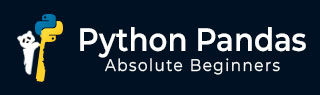
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 处理 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引的基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引进行高级重索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视表
- Python Pandas - 透视表
- Python Pandas - 堆叠和解堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和排序
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复数据
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - 时间增量
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 警告和注意事项
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 使用 CSV 格式
Python 中的 Pandas 库提供了大量用于读取和写入各种格式数据的函数。最常用的格式之一是 CSV(逗号分隔值),它是一种用于存储表格数据的流行格式。
CSV 文件是一个纯文本文件,其中数据值以逗号分隔,以纯文本格式表示表格数据。CSV 文件具有.csv扩展名。下面您可以看到 CSV 文件中的数据是什么样的 -
Sr.no,Name,Gender,Age 1,"Braund,male,22 2,"Cumings,female,38 3,"Heikkinen,female,26 4,"Futrelle,female,35
在本教程中,我们将学习如何使用 Pandas 处理 CSV 文件,包括读取和写入 CSV 文件,以及读取 CSV 文件的替代方法。
读取 CSV 文件
pandas.read_csv() 函数用于将 CSV 格式文件读取到 Pandas DataFrame 或 TextFileReader 中。此函数接受 URL 或本地文件路径以将数据加载到 Pandas 环境中。
示例
以下示例演示了如何使用pandas.read_csv() 函数读取 CSV 数据。这里我们使用StringIO将 CSV 字符串加载到类似文件对象中。
import pandas as pd # Import StringIO to load a file-like object for reading CSV from io import StringIO # Create string representing CSV data data = """Name,Gender,Age Braund,male,22 Cumings,female,38 Heikkinen,female,26 Futrelle,female,35""" # Use StringIO to convert the string data into a file-like object obj = StringIO(data) # read CSV into a Pandas DataFrame df = pd.read_csv(obj) print(df)
以下是上述代码的输出 -
Name | Gender | Age | |
---|---|---|---|
0 | Braund | male | 22 |
1 | Cumings | female | 38 |
2 | Heikkinen | female | 26 |
3 | Futrelle | female | 35 |
读取 CSV 文件的替代方法
除了pd.read_csv() 函数外,Pandas 还提供了一种读取各种格式数据的替代方法,即pd.read_table() 函数。
pd.read_table() 函数用于将通用分隔文件(如 CSV、TSV 或其他分隔符分隔格式)读取到 Pandas DataFrame 中。它是加载 CSV 格式的最佳替代方案,并且此函数可以使用sep参数轻松处理各种分隔符。此外,此函数支持迭代或将文件分成块。
示例
此示例显示了一种使用pd.read_table() 函数将 CSV 数据加载到 Pandas DataFrame 中的替代方法。在这里,您需要使用sep参数指定分隔符以读取逗号分隔值 (CSV)。
import pandas as pd url ="blood_pressure.csv" # read CSV into a Pandas DataFrame using the read_table() function df = pd.read_table(url,sep=',') print(df.head(5))
以下是上述代码的输出 -
Patient | Sex | Age Group | BP Before | BP After | |
---|---|---|---|---|---|
0 | 1 | Male | 30-45 | 143 | 153 |
1 | 2 | Male | 30-45 | 163 | 170 |
2 | 3 | Male | 30-45 | 153 | 168 |
3 | 4 | Male | 30-45 | 153 | 142 |
4 | 5 | Male | 30-45 | 146 | 141 |
写入 CSV 文件
Pandas 提供了一个名为pd.to_csv() 的函数,用于使用 Pandas 数据结构(如 DataFrame 或 Series 对象)创建或写入 CSV 文件。此函数允许您将数据导出为 CSV 格式。
示例
这是一个演示如何使用pd.to_csv() 函数将 Pandas DataFrame 写入 CSV 文件的示例。
import pandas as pd # dictionary of lists d = {'Car': ['BMW', 'Lexus', 'Audi', 'Mercedes', 'Jaguar', 'Bentley'], 'Date_of_purchase': ['2024-10-10', '2024-10-12', '2024-10-17', '2024-10-16', '2024-10-19', '2024-10-22']} # creating dataframe from the above dictionary of lists dataFrame = pd.DataFrame(d) print("Original DataFrame:\n",dataFrame) # write dataFrame to SalesRecords CSV file dataFrame.to_csv("Output_written_CSV_File.csv") # display the contents of the output csv print("The output csv file written successfully and generated...")
以下是上述代码的输出 -
Original DataFrame:
Car | Date of Purchase | |
---|---|---|
0 | BMW | 2024-10-10 |
1 | Lexus | 2024-10-12 |
2 | Audi | 2024-10-17 |
3 | Mercedes | 2024-10-16 |
4 | Jaguar | 2024-10-19 |
5 | Bentley | 2024-10-22 |
如果您在执行上述代码后访问您的工作目录,您将看到创建的名为Output_written_CSV_File.csv的 CSV 文件。