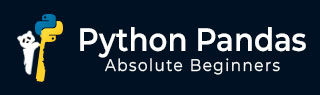
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - Panel
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 级联
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 使用文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多索引
- Python Pandas - 多索引基础
- Python Pandas - 使用多索引进行索引
- Python Pandas - 使用多索引进行高级重新索引
- Python Pandas - 重命名多索引标签
- Python Pandas - 对多索引排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和排序
- Python Pandas - 比较分类数据
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 警告和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 将数据写入 Excel 文件
Pandas 是 Python 中的一个数据分析库,广泛用于处理来自各种格式的结构化数据,包括 CSV、SQL 和 Excel 文件。该库的一个关键特性是您可以使用to_excel()方法轻松地将数据从 Pandas DataFrame 和 Series 直接导出到 Excel 电子表格中。
Pandas 中的to_excel()方法允许您将 DataFrame 或 Series 中的数据导出到 Excel 文件。此方法提供了指定各种参数的灵活性,例如文件路径、工作表名称、格式选项等等。
在本教程中,我们将学习如何使用pandas.to_excel()方法将 Pandas 对象中的数据写入 Excel 文件。
将单个 DataFrame 写入 Excel
只需调用带有 Excel 文件名和可选工作表名的DataFrame.to_excel()方法,即可直接将 Pandas DataFrame 对象的内容导出到 Excel 文件的工作表中。
示例
以下是如何使用DataFrame.to_excel()方法将 Pandas DataFrame 的内容写入 Excel 文件的基本示例。
import pandas as pd # Create a DataFrame df = pd.DataFrame([[5, 2], [4, 1]],index=["One", "Two"],columns=["Rank", "Subjects"]) # Display the DataFrame print("DataFrame:\n", df) # Export DataFrame to Excel df.to_excel('Basic_example_output.xlsx') print('The Basic_example_output.xlsx file is saved successfully..')
以下是上述代码的输出:
DataFrame:
Rank | Subjects | |
---|---|---|
One | 5 | 2 |
Two | 4 | 1 |
注意:执行每个代码后,您可以在工作目录中找到创建的输出文件。
将多个 DataFrame 写入不同的工作表
可以使用ExcelWriter类将多个 DataFrame 写入同一个 Excel 文件中的不同工作表。
示例
以下是如何使用ExcelWriter类和df.to_excel()方法将多个 DataFrame 写入同一个 Excel 文件中不同工作表的示例。
import pandas as pd df1 = pd.DataFrame( [[5, 2], [4, 1]], index=["One", "Two"], columns=["Rank", "Subjects"] ) df2 = pd.DataFrame( [[15, 21], [41, 11]], index=["One", "Two"], columns=["Rank", "Subjects"] ) print("DataFrame 1:\n", df1) print("DataFrame 2:\n", df2) with pd.ExcelWriter('output_multiple_sheets.xlsx') as writer: df1.to_excel(writer, sheet_name='Sheet_name_1') df2.to_excel(writer, sheet_name='Sheet_name_2') print('The output_multiple_sheets.xlsx file is saved successfully..')
以下是上述代码的输出:
DataFrame 1:
Rank | Subjects | |
---|---|---|
One | 5 | 2 |
Two | 4 | 1 |
Rank | Subjects | |
---|---|---|
One | 15 | 21 |
Two | 41 | 11 |
将数据追加到现有的 Excel 文件
可以使用ExcelWriter和mode='a'将 DataFrame 的内容追加到现有的 Excel 文件中。
示例
以下示例演示如何将 DataFrame 的内容追加到现有的 Excel 文件。
import pandas as pd # Create a new DataFrame data3 = { 'Rank': [7, 8, 9], 'Subjects': ['Music', 'Art', 'Literature'] } df3 = pd.DataFrame([[51, 11], [21, 38]],index=["One", "Two"],columns=["Rank", "Subjects"]) # Append the DataFrame to an existing Excel file with pd.ExcelWriter('output_multiple_sheets.xlsm', mode='a') as writer: df3.to_excel(writer, sheet_name='Sheet_name_3', index=False) print('The output_multiple_sheets.xlsm file is saved successfully with the appended sheet..')
以下是上述代码的输出:
The output_multiple_sheets.xlsm file is saved successfully with the appended sheet..
将 Excel 文件写入内存
可以通过使用BytesIO或StringIO以及ExcelWriter将 Excel 文件写入内存(类似缓冲区的对象),而不是将其保存到磁盘。
示例
以下示例演示如何使用BytesIO和ExcelWriter类将 Excel 文件写入内存对象。
import pandas as pd from io import BytesIO df = pd.DataFrame( [[5, 2], [4, 1]], index=["One", "Two"], columns=["Rank", "Subjects"] ) print("DataFrame :\n", df) # Create a BytesIO object bio = BytesIO() # Write the DataFrame to the BytesIO buffer with pd.ExcelWriter(bio, engine='xlsxwriter') as writer: df.to_excel(writer, sheet_name='Sheet1') # Get the Excel file from memory bio.seek(0) excel_data = bio.read() print('The Excel file is save in Memory successfully..')
以下是上述代码的输出:
DataFrame :
Rank | Subjects | |
---|---|---|
One | 5 | 2 |
Two | 4 | 1 |
选择 Excel Writer 引擎
Pandas 支持多个用于写入 Excel 文件的引擎,例如openpyxl和xlsxwriter。您可以根据需要使用DataFrame.to_excel()方法的engine参数显式指定引擎。并确保您已在系统中安装了所需的引擎。
示例
此示例演示使用DataFrame.to_excel()方法的engine参数保存具有指定引擎的 Excel 文件。
import pandas as pd from io import BytesIO df = pd.DataFrame( [[5, 2], [4, 1]], index=["One", "Two"], columns=["Rank", "Subjects"] ) # Write DataFrame using xlsxwriter engine df.to_excel('output_xlsxwriter.xlsx', sheet_name='Sheet1', engine='xlsxwriter') print('The output_xlsxwriter.xlsx is saved successfully..')
以下是上述代码的输出:
The output_xlsxwriter.xlsx is saved successfully..