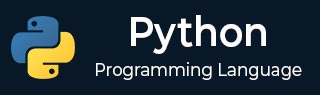
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - match-case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 使用 Flask 构建问答应用项目
在这里,我们将使用 Python Flask 创建一个问答应用程序。 Flask 是一个轻量级的 Python Web 应用框架。选项包括选择测验、回答问题以及在应用程序上查看结果。它具有用于处理测验会话的动态路由,并可以使用 HTML 模板和 CSS 来实现用户界面,使其更加精致。
安装
使用 pip 安装 Flask −
pip install flask
问答应用项目代码文件
以下是创建问答应用项目的代码文件 −
1. 文件:app.py
from flask import Flask, render_template, request, redirect, url_for, session app = Flask(__name__) app.secret_key = 'your_secret_key' # Example quiz data quiz_data = { "quizzes": { "General Knowledge": [ { "question": "What is the capital of France?", "options": ["Paris", "London", "Berlin", "Madrid"], "answer": "Paris" }, { "question": "Which planet is known as the Red Planet?", "options": ["Earth", "Mars", "Jupiter", "Venus"], "answer": "Mars" }, { "question": "Who wrote 'To Kill a Mockingbird'?", "options": ["Harper Lee", "Mark Twain", "J.K. Rowling", "Jane Austen"], "answer": "Harper Lee" }, { "question": "What is the largest ocean on Earth?", "options": ["Atlantic Ocean", "Indian Ocean", "Arctic Ocean", "Pacific Ocean"], "answer": "Pacific Ocean" }, { "question": "What is the smallest country in the world?", "options": ["Monaco", "San Marino", "Vatican City", "Liechtenstein"], "answer": "Vatican City" }, { "question": "In which year did the Titanic sink?", "options": ["1912", "1905", "1898", "1923"], "answer": "1912" }, { "question": "What is the hardest natural substance on Earth?", "options": ["Gold", "Iron", "Diamond", "Platinum"], "answer": "Diamond" }, { "question": "Which element has the chemical symbol 'O'?", "options": ["Oxygen", "Gold", "Silver", "Osmium"], "answer": "Oxygen" }, { "question": "Who painted the Mona Lisa?", "options": ["Leonardo da Vinci", "Vincent van Gogh", "Claude Monet", "Pablo Picasso"], "answer": "Leonardo da Vinci" }, { "question": "What is the longest river in the world?", "options": ["Nile", "Amazon", "Yangtze", "Mississippi"], "answer": "Nile" } ], "Science": [ { "question": "What is the chemical symbol for water?", "options": ["H2O", "O2", "CO2", "HO2"], "answer": "H2O" }, { "question": "Who developed the theory of relativity?", "options": ["Isaac Newton", "Albert Einstein", "Galileo Galilei", "Nikola Tesla"], "answer": "Albert Einstein" }, { "question": "What is the powerhouse of the cell?", "options": ["Nucleus", "Mitochondria", "Ribosome", "Endoplasmic Reticulum"], "answer": "Mitochondria" }, { "question": "What planet is known for its rings?", "options": ["Saturn", "Jupiter", "Uranus", "Neptune"], "answer": "Saturn" }, { "question": "What is the main gas found in the air we breathe?", "options": ["Oxygen", "Nitrogen", "Carbon Dioxide", "Hydrogen"], "answer": "Nitrogen" }, { "question": "What is the chemical symbol for gold?", "options": ["Au", "Ag", "Pb", "Fe"], "answer": "Au" }, { "question": "What is the speed of light?", "options": ["300,000 km/s", "150,000 km/s", "100,000 km/s", "200,000 km/s"], "answer": "300,000 km/s" }, { "question": "Who is known as the father of modern physics?", "options": ["Isaac Newton", "Albert Einstein", "Niels Bohr", "Richard Feynman"], "answer": "Albert Einstein" }, { "question": "What is the chemical formula for methane?", "options": ["CH4", "C2H6", "C3H8", "C4H10"], "answer": "CH4" }, { "question": "What force keeps us grounded on Earth?", "options": ["Gravity", "Magnetism", "Friction", "Electromagnetism"], "answer": "Gravity" } ] } } @app.route('/') def index(): return render_template('index.html', quizzes=quiz_data["quizzes"]) @app.route('/start_quiz/<quiz_name>') def start_quiz(quiz_name): session['quiz_name'] = quiz_name session['current_question'] = 0 session['score'] = 0 return redirect(url_for('quiz_question')) @app.route('/quiz_question', methods=['GET', 'POST']) def quiz_question(): quiz_name = session.get('quiz_name') current_question = session.get('current_question', 0) # Default to 0 if not set quiz_questions = quiz_data["quizzes"].get(quiz_name, []) if request.method == 'POST': selected_option = request.form.get('option') correct_answer = quiz_questions[current_question]['answer'] # Check if the selected answer is correct if selected_option == correct_answer: session['score'] = session.get('score', 0) + 1 # Increment score if the answer is correct feedback = "Correct! Well done." else: feedback = f"Wrong! The correct answer is {correct_answer}." # Move to the next question session['current_question'] = current_question + 1 session['feedback'] = feedback if session['current_question'] >= len(quiz_questions): return redirect(url_for('quiz_result')) return redirect(url_for('quiz_question')) # Handle GET request: get current question data if current_question < len(quiz_questions): current_question_data = quiz_questions[current_question] feedback = session.pop('feedback', '') # Remove feedback from session return render_template('quiz_question.html', question_data=current_question_data, current_question=current_question + 1, total_questions=len(quiz_questions), feedback=feedback) else: return redirect(url_for('quiz_result')) @app.route('/quiz_result') def quiz_result(): score = session.get('score', 0) # Default to 0 if score is not found quiz_name = session.get('quiz_name') total_questions = len(quiz_data["quizzes"].get(quiz_name, [])) # Handle case where quiz_name might not be found return render_template('quiz_result.html', score=score, total_questions=total_questions) if __name__ == '__main__': app.run(debug=True)
2. 文件:index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Quiz Platform</title> <link rel="stylesheet" href="{{ url_for('static', filename='styles.css') }}"> </head> <body> <header> <h1>Welcome to the Quiz Platform</h1> </header> <div class="container"> <h1>Select a Quiz</h1> <ul> {% for quiz in quizzes %} <li><a href="{{ url_for('start_quiz', quiz_name=quiz) }}">{{ quiz }}</a></li> {% endfor %} </ul> </div> </body> </html>
3. 文件 (quiz_question.html)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Quiz Question</title> <link rel="stylesheet" href="{{ url_for('static', filename='styles.css') }}"> </head> <body> <header> <h1>Quiz Question</h1> </header> <div class="container"> <h2>Question {{ current_question }} of {{ total_questions }}</h2> <form method="post"> <p>{{ question_data['question'] }}</p> {% for option in question_data['options'] %} <div> <input type="radio" id="{{ option }}" name="option" value="{{ option }}"> <label for="{{ option }}">{{ option }}</label> </div> {% endfor %} <button type="submit">Submit</button> </form> <p>{{ feedback }}</p> </div> </body> </html>
4. 文件:(quiz_result.html)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Quiz Result</title> <link rel="stylesheet" href="{{ url_for('static', filename='styles.css') }}"> </head> <body> <header> <h1>Quiz Result</h1> </header> <div class="container"> <h2>Your Score</h2> <p>You scored {{ score }} out of {{ total_questions }}.</p> <a href="{{ url_for('index') }}">Return to Home</a> </div> </body> </html>
5. 文件 (styles.css)
body { font-family: Arial, sans-serif; background-color: #f4f4f4; margin: 0; padding: 0; } header { background-color: #333; color: #fff; padding: 10px 0; text-align: center; } .container { max-width: 800px; margin: 20px auto; padding: 20px; background: #fff; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } h1, h2 { color: #333; } ul { list-style-type: none; padding: 0; } li { margin: 10px 0; } a { text-decoration: none; color: #007bff; } a:hover { text-decoration: underline; } form div { margin: 10px 0; } input[type="radio"] { margin-right: 10px; } button { background-color: #007bff; color: #fff; border: none; padding: 10px 20px; cursor: pointer; } button:hover { background-color: #0056b3; }
背景图片文件
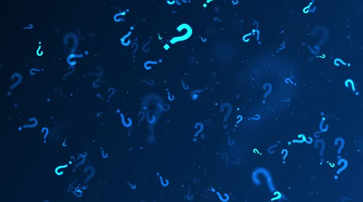
文件结构
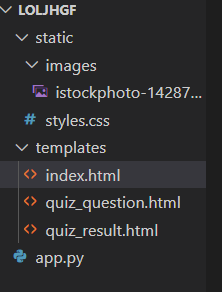
使用应用程序的步骤
- **设置虚拟环境** − 创建并激活一个虚拟空间。
- **安装依赖项** − 您可以使用安装 Python 时使用的 pip 来安装 Flask,使用命令 pip install Flask,如下面的图片所示。
- **运行应用程序** − 通过运行代码启动 Flask,并在 Web 浏览器中打开应用程序。
- **与应用程序交互** − 完成问卷,回答问题并查看您的分数。
输出
可以通过 http://127.0.0.1:5000/ 访问该应用程序。转到您的隐身标签。
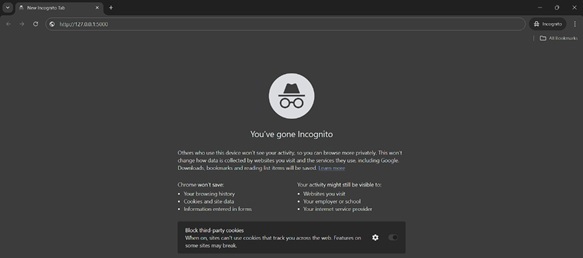
搜索后,您将看到此界面 −
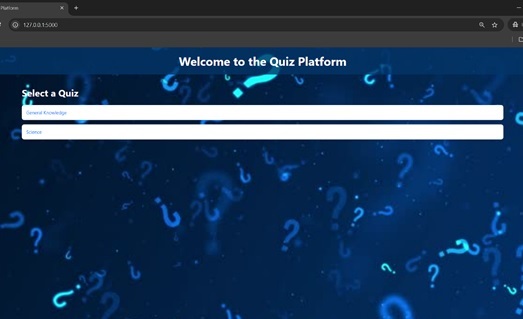
您可以选择任何一个,我选择常识 −
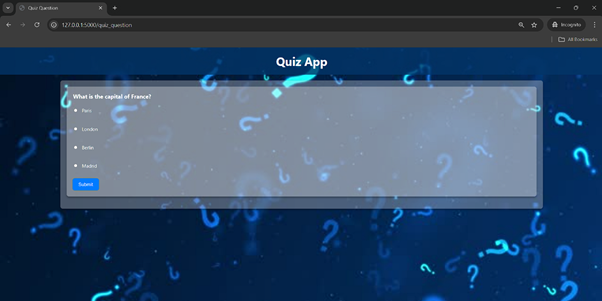
成功完成测验后,您将看到 −
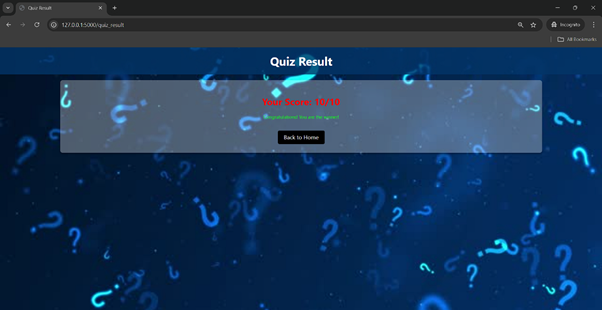
python_projects_from_basic_to_advanced.htm
广告