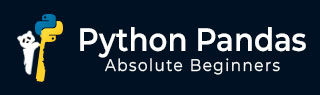
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础知识
- Python Pandas - 数据结构简介
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象上的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 上的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引进行高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和排序
- Python Pandas - 比较分类数据
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复数据
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 将 Series 转换为其他对象
Pandas Series 是一种一维类似数组的对象,包含任何类型的数据,例如整数、浮点数和字符串。数据元素与标签(索引)相关联。在某些情况下,您需要将 Pandas Series 转换为不同的格式以用于各种用例,例如创建列表、NumPy 数组、字典,甚至将 Series 转换为 DataFrame。
在本教程中,我们将学习 Pandas 中可用于将 Series 转换为不同格式(例如列表、NumPy 数组、字典、DataFrame 和字符串)的各种方法。
以下是将 Series 转换为其他格式的常用方法:
方法 | 描述 |
---|---|
to_list() | 将 Series 转换为 Python 列表。 |
to_numpy() | 将 Series 转换为 NumPy 数组。 |
to_dict() | 将 Series 转换为字典。 |
to_frame() | 将 Series 转换为 DataFrame。 |
to_string() | 将 Series 转换为字符串表示形式以进行显示。 |
将 Series 转换为列表
Series.to_list() 方法将 Pandas Series 转换为 Python 列表,其中 Series 的每个元素都成为返回列表中的一个元素。列表中每个元素的类型与 Series 中的类型相同。
示例
以下是使用 Series.to_list() 方法将 Pandas Series 转换为 Python 列表的示例。
import pandas as pd # Create a Pandas Series s = pd.Series([1, 2, 3]) # Convert Series to a Python list result = s.to_list() print("Output:",result) print("Output Type:", type(result))
以下是上述代码的输出:
Output: [1, 2, 3] Output Type: <class 'list'>
将 Series 转换为 NumPy 数组
Pandas Series.to_numpy() 方法可用于将 Pandas Series 转换为 NumPy 数组。此方法提供了其他功能,例如指定数据类型 (dtype)、处理缺失值 (na_value) 和控制结果是否应为副本或视图。
示例
此示例使用 Series.to_numpy() 方法将 Series 转换为 NumPy 数组。
import pandas as pd # Create a Pandas Series s = pd.Series([1, 2, 3]) # Convert Series to a NumPy Array result = s.to_numpy() print("Output:",result) print("Output Type:", type(result))
Output: [1, 2, 3] Output Type: <class 'numpy.ndarray'>
将 Pandas Series 转换为字典
Pandas Series.to_dict() 方法用于将 Series 转换为 Python 字典,其中每个标签(索引)都成为一个键,每个对应值都成为字典的值。
示例
以下示例使用 Series.to_dict() 方法将 Series 转换为 Python 字典。
import pandas as pd # Create a Pandas Series s = pd.Series([1, 2, 3], index=['a', 'b', 'c']) # Convert Series to a Python dictionary result = s.to_dict() print("Output:",result) print("Output Type:", type(result))
Output: {'a': 1, 'b': 2, 'c': 3} Output Type: <class 'dict'>
将 Series 转换为 DataFrame
Series.to_frame() 方法允许您将 Series 转换为 DataFrame。每个 Series 都成为 DataFrame 中的一列。此方法提供了一个 name 参数来设置结果 DataFrame 的列名。
示例
此示例使用 Series.to_frame() 方法将 Series 转换为具有单个列的 Pandas DataFrame。
import pandas as pd # Create a Pandas Series s = pd.Series([1, 2, 3], index=['a', 'b', 'c']) # Convert Series to a Pandas DataFrame result = s.to_frame(name='Numbers') print("Output:\n",result) print("Output Type:", type(result))
Output:
Numbers | |
---|---|
a | 1 |
b | 2 |
c | 3 |
将 Series 转换为 Python 字符串
要将 Pandas Series 对象转换为 Python 字符串,您可以使用 Series.to_string() 方法,该方法呈现 Series 的字符串表示形式。
此方法返回一个字符串,显示 Series 的索引和值。您可以使用各种参数(如 na_rep(表示缺失值)、header、index、float_format、length 等)自定义输出字符串。
示例
此示例使用 Series.to_string() 方法将 Series 转换为 Python 字符串表示形式。
import pandas as pd # Create a Pandas Series s = pd.Series([1, 2, 3], index=['r1', 'r2', 'r3']) # Convert Series to string representation result = s.to_string() print("Output:",repr(result)) print("Output Type:", type(result))
Output: 'r1 1\nr2 2\nr3 3' Output Type: <class 'str'>