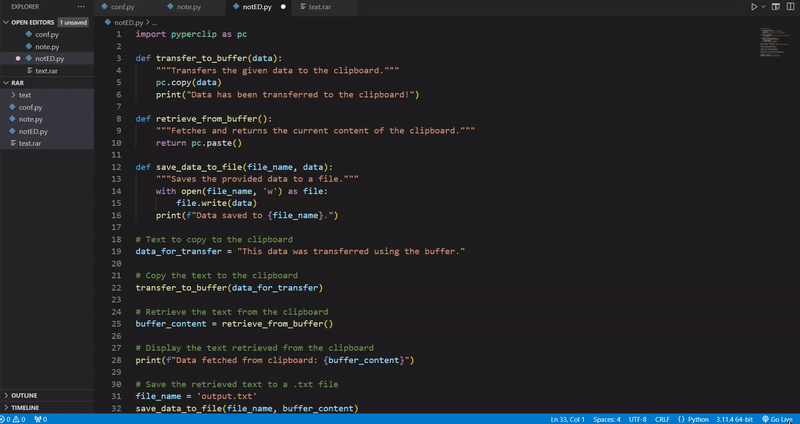
**查看我们的示例 4 代码**,这段代码将在我们的电脑路径中自动创建一个 output.txt 文件,您编写的代码将被复制并粘贴到该txt文件中。
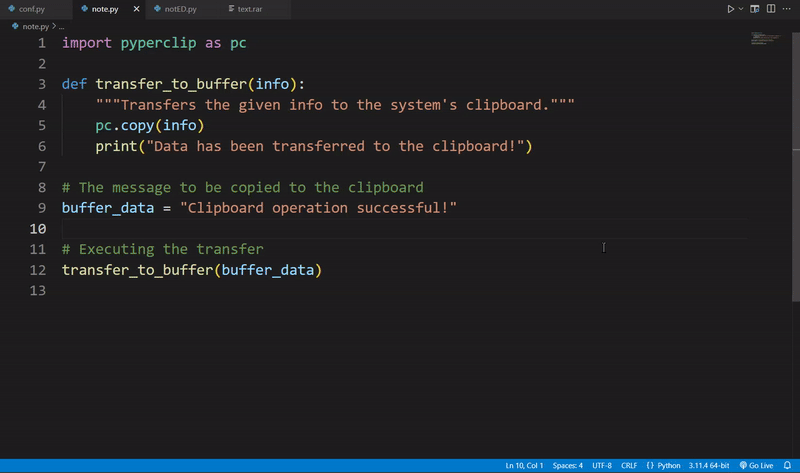
python_reference.htm
广告