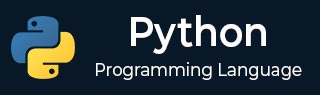
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 合并线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python Requests post() 方法
Python Requests 的 post() 方法用于向指定的 URL 发送 HTTP POST 请求。它允许向服务器发送数据,通常用于提交表单或上传文件。
此方法接受诸如“url”、“data”、“json”、“headers”、“cookies”、“files”和“timeout”之类的参数。使用“data”或“json”分别发送表单数据或 JSON 有效负载,其中“headers”可用于发送自定义 HTTP 标头,“files”可用于上传文件。
它返回一个响应对象,其中包含状态代码、标头和内容等详细信息,从而促进与 Web 服务的交互。
语法
以下是 Python Requests post() 方法的语法和参数:
requests.post(url, data=None, json=None, headers=None, params=None, auth=None, timeout=None, verify=None)
参数
以下是 Python Requests post() 方法的参数:
- url: 发送请求的 URL。
- data: 请求的主体。这可以是字典、字节或类文件对象,用于发送到请求的主体中。
- json: 发送到请求主体中的 JSON 数据。
- headers: 与请求一起发送的 HTTP 标头。
- files: 与请求一起上传的文件。
- auth: Auth 元组以启用 Basic/Digest/Custom HTTP Auth 或自定义身份验证可调用对象。
- cookies: 与请求一起发送的 Cookie。
- timeout: 请求的超时时间。
- allow_redirects: 确定是否应跟踪重定向。
- proxies: 一个字典,将协议映射到代理的 URL。
- verify: 控制是否启用 SSL 证书验证。
返回值
此方法返回一个 Response 对象。
示例 1
以下是一个基本示例,它使用 python requests 的 post() 方法向指定的 URL 发送一个简单的 POST 请求,并使用表单数据:
import requests # Define the URL url = 'https://httpbin.org/post' # Define the data to be sent in the request body data = {'key1': 'value1', 'key2': 'value2'} # Send the POST request with the data response = requests.post(url, data=data) # Print the response status code print('Status Code:', response.status_code) # Print the response content print('Response Content:', response.text)
输出
Status Code: 200 Response Content: { "args": {}, ------------- ------------ ------------ "json": null, "origin": "110.226.149.205", "url": "https://httpbin.org/post" }
示例 2
服务器将接收带有提供的 JSON 数据的 POST 请求,并打印来自服务器的响应,包括状态代码和任何响应内容。这是一个示例:
import requests # Define the URL url = 'https://httpbin.org/post' # Define the JSON data to be sent in the request body json_data = {'key1': 'value1', 'key2': 'value2'} # Send the POST request with the JSON data response = requests.post(url, json=json_data) # Print the response status code print('Status Code:', response.status_code) # Print the response content print('Response Content:', response.text)
输出
Status Code: 200 Response Content: { "args": {}, "data": "{\"key1\": \"value1\", \"key2\": \"value2\"}", ------------- ------------ ------------ "json": { "key1": "value1", "key2": "value2" }, "origin": "110.226.149.205", "url": "https://httpbin.org/post" }
示例 3
以下是一个使用 Python 的 requests 模块 post() 方法发送带有超时设置的 POST 请求的示例:
import requests # Define the URL url = 'https://httpbin.org/post' # Define the JSON data to be sent in the request body json_data = {'key1': 'value1', 'key2': 'value2'} # Set the timeout for the request (in seconds) timeout = 5 try: # Send the POST request with the JSON data and timeout response = requests.post(url, json=json_data, timeout=timeout) # Print the response status code print('Status Code:', response.status_code) # Print the response content print('Response Content:', response.text) except requests.Timeout: # Handle timeout error print('Timeout Error: Request timed out.') except requests.RequestException as e: # Handle other request exceptions print('Request Exception:', e)
输出
Status Code: 200 Response Content: { "args": {}, "data": "{\"key1\": \"value1\", \"key2\": \"value2\"}", ------------- ------------ ------------ }, "origin": "110.226.149.205", "url": "https://httpbin.org/post" }
python_modules.htm
广告