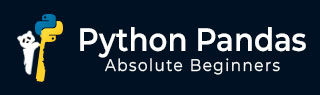
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 处理 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引进行高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视表
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 类别数据
- Python Pandas - 类别数据
- Python Pandas - 类别数据的排序和分类
- Python Pandas - 类别数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复数据
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 排序
排序是在 Pandas 中处理数据时一项基本的操作,无论您是在组织行、列还是特定值。排序可以帮助您以有意义的方式排列数据,以便更好地理解和轻松分析。
Pandas 提供了强大的工具来有效地对数据进行排序,这可以通过标签或实际值来完成。在本教程中,我们将探讨 Pandas 中各种数据排序方法,从基本的按索引或列标签排序到更高级的技术,如按多列排序和选择特定的排序算法。
Pandas 中的排序类型
Pandas 中有两种排序方式。它们是 -
按标签排序 - 这涉及根据索引标签对数据进行排序。
按值排序 - 这涉及根据 DataFrame 或 Series 中的实际值对数据进行排序。
按标签排序
要按索引标签排序,您可以使用sort_index()方法,通过传递轴参数和排序顺序,可以对数据结构对象进行排序。默认情况下,此方法会根据行标签按升序对 DataFrame 进行排序。
示例
让我们以一个基本的示例来说明如何使用sort_index()方法对 DataFrame 进行排序。
import pandas as pd import numpy as np unsorted_df = pd.DataFrame(np.random.randn(10,2),index=[1,4,6,2,3,5,9,8,0,7],columns = ['col2','col1']) print("Original DataFrame:\n", unsorted_df) # Sort the DataFrame by labels sorted_df=unsorted_df.sort_index() print("\nOutput Sorted DataFrame:\n", sorted_df)
其输出如下 -
Original DataFrame: col2 col1 1 1.116188 1.631727 4 0.287900 -1.097359 6 0.058885 -0.642273 2 -2.070172 0.148255 3 -1.458229 1.298907 5 -0.723663 2.220048 9 -1.271494 2.001025 8 -0.412954 -0.808688 0 0.922697 -0.429393 7 -0.476054 -0.351621 Output Sorted DataFrame: col2 col1 0 0.922697 -0.429393 1 1.116188 1.631727 2 -2.070172 0.148255 3 -1.458229 1.298907 4 0.287900 -1.097359 5 -0.723663 2.220048 6 0.058885 -0.642273 7 -0.476054 -0.351621 8 -0.412954 -0.808688 9 -1.271494 2.001025
示例 - 控制排序顺序
通过向 ascending 参数传递布尔值,可以控制排序顺序。让我们考虑以下示例来理解这一点。
import pandas as pd import numpy as np unsorted_df = pd.DataFrame(np.random.randn(10,2),index=[1,4,6,2,3,5,9,8,0,7],columns = ['col2','col1']) print("Original DataFrame:\n", unsorted_df) # Sort the DataFrame by ascending order sorted_df = unsorted_df.sort_index(ascending=False) print("\nOutput Sorted DataFrame:\n", sorted_df)
其输出如下 -
Original DataFrame: col2 col1 1 -0.668366 0.576422 4 0.605218 -0.066065 6 1.140478 0.236687 2 0.137617 0.312423 3 -0.055631 0.774057 5 0.108002 1.038820 9 -0.929134 -0.982358 8 -0.207542 -1.283386 0 -0.210571 -0.656371 7 -0.106388 0.672418 Output Sorted DataFrame: col2 col1 9 -0.929134 -0.982358 8 -0.207542 -1.283386 7 -0.106388 0.672418 6 1.140478 0.236687 5 0.108002 1.038820 4 0.605218 -0.066065 3 -0.055631 0.774057 2 0.137617 0.312423 1 -0.668366 0.576422 0 -0.210571 -0.656371
示例 - 对列进行排序
通过向 axis 参数传递值 0 或 1,可以在列标签上进行排序。默认情况下,axis=0,按行排序。让我们考虑以下示例来理解这一点。
import pandas as pd import numpy as np unsorted_df = pd.DataFrame(np.random.randn(6,4),index=[1,4,2,3,5,0],columns = ['col2','col1', 'col4', 'col3']) print("Original DataFrame:\n", unsorted_df) # Sort the DataFrame columns sorted_df=unsorted_df.sort_index(axis=1) print("\nOutput Sorted DataFrame:\n", sorted_df)
其输出如下 -
Original DataFrame: col2 col1 col4 col3 1 -0.828951 -0.798286 -1.794752 -0.082656 4 0.440243 -0.693218 -0.218277 -0.790168 2 1.017670 1.443679 -1.939119 -1.887223 3 -0.992471 -1.425046 0.651336 -0.278247 5 -0.103537 -0.879433 0.471838 0.860885 0 -0.222297 1.094805 0.501531 -0.580382 Output Sorted DataFrame: col1 col2 col3 col4 1 -0.798286 -0.828951 -0.082656 -1.794752 4 -0.693218 0.440243 -0.790168 -0.218277 2 1.443679 1.017670 -1.887223 -1.939119 3 -1.425046 -0.992471 -0.278247 0.651336 5 -0.879433 -0.103537 0.860885 0.471838 0 1.094805 -0.222297 -0.580382 0.501531
按实际值排序
与索引排序类似,可以使用sort_values()方法按实际值进行排序。此方法允许按一个或多个列进行排序。它接受一个“by”参数,该参数将使用 DataFrame 的列名来对值进行排序。
示例 - 对 Series 值进行排序
以下示例演示了如何使用sort_values()方法对 pandas Series 对象进行排序。
import pandas as pd panda_series = pd.Series([18, 95, 66, 12, 55, 0]) print("Unsorted Pandas Series: \n", panda_series) panda_series_sorted = panda_series.sort_values(ascending=True) print("\nSorted Pandas Series: \n", panda_series_sorted)
执行以上代码后,您将获得以下输出 -
Unsorted Pandas Series: 0 18 1 95 2 66 3 12 4 55 5 0 dtype: int64 Sorted Pandas Series: 5 0 3 12 0 18 4 55 2 66 1 95 dtype: int64
示例 - 对 DataFrame 值进行排序
以下示例演示了sort_values()方法在 DataFrame 对象上的工作原理。
import pandas as pd import numpy as np unsorted_df = pd.DataFrame({'col1':[2,9,5,0],'col2':[1,3,2,4]}) print("Original DataFrame:\n", unsorted_df) # Sort the DataFrame by values sorted_df = unsorted_df.sort_values(by='col1') print("\nOutput Sorted DataFrame:\n", sorted_df)
其输出如下 -
Original DataFrame: col1 col2 0 2 1 1 9 3 2 5 2 3 0 4 Output Sorted DataFrame: col1 col2 3 0 4 0 2 1 2 5 2 1 9 3
观察,col1 值已排序,相应的 col2 值和行索引将与 col1 一起更改。因此,它们看起来未排序。
示例 - 对多个列的值进行排序
您还可以通过向'by'参数传递列名列表来按多列排序。
import pandas as pd import numpy as np unsorted_df = pd.DataFrame({'col1':[2,1,0,1],'col2':[1,3,4,2]}) print("Original DataFrame:\n", unsorted_df) # Sort the DataFrame multiple columns by values sorted_df = unsorted_df.sort_values(by=['col1','col2']) print("\nOutput Sorted DataFrame:\n", sorted_df)
其输出如下 -
Original DataFrame: col1 col2 0 2 1 1 1 3 2 0 4 3 1 2 Output Sorted DataFrame: col1 col2 2 0 4 3 1 2 1 1 3 0 2 1
选择排序算法
Pandas 允许您使用sort_values()方法中的 kind 参数指定排序算法。您可以在“mergesort”、“heapsort”和“quicksort”之间进行选择。“mergesort”是唯一稳定的算法。
示例
以下示例使用sort_values()方法和特定算法对 DataFrame 进行排序。
import pandas as pd import numpy as np unsorted_df = pd.DataFrame({'col1':[2,5,0,1],'col2':[1,3,0,4]}) print("Original DataFrame:\n", unsorted_df) # Sort the DataFrame sorted_df = unsorted_df.sort_values(by='col1' ,kind='mergesort') print("\nOutput Sorted DataFrame:\n", sorted_df)
其输出如下 -
Original DataFrame: col1 col2 0 2 1 1 5 3 2 0 0 3 1 4 Output Sorted DataFrame: col1 col2 2 0 0 3 1 4 0 2 1 1 5 3