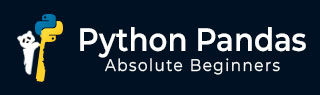
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引的高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视表
- Python Pandas - 透视表
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和排序
- Python Pandas - 分类数据比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 分类数据比较
比较分类数据是获得见解和理解数据不同类别之间关系的一项基本任务。在 Python 中,Pandas 提供了多种方法来使用比较运算符(==、!=、>、>=、< 和 <=)对分类数据执行比较。这些比较可以在三种主要场景中进行:
相等比较(== 和 !=)。
所有比较(==、!=、>、>=、< 和 <=)。
将分类数据与标量值进行比较。
需要注意的是,在具有不同类别的分类数据之间或在分类 Series 与列表状对象之间进行任何非相等比较都会引发TypeError。这是因为类别的顺序可以解释为两种方式,一种考虑顺序,另一种不考虑顺序。
在本教程中,我们将学习如何在 Python Pandas 库中使用比较运算符(如==、!=、>、>=、< 和<=)来比较分类数据。
分类数据的相等比较
在 Pandas 中,可以使用各种对象(如列表、数组或与分类数据长度相同的 Series 对象)对分类数据进行相等比较。
示例
以下示例演示了如何在分类 Series 和列表状对象之间执行相等和不等比较。
import pandas as pd from pandas.api.types import CategoricalDtype import numpy as np # Creating a categorical Series s = pd.Series([1, 2, 1, 1, 2, 3, 1, 3]).astype(CategoricalDtype([3, 2, 1], ordered=True)) # Creating another categorical Series for comparison s2 = pd.Series([2, 2, 2, 1, 1, 3, 3, 3]).astype(CategoricalDtype([3, 2, 1], ordered=True)) # Equality comparison print("Equality comparison (s == s2):") print(s == s2) print("\nInequality comparison (s != s2):") print(s != s2) # Equality comparison with a NumPy array print("\nEquality comparison with NumPy array:") print(s == np.array([1, 2, 3, 1, 2, 3, 2, 1]))
以下是上述代码的输出:
Equality comparison (s == s2): 0 False 1 True 2 False 3 True 4 False 5 True 6 False 7 True dtype: bool Inequality comparison (s != s2): 0 True 1 False 2 True 3 False 4 True 5 False 6 True 7 False dtype: bool Equality comparison with NumPy array: 0 True 1 True 2 False 3 True 4 True 5 True 6 False 7 False dtype: bool
分类数据的所有比较
Pandas 允许您在有序分类数据之间执行各种比较操作,包括(>、>=、<=、<)。
示例
此示例演示了如何在有序分类数据上执行非相等比较(>、>=、<=、<)。
import pandas as pd from pandas.api.types import CategoricalDtype import numpy as np # Creating a categorical Series s = pd.Series([1, 2, 1, 1, 2, 3, 1, 3]).astype(CategoricalDtype([3, 2, 1], ordered=True)) # Creating another categorical Series for comparison s2 = pd.Series([2, 2, 2, 1, 1, 3, 3, 3]).astype(CategoricalDtype([3, 2, 1], ordered=True)) # Greater than comparison print("Greater than comparison:\n",s > s2) # Less than comparison print("\nLess than comparison:\n",s < s2) # Greater than or equal to comparison print("\nGreater than or equal to comparison:\n",s >= s2) # Lessthan or equal to comparison print("\nLess than or equal to comparison:\n",s <= s2)
以下是上述代码的输出:
Greater than comparison: 0 True 1 False 2 True 3 False 4 False 5 False 6 True 7 False dtype: bool Less than comparison: 0 False 1 False 2 False 3 False 4 True 5 False 6 False 7 False dtype: bool Greater than or equal to comparison: 0 True 1 True 2 True 3 True 4 False 5 True 6 True 7 True dtype: bool Lessthan or equal to comparison: 0 False 1 True 2 False 3 True 4 True 5 True 6 False 7 True dtype: bool
将分类数据与标量进行比较
分类数据也可以使用所有比较运算符(==、!=、>、>=、< 和 <=)与标量值进行比较。分类值根据其类别的顺序与标量进行比较。
示例
以下示例演示了如何将分类数据与标量值进行比较。
import pandas as pd # Creating a categorical Series s = pd.Series([1, 2, 3]).astype(pd.CategoricalDtype([3, 2, 1], ordered=True)) # Compare to a scalar print("Comparing categorical data to a scalar:") print(s > 2)
以下是上述代码的输出:
Comparing categorical data to a scalar: 0 True 1 False 2 False dtype: bool
比较具有不同类别的分类数据
当比较两个具有不同类别或排序的分类 Series 时,将引发TypeError。
示例
以下示例演示了在两个具有不同类别或顺序的分类 Series 对象之间执行比较时如何处理TypeError。
import pandas as pd from pandas.api.types import CategoricalDtype import numpy as np # Creating a categorical Series s = pd.Series([1, 2, 1, 1, 2, 3, 1, 3]).astype(CategoricalDtype([3, 2, 1], ordered=True)) # Creating another categorical Series for comparison s3 = pd.Series([2, 2, 2, 1, 1, 3, 1, 2]).astype(CategoricalDtype(ordered=True)) try: print("Attempting to compare differently ordered two Series objects:") print(s > s3) except TypeError as e: print("TypeError:", str(e))
以下是上述代码的输出:
Attempting to compare differently ordered two Series objects: TypeError: Categoricals can only be compared if 'categories' are the same.
广告