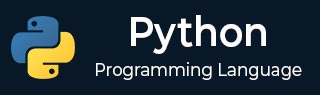
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策制定
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 修改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 对列表排序
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组项
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合项
- Python - 添加集合项
- Python - 删除集合项
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 修改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 对数组排序
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
使用 Python 编程截取屏幕截图
在某些情况下,例如自动化测试或制作故障纪录片和教学内容,程序化截屏方法可能很有用。作为一门动态语言,Python 拥有多个用户友好的库,例如 pytest;在这些库中,pyscreenshot 被认为是最简单、最快速的库之一。使用此工具,可以捕获整个屏幕或屏幕的一部分。
安装
在开始编写代码之前,需要安装 pyscreenshot 库。这可以通过 pip 轻松完成。
语法
pip install pyscreenshot
此外,请确保在系统中安装了 Python 库 pillow,它将帮助您获得图像处理功能。
语法
pip install pillow
基本截图
截取屏幕截图最简单的方法是捕获整个屏幕。ImageGrab.grab() 捕获整个屏幕并将其作为图像对象返回。screenshot.show() 在默认的图像查看器中打开捕获的屏幕截图。
示例
import pyscreenshot as ImageGrab # Capture full screen screenshot = ImageGrab.grab() # Save the screenshot to a file screenshot.save('screenshot.png') # You can change the file name and extension as needed # Display the screenshot screenshot.show()
输出
屏幕截图将在默认的图像查看器中显示。
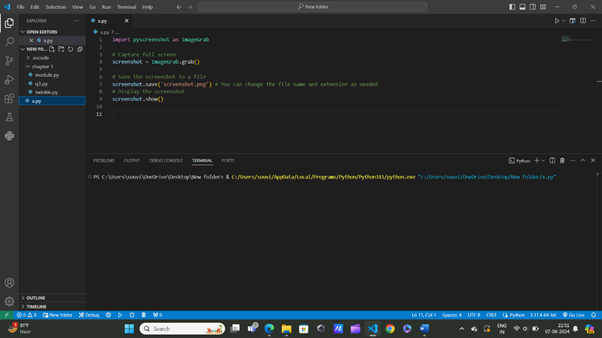
捕获特定区域
有时,您只需要屏幕的一部分,而不是整个部分。在这种情况下,您可以指定捕获特定区域。bbox 是一个元组,定义了要捕获的区域,而 ImageGrab.grab(bbox=bbox) 则捕获指定的区域。
示例
import pyscreenshot as ImageGrab # Define the bounding box (left_x, top_y, right_x, bottom_y) bbox = (10, 10, 500, 500) # Capture a specific region screenshot = ImageGrab.grab(bbox=bbox) # Display the screenshot screenshot.show()
输出
定义的区域将在默认的图像查看器中显示。
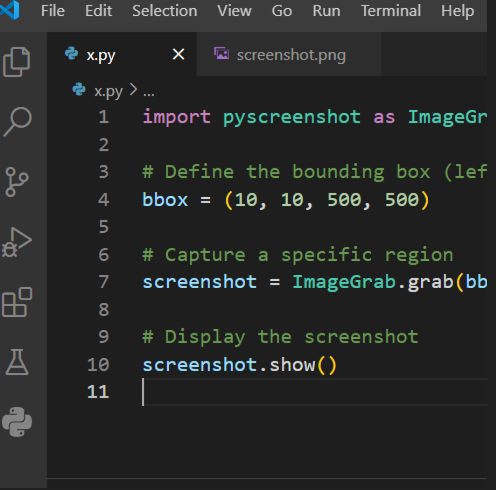
保存屏幕截图
要将捕获的屏幕截图保存到文件,请使用 save 方法。screenshot.save('screenshot.png') 将屏幕截图保存到名为 screenshot.png 的文件中。
示例
import pyscreenshot as ImageGrab # Capture full screen screenshot = ImageGrab.grab() # Save the screenshot to a file screenshot.save('screenshot.png')
输出
屏幕截图将保存为当前工作目录中的 screenshot.png。
在截取屏幕截图之前添加延迟
在捕获屏幕截图之前引入延迟在各种场景中都很有用。time.sleep(5) 在截取屏幕截图之前暂停执行 5 秒。
示例
import pyscreenshot as ImageGrab import time # Delay for 5 seconds time.sleep(5) # Capture full screen screenshot = ImageGrab.grab() # Display the screenshot screenshot.show()
输出
屏幕截图将在延迟 5 秒后捕获,并在默认的图像查看器中显示。
连续截取多个屏幕截图
您可能需要快速连续捕获多个屏幕截图。循环捕获五个屏幕截图,每个屏幕截图都使用唯一的文件名保存。time.sleep(2) 在每次捕获之间引入 2 秒的延迟。
示例
import pyscreenshot as ImageGrab import time for i in range(5): # Capture full screen screenshot = ImageGrab.grab() # Save the screenshot to a file screenshot.save(f'screenshot_{i}.png') # Delay for 2 seconds time.sleep(2)
输出
五个屏幕截图将保存为 screenshot_0.png、screenshot_1.png、screenshot_2.png、screenshot_3.png 和 screenshot_4.png。
请注意,这将创建多个图像屏幕截图。
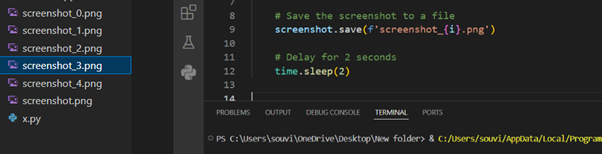
在屏幕截图中添加白色文本
有时,您可能希望在屏幕截图中添加文本以进行注释或标记。以下是使用 Python 中的 Pillow 库将白色文本添加到屏幕截图的示例。此示例捕获屏幕截图,向其添加白色文本,然后保存并显示修改后的图像。
安装
在开始编写代码之前,您必须安装 pillow 库。这可以使用 pip 轻松完成。
pip install pillow
示例
from PIL import Image, ImageDraw, ImageFont import pyscreenshot as ImageGrab # Define the text to add x = "Hello, World!" try: # Capture the screenshot screenshot = ImageGrab.grab() # Create an ImageDraw object draw = ImageDraw.Draw(screenshot) # Load a font try: # Use a truetype font (you can download a .ttf file and specify the path here) font = ImageFont.truetype("arial.ttf", size=50) except IOError: # Fallback to default font if the specified font is not available font = ImageFont.load_default() # Define the position and color for the text text_position = (50, 50) # Adjusted position text_color = "white" # Change text color to white # Add text to the screenshot draw.text(text_position, x, font=font, fill=text_color) # Save the screenshot with text screenshot.save('screenshot_with_text.png') # Display the screenshot screenshot.show() print("Screenshot captured and saved successfully.") except Exception as e: print(f"An error occurred: {e}")
输出
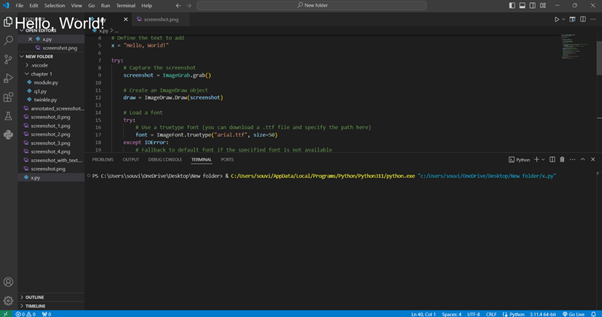
结论
在本指南中,我们向您展示了使用 Python 中的 **pyscreenshot** 捕获屏幕截图的各种技术。从基本的全屏捕获到目标特定区域、保存文件、添加延迟以及连续拍摄多张屏幕截图,**pyscreenshot** 被证明是自动化和教学内容创建的通用工具。