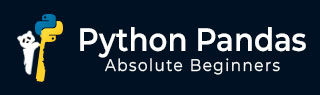
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础知识
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板(Panel)
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 处理 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引的高级重索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视表
- Python Pandas - 透视表
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和分类
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复数据
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - 时间差
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 访问 DataFrame
Pandas DataFrame 是一种二维带标签的数据结构,具有行和列标签,它看起来和工作方式类似于数据库或电子表格中的表格。为了使用 DataFrame 标签,pandas 提供了简单的工具来使用索引访问和修改行和列,即 DataFrame 的index 和columns 属性。
在本教程中,我们将学习如何使用 DataFrame 的index 和columns 属性来访问和操作 DataFrame 的行和列。
访问 DataFrame 行
Pandas 的DataFrame.index 属性用于访问 DataFrame 的行标签。它返回一个索引对象,其中包含与 DataFrame 每行中表示的数据相对应的标签序列。这些标签可以是整数、字符串或其他可哈希类型。
示例:访问 DataFrame 行标签
以下示例使用pd.index 属性访问 DataFrame 行标签。
import pandas as pd # Create a DataFrame df = pd.DataFrame({ 'Name': ['Steve', 'Lia', 'Vin', 'Katie'], 'Age': [32, 28, 45, 38], 'Gender': ['Male', 'Female', 'Male', 'Female'], 'Rating': [3.45, 4.6, 3.9, 2.78]}, index=['r1', 'r2', 'r3', 'r4']) # Access the rows of the DataFrame result = df.index print('Output Accessed Row Labels:', result)
以下是上述代码的输出 -
Output Accessed Row Labels: Index(['r1', 'r2', 'r3', 'r4'], dtype='object')
示例:修改 DataFrame 行索引
此示例访问并修改 Pandas DataFrame 的行标签。
import pandas as pd # Create a DataFrame df = pd.DataFrame({ 'Name': ['Steve', 'Lia', 'Vin', 'Katie'], 'Age': [32, 28, 45, 38], 'Gender': ['Male', 'Female', 'Male', 'Female'], 'Rating': [3.45, 4.6, 3.9, 2.78]}, index=['r1', 'r2', 'r3', 'r4']) # Display the Input DataFrame print('Input DataFrame:\n', df) # Modify the Row labels of the DataFrame df.index = [100, 200, 300, 400] print('Output Modified DataFrame with the updated index labels:\n', df)
执行上述代码后,您将获得以下输出 -
Input DataFrame:
Name | Age | Gender | Rating | |
---|---|---|---|---|
r1 | Steve | 32 | Male | 3.45 |
r2 | Lia | 28 | Female | 4.60 |
r3 | Vin | 45 | Male | 3.90 |
r4 | Katie | 38 | Female | 2.78 |
Name | Age | Gender | Rating | |
---|---|---|---|---|
100 | Steve | 32 | Male | 3.45 |
200 | Lia | 28 | Female | 4.60 |
300 | Vin | 45 | Male | 3.90 |
400 | Katie | 38 | Female | 2.78 |
访问 DataFrame 列
Pandas 的pd.columns 属性用于访问 DataFrame 中列的标签。您可以类似于我们处理行标签的方式来访问和修改这些列标签。
示例:访问 DataFrame 列标签
以下示例使用pd.columns 属性访问 DataFrame 列标签。
import pandas as pd # Create a DataFrame df = pd.DataFrame({ 'Name': ['Steve', 'Lia', 'Vin', 'Katie'], 'Age': [32, 28, 45, 38], 'Gender': ['Male', 'Female', 'Male', 'Female'], 'Rating': [3.45, 4.6, 3.9, 2.78]}, index=['r1', 'r2', 'r3', 'r4']) # Access the column labels of the DataFrame result = df.columns print('Output Accessed column Labels:', result)
以下是上述代码的输出 -
Output Accessed column Labels: Index(['Name', 'Age', 'Gender', 'Rating'], dtype='object')
示例:修改 DataFrame 列标签
此示例使用pd.columns 属性访问并修改 DataFrame 列标签。
import pandas as pd # Create a DataFrame df = pd.DataFrame({ 'Name': ['Steve', 'Lia', 'Vin', 'Katie'], 'Age': [32, 28, 45, 38], 'Gender': ['Male', 'Female', 'Male', 'Female'], 'Rating': [3.45, 4.6, 3.9, 2.78]}, index=['r1', 'r2', 'r3', 'r4']) # Display the Input DataFrame print('Input DataFrame:\n', df) # Modify the Column labels of the DataFrame df.columns = ['Col1', 'Col2', 'Col3', 'Col4'] print('Output Modified DataFrame with the updated Column Labels\n:', df)
以下是上述代码的输出 -
Input DataFrame:
Name | Age | Gender | Rating | |
---|---|---|---|---|
r1 | Steve | 32 | Male | 3.45 |
r2 | Lia | 28 | Female | 4.60 |
r3 | Vin | 45 | Male | 3.90 |
r4 | Katie | 38 | Female | 2.78 |
Col1 | Col2 | Col3 | Col4 | |
---|---|---|---|---|
r1 | Steve | 32 | Male | 3.45 |
r2 | Lia | 28 | Female | 4.60 |
r3 | Vin | 45 | Male | 3.90 |
r4 | Katie | 38 | Female | 2.78 |
广告