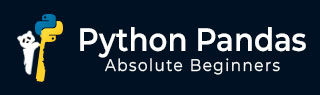
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础知识
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - Panel
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片Series对象
- Python Pandas - Series对象的属性
- Python Pandas - 数列对象的算术运算
- Python Pandas - 将Series转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问DataFrame
- Python Pandas - 切片DataFrame对象
- Python Pandas - 修改DataFrame
- Python Pandas - 从DataFrame中删除行
- Python Pandas - DataFrame的算术运算
- Python Pandas - I/O工具
- Python Pandas - I/O工具
- Python Pandas - 使用CSV格式
- Python Pandas - 读取和写入JSON文件
- Python Pandas - 从Excel文件读取数据
- Python Pandas - 将数据写入Excel文件
- Python Pandas - 使用HTML数据
- Python Pandas - 剪贴板
- Python Pandas - 使用HDF5格式
- Python Pandas - 与SQL的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引进行高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视表
- Python Pandas - 堆叠和解堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 数列对象的算术运算
Pandas Series是主要的 数据结构之一,它存储一维标记数据。数据可以是任何类型,例如整数、浮点数或字符串。使用Pandas Series的主要优点之一是能够以向量化方式执行算术运算。这意味着对Series的算术运算无需手动循环遍历元素。
在本教程中,我们将学习如何将加法(+)、减法(-)、乘法(*)和除法(/)等算术运算应用于单个Series或两个Series对象之间。
对Series与标量值的算术运算
Pandas Series对象的算术运算可以直接应用于整个Series元素,这意味着该运算将逐元素地对所有值执行。这与NumPy数组的操作方式非常相似。
以下是Pandas Series中常用算术运算的列表:
运算 | 示例 | 描述 |
---|---|---|
加法 | s + 2 | 将2加到每个元素 |
减法 | s - 2 | 从每个元素中减去2 |
乘法 | s * 2 | 将每个元素乘以2 |
除法 | s / 2 | 将每个元素除以2 |
幂运算 | s ** 2 | 将每个元素的幂提升到2 |
取模 | s % 2 | 求除以2的余数 |
地板除 | s // 2 | 除法并向下取整 |
示例
以下示例演示了如何将所有算术运算应用于带有标量值的Series对象。
import pandas as pd s = pd.Series([1,2,3,4,5],index = ['a','b','c','d','e']) # Display the Input Series print('Input Series\n',s) # Apply all Arithmetic Operation and Display the Results print('\nAddition:\n',s+2) print('\nSubtraction:\n', s-2) print('\nMultiplication:\n', s * 2) print('\nDivision:\n', s/2) print('\nExponentiation:\n', s**2) print('\nModulus:\n', s%2) print('\nFloor Division:\n', s//2)
以下是上述代码的输出:
Input Series a 1 b 2 c 3 d 4 e 5 dtype: int64 Addition: a 3 b 4 c 5 d 6 e 7 dtype: int64 Subtraction: a -1 b 0 c 1 d 2 e 3 dtype: int64 Multiplication: a 2 b 4 c 6 d 8 e 10 dtype: int64 Division: a 0.5 b 1.0 c 1.5 d 2.0 e 2.5 dtype: float64 Exponentiation: a 1 b 4 c 9 d 16 e 25 dtype: int64 Modulus: a 1 b 0 c 1 d 0 e 1 dtype: int64 Floor Division: a 0 b 1 c 1 d 2 e 2 dtype: int64
两个Series之间的算术运算
您可以在两个Series对象之间执行算术运算。Pandas会自动根据索引标签对齐数据。如果其中一个Series对象没有索引,而另一个有索引,则该索引的结果值将为NaN。
示例
此示例演示了对两个Series对象应用算术运算。
import pandas as pd s1 = pd.Series([1,2,3,4,5],index = ['a','b','c','d','e']) s2 = pd.Series([9, 8, 6, 5], index=['x','a','b','c']) # Apply all Arithmetic Operations and Display the Results print('\nAddition:\n',s1+s2) print('\nSubtraction:\n', s1-s2) print('\nMultiplication:\n', s1 * s2) print('\nDivision:\n', s1/s2)
以下是上述代码的输出:
Addition: a 9.0 b 8.0 c 8.0 d NaN e NaN x NaN dtype: float64 Subtraction: a -7.0 b -4.0 c -2.0 d NaN e NaN x NaN dtype: float64 Multiplication: a 8.0 b 12.0 c 15.0 d NaN e NaN x NaN dtype: float64 Division: a 0.125000 b 0.333333 c 0.600000 d NaN e NaN x NaN dtype: float64
广告