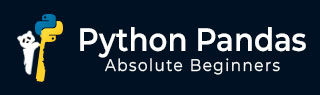
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象上的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 上的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 使用文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引的基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引的高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 类别数据
- Python Pandas - 类别数据
- Python Pandas - 类别数据的排序和分类
- Python Pandas - 比较类别数据
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 警告和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 熔化
Pandas 中的熔化是指将 DataFrame 从宽格式转换为长格式的过程。在宽格式中,数据分布在多个列中。简单来说,它会“反转”DataFrame 的列为行,这对于可视化和对数据集执行统计分析很有用。
Pandas 库提供了melt() 和wide_to_long() 函数,用于将 DataFrame 从宽格式转换为长格式。在本教程中,我们将学习 Pandas 中的melt() 和wide_to_long() 函数,以及如何使用这两种方法将 DataFrame 从宽格式转换为长格式。
Pandas 中的熔化
Pandas 中的melt() 函数将宽 DataFrame 转换为长格式。这只不过是“反转”DataFrame。
示例
以下示例演示了使用pandas.melt() 函数熔化一个简单的 DataFrame。
import pandas as pd # Create a DataFrame df = pd.DataFrame({'A': {0: 'a', 1: 'b', 2: 'c'},'B': {0: 1, 1: 3, 2: 5},'C': {0: 2, 1: 4, 2: 6}}) # Display the input DataFrame print('Input DataFrame:\n', df) # Melt the DataFrame melted_df = pd.melt(df, id_vars=['A'], value_vars=['B']) print('Output melted DataFrame:\n', melted_df)
以下是上述代码的输出 -
Input DataFrame:
A | B | C | |
---|---|---|---|
0 | a | 1 | 2 |
1 | b | 3 | 4 |
2 | c | 5 | 6 |
A | variable | value | |
---|---|---|---|
0 | a | B | 1 |
1 | b | B | 3 |
2 | c | B | 5 |
示例:在熔化时处理索引值
此示例演示了在使用pandas.melt() 函数熔化 DataFrame 时如何处理缺失值。
import pandas as pd # Create a DataFrame index = pd.MultiIndex.from_tuples([("person", "A"), ("person", "B")]) df= pd.DataFrame({ "first": ["John", "Mary"],"last": ["Doe", "Bo"], "height": [5.5, 6.0],"weight": [130, 150]}, index=index) # Display the input DataFrame print('Input DataFrame:\n', df) # Melt the DataFrame melted_df = pd.melt(df, id_vars=["first", "last"], ignore_index=False) print('Output melted DataFrame:\n', melted_df)
以下是上述代码的输出 -
Input DataFrame:
first | last | height | weight | ||
---|---|---|---|---|---|
person | A | John | Doe | 5.5 | 130 |
B | Mary | Bo | 6.0 | 150 |
first | last | variable | value | ||
---|---|---|---|---|---|
person | A | John | Doe | height | 5.5 |
B | Mary | Bo | height | 6.0 | |
A | John | Doe | weight | 130.0 | |
B | Mary | Bo | weight | 150.0 |
使用 wide_to_long() 熔化
pandas wide_to_long() 函数提供了对转换的更多控制。当您的列具有包含后缀的结构化命名模式时,它很有用。
示例
此示例使用wide_to_long() 函数执行高级熔化转换。
import pandas as pd # Create a DataFrame df = pd.DataFrame({'famid': [1, 1, 1, 2, 2, 2, 3, 3, 3], 'birth': [1, 2, 3, 1, 2, 3, 1, 2, 3], 'ht1': [2.8, 2.9, 2.2, 2, 1.8, 1.9, 2.2, 2.3, 2.1], 'ht2': [3.4, 3.8, 2.9, 3.2, 2.8, 2.4, 3.3, 3.4, 2.9]}) # Display the input DataFrame print('Input DataFrame:\n', df) # Melt the DataFrame using wide_to_long() long_df = pd.wide_to_long(df, stubnames='ht', i=['famid', 'birth'], j='age') print('Output Long Melted DataFrame:\n', long_df)
以下是上述代码的输出 -
Input DataFrame:
famid | birth | ht1 | ht2 | |
---|---|---|---|---|
0 | 1 | 1 | 2.8 | 3.4 |
1 | 1 | 2 | 2.9 | 3.8 |
2 | 1 | 3 | 2.2 | 2.9 |
3 | 2 | 1 | 2.0 | 3.2 |
4 | 2 | 2 | 1.8 | 2.8 |
5 | 2 | 3 | 1.9 | 2.4 |
6 | 3 | 1 | 2.2 | 3.3 |
7 | 3 | 2 | 2.3 | 3.4 |
8 | 3 | 3 | 2.1 | 2.9 |
ht | |||
---|---|---|---|
famid | birth | age | |
1 | 1 | 1 | 2.8 |
2 | 3.4 | ||
2 | 1 | 2.9 | |
2 | 3.8 | ||
3 | 1 | 2.2 | |
2 | 2.9 | ||
2 | 1 | 1 | 2.0 |
2 | 3.2 | ||
2 | 1 | 1.8 | |
2 | 2.8 | ||
3 | 1 | 1.9 | |
2 | 2.4 | ||
3 | 1 | 1 | 2.2 |
2 | 3.3 | ||
2 | 1 | 2.3 | |
2 | 3.4 | ||
3 | 1 | 2.1 | |
2 | 2.9 |
广告