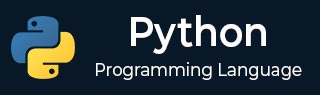
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策制定
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python random.lognormvariate() 方法
Python 中的 **random.lognormvariate()** 方法生成遵循对数正态 (lognormal) 分布的随机数。这种分布是连续概率分布族,随机变量的对数服从正态分布。
它依赖于两个参数,**mu** 和 **sigma**,其中 mu 是均值,sigma 是基础正态分布的标准差。
对数正态分布常用于自然科学、工程、医学、经济学和其他领域。
此函数无法直接访问,因此我们需要导入 random 模块,然后需要使用 random 静态对象调用此函数。
语法
以下是 lognormvariate() 方法的语法:
random.lognormvariate(mu, sigma)
参数
Python random.lognormvariate() 方法接受两个参数:
**mu:** 这是基础正态分布的均值(对数正态分布的自然对数)。它可以取任何实数值。
**sigma:** 这是基础正态分布的标准差。它必须大于零。
返回值
此 **random.lognormvariate()** 方法返回一个遵循对数正态分布的随机数。
示例 1
让我们看看使用 **random.lognormvariate()** 方法从均值为 0,标准差为 1 的正态分布生成随机数的基本示例。
import random # mean mu = 0 # standard deviation sigma = 1 # Generate a log normal-distributed random number random_number = random.lognormvariate(mu, sigma) # Print the output print("Generated random number from log normal distribution:",random_number)
以下是输出:
Generated random number from log normal distribution: 9.472544796309364
**注意:**由于其随机性,每次运行程序时生成的输出都会有所不同。
示例 2
此示例使用 **random.lognormvariate()** 方法生成一个包含 10 个遵循对数正态分布的随机数的列表。
import random # mean mu = 0 # standard deviation sigma = 0.5 result = [] # Generate a list of random numbers from the log normal distribution for i in range(10): result.append(random.lognormvariate(mu, sigma)) print("List of random numbers from log normal distribution:", result)
执行上述代码时,您将获得类似于以下的输出:
List of random numbers from log normal distribution: [0.500329149795808, 1.7367179979113172, 0.5143664713594474, 0.5493391936855808, 1.3565058546966193, 1.4841135680348012, 0.5950837276748621, 0.8880005878135713, 1.0527856543498058, 0.7471389015523113]
示例 3
这是一个使用 **random.lognormvariate()** 方法的另一个示例,它演示了如何更改均值和标准差会影响正态分布的形状。
import random import matplotlib.pyplot as plt # Define a function to generate and plot data for a given mu and sigma def plot_log_norm(mu, sigma, label, color): # Generate log normal-distributed data data = [random.lognormvariate(mu, sigma) for _ in range(10000)] # Plot histogram of the generated data plt.hist(data, bins=100, density=True, alpha=0.8, color=color, label=f'(mu={mu}, sigma={sigma})') fig = plt.figure(figsize=(7, 4)) # Plotting for each set of parameters plot_log_norm(0, 1, '0, 1', 'blue') plot_log_norm(0, 0.5, '0, 0.5', 'green') plot_log_norm(0, 0.25, '0, 0.25', 'yellow') # Adding labels and title plt.title('Log Normal Distributions') plt.legend() # Show plot plt.show()
以上代码的输出如下:
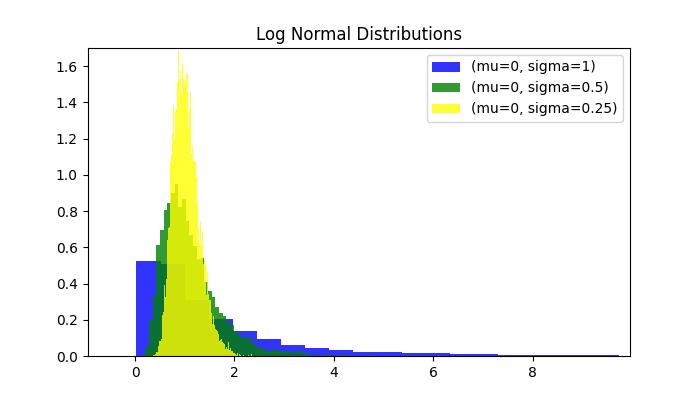
广告