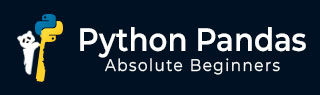
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构简介
- Python Pandas - 索引对象
- Python Pandas - Panel (面板)
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series (序列)
- Python Pandas - Series (序列)
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame (数据框)
- Python Pandas - DataFrame (数据框)
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 级联
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 使用文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多层索引
- Python Pandas - 多层索引基础
- Python Pandas - 使用多层索引进行索引
- Python Pandas - 使用多层索引的高级重新索引
- Python Pandas - 重命名多层索引标签
- Python Pandas - 对多层索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视表
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和分类
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据的计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta (时间差)
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 警告和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 布尔掩码
Pandas 中的布尔掩码是一种基于特定条件过滤数据的有用技术。它的工作原理是创建一个布尔掩码,并将其应用于 DataFrame 或 Series 以选择满足给定条件的数据。布尔掩码是一个 DataFrame 或 Series,其中每个元素都用 True 或 False 表示。当您将此布尔掩码应用于您的数据时,它将只选择满足定义条件的行或列。
在本教程中,我们将学习如何在 Pandas 中应用布尔掩码并根据索引和列值过滤数据。
使用布尔掩码选择数据
DataFrame 中的数据选择或过滤是通过创建定义行选择条件的布尔掩码来完成的。
示例
以下示例演示如何使用布尔掩码过滤数据。
import pandas as pd # Create a sample DataFrame df= pd.DataFrame({'Col1': [1, 3, 5, 7, 9], 'Col2': ['A', 'B', 'A', 'C', 'A']}) # Dispaly the Input DataFrame print('Original DataFrame:\n', df) # Create a boolean mask mask = (df['Col2'] == 'A') & (df['Col1'] > 4) # Apply the mask to the DataFrame filtered_data = df[mask] print('Filtered Data:\n',filtered_data)
以下是上述代码的输出:
Original DataFrame:
Col1 | Col2 | |
---|---|---|
0 | 1 | A |
1 | 3 | B |
2 | 5 | A |
3 | 7 | C |
4 | 9 | A |
Col1 | Col2 | |
---|---|---|
2 | 5 | A |
4 | 9 | A |
基于索引值掩码数据
可以通过为索引创建掩码来根据 DataFrame 的索引值过滤数据,以便您可以根据其位置或标签选择行。
示例
此示例使用 **df.isin()** 方法根据索引标签创建布尔掩码。
import pandas as pd # Create a DataFrame with a custom index df = pd.DataFrame({'A1': [10, 20, 30, 40, 50], 'A2':[9, 3, 5, 3, 2] }, index=['a', 'b', 'c', 'd', 'e']) # Dispaly the Input DataFrame print('Original DataFrame:\n', df) # Define a mask based on the index mask = df.index.isin(['b', 'd']) # Apply the mask filtered_data = df[mask] print('Filtered Data:\n',filtered_data)
以下是上述代码的输出:
Original DataFrame:
A1 | A2 | |
---|---|---|
a | 10 | 9 |
b | 20 | 3 |
c | 30 | 5 |
d | 40 | 3 |
e | 50 | 2 |
A1 | A2 | |
---|---|---|
b | 20 | 3 |
d | 40 | 3 |
基于列值掩码数据
除了根据索引值进行过滤外,您还可以使用布尔掩码根据特定的列值过滤数据。**df.isin()** 方法用于检查列中的值是否与值的列表匹配。
示例
以下示例演示如何创建和应用布尔掩码以根据 DataFrame 列值选择数据。
import pandas as pd # Create a DataFrame df= pd.DataFrame({'A': [1, 2, 3],'B': ['a', 'b', 'f']}) # Dispaly the Input DataFrame print('Original DataFrame:\n', df) # Define a mask for specific values in column 'A' and 'B' mask = df['A'].isin([1, 3]) | df['B'].isin(['a']) # Apply the mask using the boolean indexing filtered_data = df[mask] print('Filtered Data:\n', filtered_data)
以下是上述代码的输出:
Original DataFrame:
A | B | |
---|---|---|
0 | 1 | a |
1 | 2 | b |
2 | 3 | f |
A | B | |
---|---|---|
0 | 1 | a |
2 | 3 | f |
广告