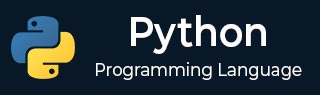
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 连接线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期和时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - Rarfile 模块
在 Python 中,**rarfile** 模块用于处理 rar 压缩文件(.rar 文件)。通过使用 **rarfile** 模块,您可以获取文件、列出源内容,甚至直接读取压缩文件中的文件,而无需提取它们。此库是应用程序 **unrar** 或 **rar** 的直接 Python 访问,后者必须在命令级别安装。
在本教程中,我们将学习 **rarfile** 模块的安装方法、使用方法、模块提供的多种方法以及简单的示例。
安装 rarfile 模块
要使用 **rarfile** 模块,您必须安装它以及 **unrar** 或 **rar** 工具,这些工具将在模块内部使用以处理 RAR 压缩文件。
要安装 rarfile 模块,请使用以下命令:
pip install rarfile
安装 WinRAR 实用程序
提取和处理 RAR 文件需要 **WinRAR** 命令行工具。您可以从官方 WINRAR 网站下载它。下载适用于 Windows 的 **WinRAR** 并安装。
将 **C:\Program Files\WinRAR\** 添加到系统的 **Path** 环境变量中,以启用命令行访问。之后,WinRAR 将可以与 Python 的 **rarfile** 模块一起使用。
rarfile 模块的方法
以下是 rarfile 模块的重要方法:
序号 | 方法及描述 |
---|---|
1 | rarfile.open(name, mode='r', password=None) 此方法用于读取 RAR 压缩文件中的文件,但将其写入另一个文件会将所有数据加载到 RAM 中。这意味着此方法将返回一个类似文件的对象。 |
2 | rarfile.extract(member, path=None, password=None) 此方法将 RAR 压缩文件中的一个源文件提取到指定的目录。 |
3 | rarfile.extractall(path=None, password=None) 此方法提取所有文件并将所有文件保存到参数中指定的目录。 |
4 | rarfile.extractall(members=None, path=None, password=None) 此方法用于将 RAR 压缩文件中指定的多个文件提取到指定的目录。如果“members”为“None”,则解压所有文件。 |
5 | rarfile.infolist() 此方法返回压缩文件中所有文件的“RarInfo”对象的列表,无论它们是否都描述文件,因此,每个“RarInfo”对象都将包含对应于该文件的详细信息。 |
6 | rarfile.getinfo(name) 此方法用于查找有关压缩文件的信息,并返回一个“RarInfo”对象。 |
7 | rarfile.namelist() 此方法实际上是一个解压实用程序,它返回压缩文件中所有文件的名称列表。 |
8 | rarfile.test(zipfile=None) 此方法检查档案或档案中选定文件的“健壮性”,如果未发现错误,则返回“True”。 |
9 | rarfile.extractfile(name) 此方法为档案中的给定文件返回“类文件”对象,允许读取文件内容,而无需实际从档案中提取文件。 |
10 | rarfile.close() 此方法用于保存和释放RAR文件及其连接的任何资源。当使用“with”语句时,此方法通常会自动调用,但也可以在其他时间手动调用。 |
rarfile模块示例
安装rarfile模块和winrar后,您就可以开始在Python中使用RAR文件。以下是一些示例:
示例1:显示RAR档案的内容
在此示例中,我们将看到如何在不提取的情况下列出RAR档案中包含的所有文件。它打印档案中每个文件的名称和大小:
from rarfile import RarFile # Path to the RAR file file_archive = 'data_archive.rar' # Open the RAR file archive_instance = RarFile(file_archive) try: # Process each file in the RAR archive for file_entry in archive_instance.infolist(): print(f"Name: {file_entry.filename}, Size: {file_entry.file_size} bytes") finally: # Ensure the file is closed properly archive_instance.close()
示例2:将RAR档案中的所有文件提取到目录
此示例演示如何将RAR档案中的所有文件提取到指定的目录。它确保在提取后正确关闭RAR文件:
import rarfile # Specify the path to unrar.exe rarfile.UNRAR_TOOL = r"C:\Program Files\WinRAR\Unrar.exe" # Path to the RAR file rar_file_location = “text.rar" # Directory where files will be extracted output_directory = './extracted_files' # Access the RAR file rar_archive = rarfile.RarFile(rar_file_location) try: # Extract all contents to the specified directory rar_archive.extractall(path=output_directory) finally: # Close the RAR file to release system resources rar_archive.close()
示例3:从RAR档案中提取单个文件
在此示例中,我们将特定文件从RAR档案提取到给定目录。代码在提取之前检查文件是否存在:
import rarfile # Location of the RAR file rar_file_path = 'data_archive.rar' # Name of the file to extract target_file = 'report.txt' # Open the RAR file rar_archive = rarfile.RarFile(rar_file_path) try: # Extract the specific file to the target directory rar_archive.extract(target_file, path='./extracted_files') finally: # Close the RAR file to release resources rar_archive.close()
示例4:直接从RAR档案读取文件(无需提取)
此示例说明如何在不提取的情况下读取RAR档案内文件的內容。文件直接从档案中打开和读取,并打印其内容:
import rarfile # Location of the RAR file compressed_file_path = 'data_archive.rar' # File to be read from within the RAR archive inner_file = 'notes.txt' # Open the RAR file rar_archive = rarfile.RarFile(compressed_file_path) try: # Access and read the contents of the specified file inside the archive extracted_file = rar_archive.open(inner_file) try: file_content = extracted_file.read() print(file_content.decode('utf-8')) # Assuming the file contains text finally: extracted_file.close() finally: # Close the RAR archive rar_archive.close()
示例5:验证RAR档案中是否存在文件
此示例演示如何检查特定文件是否存在于RAR档案中。如果找到该文件,则会显示一条消息指示其存在:
import rarfile # Path to the RAR archive rar_file_location = 'data_archive.rar' # Name of the file to check within the archive target_file_name = 'report.txt' # Open the RAR archive rar_archive = rarfile.RarFile(rar_file_location) try: # Check if the file exists in the archive if target_file_name in rar_archive.namelist(): print(f"'{target_file_name}' is available in the archive.") else: print(f"'{target_file_name}' was not found in the archive.") finally: # Close the RAR archive rar_archive.close()
输出
此示例演示如何将RAR档案中的所有文件提取到指定的文件夹中。(请参阅我们的示例2代码)
我们将Text.rar档案文件转换为Folder文件夹:
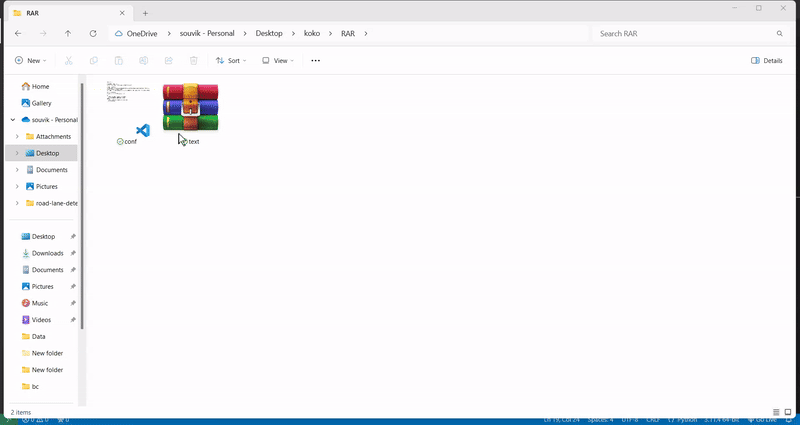