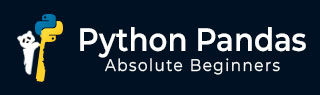
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引的高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 类别数据
- Python Pandas - 类别数据
- Python Pandas - 类别数据的排序和排序
- Python Pandas - 比较类别数据
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 警告和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 多级索引基础
多级索引也称为分层索引,它是 pandas 中一个强大的功能,允许您在低维结构(如 Series(一维)和 DataFrame(二维))中处理高维数据。使用多级索引,pandas 对象具有多个级别的索引标签。使用多级索引,您可以表示和操作具有多个索引级别的数据,从而更容易有效地处理复杂的数据集。
在本教程中,我们将学习多级索引的基础知识,包括如何创建具有多级索引的 Series 和 DataFrame,如何在多级索引轴上执行基本索引,以及如何使用多级索引对齐数据。
创建具有多级索引的 Pandas 对象
在 pandas 中创建多级索引对象有多种方法,包括从数组列表、元组、可迭代对象的乘积或直接从 DataFrame 创建。
以下是构建新的多级索引的辅助方法列表:
MultiIndex.from_arrays()
MultiIndex.from_product()
MultiIndex.from_tuples()
MultiIndex.from_frame()
从数组列表创建多级索引
通过使用pandas.MultiIndex.from_arrays()方法,我们可以从数组列表创建多级索引。
示例:从列表列表创建具有多级索引的 Series
以下示例演示了使用pandas.MultiIndex.from_arrays()方法创建具有多级索引的 Series 对象。
import pandas as pd import numpy as np # Create a 2D list list_2d = [["BMW", "BMW", "Lexus", "Lexus", "foo", "foo", "Audi", "Audi"], ["1", "2", "1", "2", "1", "2", "1", "2"]] # Create a MultiIndex object index = pd.MultiIndex.from_arrays(list_2d, names=["first", "second"]) # Creating a MultiIndexed Series s = pd.Series(np.random.randn(8), index=index) # Display the output Series print("Output MultiIndexed Series:\n",s)
以下是上述代码的输出:
Output MultiIndexed Series:
First | Second | |
---|---|---|
BMW | 1 | -1.334159 |
2 | -0.233286 | |
Lexus | 1 | 1.167558 |
2 | -0.875364 | |
foo | 1 | -0.338715 |
2 | 0.021517 | |
Audi | 1 | -0.272688 |
2 | 0.588359 |
从元组创建多级索引
Pandas MultiIndex.from_tuples()方法用于将元组列表转换为多级索引。
示例:从元组创建具有多级索引的 DataFrame
此示例演示了如何使用pandas.MultiIndex.from_tuples()方法创建具有多级索引的 DataFrame 对象。
import pandas as pd import numpy as np # Create a 2D list list_2d = [["BMW", "BMW", "Lexus", "Lexus", "foo", "foo", "Audi", "Audi"], ["1", "2", "1", "2", "1", "2", "1", "2"]] # Create a MultiIndex object tuples = list(zip(*list_2d )) index = pd.MultiIndex.from_tuples(tuples, names=["first", "second"]) # Creating a MultiIndexed DataFrame df = pd.DataFrame(np.random.randn(8, 4), index=index, columns=["A", "B", "C", "D"]) # Display the output Series print("Output MultiIndexed DataFrame:\n", df)
以下是上述代码的输出:
Output MultiIndexed DataFrame:
A | B | C | D | ||
---|---|---|---|---|---|
First | Second | ||||
BMW | 1 | -0.347846 | 1.011760 | -1.224244 | -1.225259 |
2 | 0.237115 | -1.316433 | 0.962960 | -1.008623 | |
Lexus | 1 | -1.806209 | 1.038973 | 0.246994 | -1.596616 |
2 | -0.325284 | -0.264822 | -0.735029 | 0.377645 | |
foo | 1 | 0.243560 | -0.408718 | 0.717466 | -1.446259 |
2 | -0.817704 | 0.711299 | 2.567860 | -1.054871 | |
Audi | 1 | 0.583519 | -0.007577 | 0.828928 | -0.826645 |
2 | 2.454626 | 1.558045 | 0.981507 | -0.148554 |
使用 from_product() 创建多级索引
Pandas MultiIndex.from_product()方法使用多个可迭代对象的笛卡尔积来创建多级索引。当您需要来自两个或多个可迭代对象的每个可能的元素组合时,它非常有用。
示例:使用 from_product() 创建具有多级索引的 DataFrame
此示例演示了如何使用 pandas MultiIndex.from_product()方法创建具有多级索引的 DataFrame。
import pandas as pd import numpy as np # Create a list of lits iterable = [[1, 2, 3], ['green', 'black']] # Create a MultiIndex object index = pd.MultiIndex.from_product(iterable, names=["number", "color"]) # Creating a MultiIndexed DataFrame df = pd.DataFrame(np.random.randn(6, 3), index=index, columns=["A", "B", "C"]) # Display the output Series print("Output MultiIndexed DataFrame:\n", df)
以下是上述代码的输出:
Output MultiIndexed DataFrame:
A | B | C | ||
---|---|---|---|---|
Number | Color | |||
1 | green | -1.174910 | -0.861695 | -0.026601 |
black | -2.824289 | 0.674870 | 1.132675 | |
2 | green | -0.285381 | -0.104188 | 1.993371 |
black | -0.926109 | -0.579404 | -1.119692 | |
3 | green | -3.278989 | -0.873407 | -1.359360 |
black | 0.735492 | 0.066735 | -0.099568 |
从 DataFrame 创建多级索引
Pandas MultiIndex.from_frame()方法用于从 DataFrame 创建多级索引。
示例:从 DataFrame 创建多级索引
此示例使用pd.MultiIndex.from_frame()方法直接从 DataFrame 创建多级索引对象。
import pandas as pd import numpy as np # Create a DataFrame df = pd.DataFrame([["BMW", 1], ["BMW", 2], ["Lexus", 1],["Lexus", 2]], columns=["first", "second"]) # Create a MultiIndex object index = pd.MultiIndex.from_frame(df) # Creating a MultiIndexed DataFrame df = pd.DataFrame(np.random.randn(4, 3), index=index, columns=["A", "B", "C"]) # Display the output Series print("Output MultiIndexed DataFrame:\n", df)
以下是上述代码的输出:
Output MultiIndexed DataFrame:
A | B | C | ||
---|---|---|---|---|
First | Second | |||
BMW | 1 | -0.662779 | -0.270775 | 0.129462 |
2 | -0.251308 | 1.920896 | 0.756204 | |
Lexus | 1 | -0.466133 | 0.590872 | 0.252439 |
2 | -1.226775 | -0.239043 | -0.023214 |
具有多级索引的轴的基本索引
使用多级索引进行索引用于以比常规索引更灵活的方式切片和选择数据。
示例:按索引级别选择数据
这是一个基本示例,演示了如何使用.loc[]方法索引具有多级索引的 Series 对象。
import pandas as pd import numpy as np # Creating MultiIndex from arrays arrays = [["bar", "bar", "baz", "baz", "foo", "foo", "qux", "qux"], ["one", "two", "one", "two", "one", "two", "one", "two"]] # Creating a list of tuples from the arrays tuples = list(zip(*arrays)) # Creating a MultiIndex from tuples index = pd.MultiIndex.from_tuples(tuples, names=["first", "second"]) # Creating a Series with MultiIndex s = pd.Series([2, 3, 1, 4, 6, 1, 7, 8], index=index) print("MultiIndexed Series:\n", s) # Indexing the MultiIndexed Series using .loc[] print("\nSelecting data at index ('bar', 'one') and column 'A':") print(s.loc[('bar', 'one')])
以下是上述代码的输出:
MultiIndexed Series:
First | Second | |
---|---|---|
bar | one | 2 |
two | 2 | |
one | 1 | 1 |
two | 4 | |
one | 1 | 6 |
two | 1 | |
one | 1 | 7 |
two | 8 |