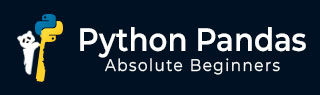
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境搭建
- Python Pandas - 基础
- Python Pandas - 数据结构简介
- Python Pandas - 索引对象
- Python Pandas - Panel (面板)
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片Series对象
- Python Pandas - Series对象的属性
- Python Pandas - Series对象的算术运算
- Python Pandas - 将Series转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问DataFrame
- Python Pandas - 切片DataFrame对象
- Python Pandas - 修改DataFrame
- Python Pandas - 从DataFrame中删除行
- Python Pandas - DataFrame的算术运算
- Python Pandas - I/O工具
- Python Pandas - I/O工具
- Python Pandas - 使用CSV格式
- Python Pandas - 读取和写入JSON文件
- Python Pandas - 从Excel文件读取数据
- Python Pandas - 将数据写入Excel文件
- Python Pandas - 使用HTML数据
- Python Pandas - 剪贴板
- Python Pandas - 使用HDF5格式
- Python Pandas - 与SQL的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重索引
- Python Pandas - 迭代
- Python Pandas - 级联
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 使用文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多层索引
- Python Pandas - 多层索引基础
- Python Pandas - 使用多层索引进行索引
- Python Pandas - 使用多层索引进行高级重索引
- Python Pandas - 重命名多层索引标签
- Python Pandas - 对多层索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视表
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复数据
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta (时间增量)
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 警告和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 切片Series对象
Pandas Series切片是从Series对象中选择一组元素的过程。Pandas中的Series是一个一维带标签的数组,它类似于一维ndarray(NumPy数组),但是带有标签,也称为索引。
Pandas Series切片与Python和NumPy切片非常相似,但它具有附加功能,例如基于位置和标签的切片。在本教程中,我们将学习Pandas Series对象的切片操作。
Pandas Series切片的基础
可以使用[:]运算符进行Series切片,它允许您通过指定的起始点和结束点从Series对象中选择元素子集。
以下是Series切片的语法:
Series[start:stop:step]:它以指定的步长值选择从start到end的元素。
Series[start:stop]:它以步长1选择从start到stop的元素。
Series[start:]:它以步长1选择从start到对象末尾的元素。
Series[:stop]:它以步长1选择从开头到stop的元素。
Series[:]:它选择Series对象中的所有元素。
按位置切片Series
Pandas Series允许您根据元素的位置(即索引值)选择元素,就像Python列表对象一样。
示例:从Series中切片一系列值
以下示例演示如何使用位置从Series对象中切片一系列值。
import pandas as pd import numpy as np data = np.array(['a', 'b', 'c', 'd']) s = pd.Series(data) # Display the Original series print('Original Series:',s, sep='\n') # Slice the range of values result = s[1:3] # Display the output print('Values after slicing the Series:', result, sep='\n')
以上代码的输出如下:
Original Series: 0 a 1 b 2 c 3 d dtype: object Values after slicing the Series: 1 b 2 c dtype: object
示例:从Series中切片前三个元素
此示例使用其位置(即索引值)检索Series中的前三个元素。
import pandas as pd s = pd.Series([1,2,3,4,5],index = ['a','b','c','d','e']) #retrieve the first three element print(s[:3])
其输出如下:
a 1 b 2 c 3 dtype: int64
示例:从Series中切片后三个元素
与上述示例类似,以下示例使用元素位置从Series中检索后三个元素。
import pandas as pd s = pd.Series([1,2,3,4,5],index = ['a','b','c','d','e']) #retrieve the last three element print(s[-3:])
其输出如下:
c 3 d 4 e 5 dtype: int64
按标签切片Series
Pandas Series类似于固定大小的Python dict,您可以通过索引标签获取和设置值。
示例:使用标签从Series中切片一组元素
以下示例通过切片Series的标签来检索多个元素。
import pandas as pd s = pd.Series([1,2,3,4,5],index = ['a','b','c','d','e']) # Slice multiple elements print(s['a':'d'])
其输出如下:
a 1 b 2 c 3 d 4 dtype: int64
示例:使用标签切片前三个元素
以下示例使用Series数据的标签切片前几个元素。
import pandas as pd s = pd.Series([1,2,3,4,5],index = ['a','b','c','d','e']) # Slice multiple elements print(s[:'c'])
其输出如下:
a 1 b 2 c 3 dtype: int64
切片后修改值
切片Series后,您还可以通过将新值赋给那些切片元素来修改值。
示例
以下示例演示如何通过切片访问范围值后修改Series值。
import pandas as pd s = pd.Series([1,2,3,4,5]) # Display the original series print("Original Series:\n",s) # Modify the values of first two elements s[:2] = [100, 200] print("Series after modifying the first two elements:",s)
以上代码的输出如下:
Original Series: 0 1 1 2 2 3 3 4 4 5 dtype: int64 Series after modifying the first two elements: 0 100 1 200 2 3 3 4 4 5 dtype: int64