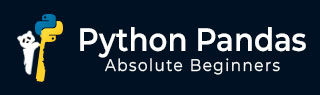
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象上的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 上的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 使用文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引的高级重索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和排序
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 重复标签
在 Pandas 中,Series 和 DataFrames 中的行和列标签都不需要唯一。如果数据集包含重复的索引标签,则我们将其称为重复标签,这可能导致某些操作(例如过滤、聚合或切片)出现意外结果。
Pandas 提供了几种方法来检测、管理和处理此类重复标签。在本教程中,我们将学习各种方法来检测、管理和处理 Pandas 中的重复标签。
检查唯一标签
要检查 DataFrame 的行或列标签是否唯一,可以使用 pandas 的 Index.is_unique 属性。如果它返回 False,则表示您的索引中存在重复标签。
示例
以下示例使用 pandas 的 Index.is_unique 属性来检查 DataFrame 的唯一标签。
import pandas as pd # Creating a DataFrame with duplicate row labels df = pd.DataFrame({"A": [0, 1, 2], 'B': [4, 1, 1]}, index=["a", "a", "b"]) # Display the Original DataFrame print("Original DataFrame:") print(df) # Check if the row index is unique print("Is row index is unique:",df.index.is_unique) # Check if the column index is unique print('Is column index is unique:',df.columns.is_unique)
以下是上述代码的输出 -
Original DataFrame:
A | B | |
---|---|---|
a | 0 | 4 |
a | 1 | 1 |
b | 2 | 1 |
检测重复标签
Index.duplicated() 方法用于检测 Pandas 对象的重复标签,它返回一个布尔数组,指示索引中的每个标签是否重复。
示例
以下示例使用 Index.duplicated() 方法来检测 Pandas DataFrame 的重复行标签。
import pandas as pd # Creating a DataFrame with duplicate row labels df = pd.DataFrame({"A": [0, 1, 2], 'B': [4, 1, 1]}, index=["a", "a", "b"]) # Display the Original DataFrame print("Original DataFrame:") print(df) # Identify duplicated row labels print('Duplicated Row Labels:', df.index.duplicated())
以下是上述代码的输出 -
Original DataFrame:
A | B | |
---|---|---|
a | 0 | 4 |
a | 1 | 1 |
b | 2 | 1 |
拒绝重复标签
Pandas 提供了拒绝重复标签的功能。默认情况下,pandas 允许重复标签,但您可以通过设置 .set_flags(allows_duplicate_labels=False) 来禁止它们。这可以应用于 Series 和 DataFrames。如果 pandas 检测到重复标签,它将引发 DuplicateLabelError。
示例
以下示例演示了创建不允许重复标签的 Pandas Series 对象。
import pandas as pd # Create a Series with duplicate labels and disallow duplicates try: pd.Series([0, 1, 2], index=["a", "b", "b"]).set_flags(allows_duplicate_labels=False) except pd.errors.DuplicateLabelError as e: print(e)
以下是上述代码的输出 -
Index has duplicates. positions label b [1, 2]
广告