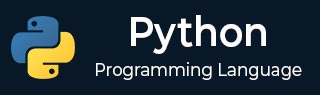
- Python基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python控制语句
- Python - 控制流
- Python - 决策
- Python - if语句
- Python - if else
- Python - 嵌套if
- Python - Match-Case语句
- Python - 循环
- Python - for循环
- Python - for-else循环
- Python - while循环
- Python - break语句
- Python - continue语句
- Python - pass语句
- Python - 嵌套循环
- Python函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python列表
- Python - 列表
- Python - 访问列表项
- Python - 修改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python元组
- Python - 元组
- Python - 访问元组项
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python集合
- Python - 集合
- Python - 访问集合项
- Python - 添加集合项
- Python - 删除集合项
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python字典
- Python - 字典
- Python - 访问字典项
- Python - 修改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS文件/目录方法
- Python - OS路径方法
- 面向对象编程
- Python - OOPs概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except块
- Python - try-finally块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套try块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 合并线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL处理
- Python - 泛型
- Python库
- NumPy教程
- Pandas教程
- SciPy教程
- Matplotlib教程
- Django教程
- OpenCV教程
- Python杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI编程
- Python - XML处理
- Python - GUI编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类的元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python实用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 实用资源
- Python - 讨论
- Python编译器
- NumPy编译器
- Matplotlib编译器
- SciPy编译器
Python中的随机游走实现
随机游走是一个每一步都随机确定的过程,通常用于模拟不可预测的运动。它用于描述由一系列随机移动组成的路径。
简单的随机游走可以是一维的,其中粒子必须向左或向右移动,没有任何偏向。关于随机游走应用于更高维度(如二维、三维和四维)的概念,运动是在特定维度中随机方向进行的。
每个额外的维度都会增加游走的难度,并提供更多关于随机过程和空间搜索的信息。这些理论包含了Python代码,用于一维、二维、三维和四维随机游走,以解释如何用计算机图形模拟它们。
所需库的安装
1. NumPy
用于Python数值计算的NumPy库,用于处理数组和执行数学运算。
语法
pip install numpy
2. Matplotlib
Matplotlib是一个绘图库,用于在Python中创建静态、动画和交互式可视化。
语法
pip install matplotlib
一维随机游走的实现
以下代码用于在Python中实现一维随机游走:
import numpy as np import matplotlib.pyplot as plt def random_walk_1d(steps): """Generate a 1D random walk.""" walk = np.zeros(steps) for i in range(1, steps): step = np.random.choice([-1, 1]) walk[i] = walk[i - 1] + step return walk # Number of steps steps = 1000 walk = random_walk_1d(steps) # Plot the random walk plt.figure(figsize=(10, 6)) plt.plot(walk, label='1D Random Walk') plt.xlabel('Steps') plt.ylabel('Position') plt.title('1D Random Walk') plt.legend() plt.show()
输出
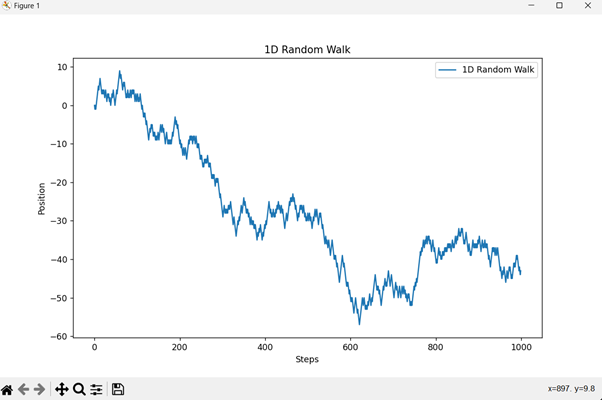
代码解释
- **导入** - numpy用于数值运算。matplotlib.pyplot用于绘制游走。**random_walk_1d**
- **函数** - 创建一维随机游走。
对于每一步,随机决定向左移动(-1)还是向右移动(+1)。
累加这些步数以计算每一步的位置。 - **绘图** - 绘制位置与步数的关系图,以便可视化随机游走。
二维随机游走的实现
以下代码用于在Python中实现二维随机游走:
import numpy as np import matplotlib.pyplot as plt def random_walk_2d(steps): """Generate a 2D random walk.""" positions = np.zeros((steps, 2)) for i in range(1, steps): step = np.random.choice(['up', 'down', 'left', 'right']) if step == 'up': positions[i] = positions[i - 1] + [0, 1] elif step == 'down': positions[i] = positions[i - 1] + [0, -1] elif step == 'left': positions[i] = positions[i - 1] + [-1, 0] elif step == 'right': positions[i] = positions[i - 1] + [1, 0] return positions # Number of steps steps = 1000 positions = random_walk_2d(steps) # Plot the random walk plt.figure(figsize=(10, 10)) plt.plot(positions[:, 0], positions[:, 1], label='2D Random Walk') plt.xlabel('X Position') plt.ylabel('Y Position') plt.title('2D Random Walk') plt.legend() plt.grid(True) plt.show()
输出
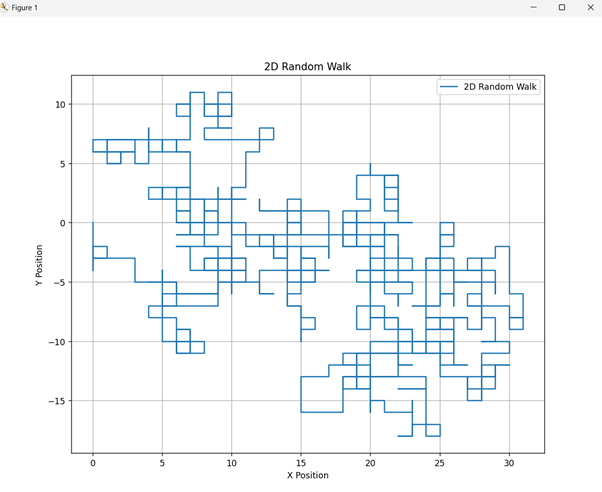
代码解释
**向四个方向之一移动** - 向上、向下、向左或向右。相应地更新位置并记录每一步。
三维随机游走的实现
以下代码用于在Python中实现三维随机游走:
import numpy as np import matplotlib.pyplot as plt from mpl_toolkits.mplot3d import Axes3D def random_walk_3d(steps): """Generate a 3D random walk.""" positions = np.zeros((steps, 3)) for i in range(1, steps): step = np.random.choice(['x+', 'x-', 'y+', 'y-', 'z+', 'z-']) if step == 'x+': positions[i] = positions[i - 1] + [1, 0, 0] elif step == 'x-': positions[i] = positions[i - 1] + [-1, 0, 0] elif step == 'y+': positions[i] = positions[i - 1] + [0, 1, 0] elif step == 'y-': positions[i] = positions[i - 1] + [0, -1, 0] elif step == 'z+': positions[i] = positions[i - 1] + [0, 0, 1] elif step == 'z-': positions[i] = positions[i - 1] + [0, 0, -1] return positions # Number of steps steps = 1000 positions = random_walk_3d(steps) # Plot the random walk fig = plt.figure(figsize=(10, 10)) ax = fig.add_subplot(111, projection='3d') ax.plot(positions[:, 0], positions[:, 1], positions[:, 2], label='3D Random Walk') ax.set_xlabel('X Position') ax.set_ylabel('Y Position') ax.set_zlabel('Z Position') ax.set_title('3D Random Walk') ax.legend() plt.show()
输出
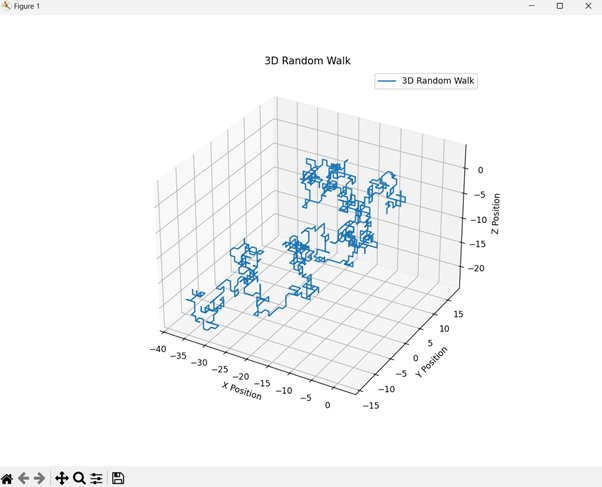
代码解释
- **向六个方向之一移动** - x+、x-、y+、y-、z+或z-。更新三维空间中的位置。
- **绘图** - 使用matplotlib绘制二维随机游走。使用mpl_toolkits.mplot3d进行三维可视化绘制三维随机游走。
四维随机游走的实现
以下代码用于在Python中实现四维随机游走:
import numpy as np import matplotlib.pyplot as plt from mpl_toolkits.mplot3d import Axes3D def random_walk_4d(steps): """Generate a 4D random walk.""" positions = np.zeros((steps, 4)) for i in range(1, steps): direction = np.random.choice(['x+', 'x-', 'y+', 'y-', 'z+', 'z-', 'w+', 'w-']) if direction == 'x+': positions[i] = positions[i - 1] + [1, 0, 0, 0] elif direction == 'x-': positions[i] = positions[i - 1] + [-1, 0, 0, 0] elif direction == 'y+': positions[i] = positions[i - 1] + [0, 1, 0, 0] elif direction == 'y-': positions[i] = positions[i - 1] + [0, -1, 0, 0] elif direction == 'z+': positions[i] = positions[i - 1] + [0, 0, 1, 0] elif direction == 'z-': positions[i] = positions[i - 1] + [0, 0, -1, 0] elif direction == 'w+': positions[i] = positions[i - 1] + [0, 0, 0, 1] elif direction == 'w-': positions[i] = positions[i - 1] + [0, 0, 0, -1] return positions # Number of steps steps = 1000 positions = random_walk_4d(steps) # Plot a 4D random walk by projecting onto 3D fig = plt.figure(figsize=(10, 10)) # 3D projection using first three dimensions ax1 = fig.add_subplot(121, projection='3d') ax1.plot(positions[:, 0], positions[:, 1], positions[:, 2], label='Projection: X-Y-Z') ax1.set_xlabel('X Position') ax1.set_ylabel('Y Position') ax1.set_zlabel('Z Position') ax1.set_title('4D Random Walk (Projection)') ax1.legend() # Another 3D projection using last three dimensions ax2 = fig.add_subplot(122, projection='3d') ax2.plot(positions[:, 1], positions[:, 2], positions[:, 3], label='Projection: Y-Z-W') ax2.set_xlabel('Y Position') ax2.set_ylabel('Z Position') ax2.set_zlabel('W Position') ax2.set_title('4D Random Walk (Projection)') ax2.legend() plt.show()
输出
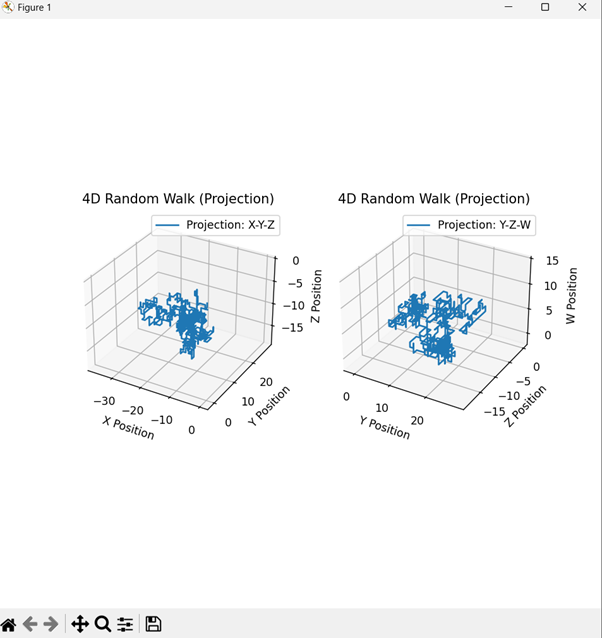
代码解释
- **random_walk_4d函数** - 向八个方向之一移动:x+、x-、y+、y-、z+、z-、w+、w-。更新四维空间中的位置。
- **绘图** - 由于四维数据太复杂而无法直接可视化,我们将其映射到三维空间,以便我们可以很好地可视化。第一个子图投影到X-Y-Z空间。第二个子图投影到Y-Z-W空间。
- **可视化** - 在这些三维投影中,游走的演变是清晰的——你可以从这里了解四维游走的行为。
python_projects_from_basic_to_advanced.htm
广告