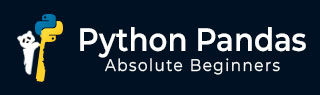
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板(Panel)
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引进行高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视表
- Python Pandas - 透视表
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和排序
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复数据
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 基本功能
Pandas 是 Python 中一个强大的数据操作库,提供用于处理 Series 和 DataFrame 格式数据的必要工具。这两种数据结构对于处理和分析大型数据集至关重要。
了解 Pandas 的基本功能,包括其属性和方法,对于有效地管理数据至关重要,这些属性和方法提供了对数据的宝贵见解,使理解和处理数据变得更容易。在本教程中,您将学习 Pandas 中一些基本属性和方法,这些属性和方法对于使用这些数据结构至关重要。
使用 Pandas 中的属性
Pandas 中的属性允许您访问有关 Series 和 DataFrame 对象的元数据。通过使用这些属性,您可以探索并轻松理解数据。
Series 和 DataFrame 属性
以下是 Series 和 DataFrame 对象中广泛使用的属性:
序号 | 属性和描述 |
---|---|
1 |
dtype 返回 Series 或 DataFrame 中元素的数据类型。 |
2 |
index 提供 Series 或 DataFrame 的索引(行标签)。 |
3 |
values 将 Series 或 DataFrame 中的数据作为 NumPy 数组返回。 |
4 |
shape 返回一个元组,表示 DataFrame 的维度(行,列)。 |
5 |
ndim 返回对象的维度数。Series 始终是 1D,DataFrame 是 2D。 |
6 |
size 给出对象中元素的总数。 |
7 |
empty 检查对象是否为空,如果为空则返回 True。 |
8 |
columns 提供 DataFrame 对象的列标签。 |
示例
让我们创建一个 Pandas Series 并探索这些属性操作。
import pandas as pd import numpy as np # Create a Series with random numbers s = pd.Series(np.random.randn(4)) # Exploring attributes print("Data type of Series:", s.dtype) print("Index of Series:", s.index) print("Values of Series:", s.values) print("Shape of Series:", s.shape) print("Number of dimensions of Series:", s.ndim) print("Size of Series:", s.size) print("Is Series empty?:", s.empty)
其输出如下:
Data type of Series: float64 Index of Series: RangeIndex(start=0, stop=4, step=1) Values of Series: [-1.02016329 1.40840089 1.36293022 1.33091391] Shape of Series: (4,) Number of dimensions of Series: 1 Size of Series: 4 Is Series empty?: False
示例
让我们看看下面的示例,并了解这些属性在 DataFrame 对象上的工作原理。
import pandas as pd import numpy as np # Create a DataFrame with random numbers df = pd.DataFrame(np.random.randn(3, 4), columns=list('ABCD')) print("DataFrame:") print(df) print("Results:") print("Data types:", df.dtypes) print("Index:", df.index) print("Columns:", df.columns) print("Values:") print(df.values) print("Shape:", df.shape) print("Number of dimensions:", df.ndim) print("Size:", df.size) print("Is empty:", df.empty)
执行上述代码后,您将获得以下输出:
DataFrame: A B C D 0 2.161209 -1.671807 -1.020421 -0.287065 1 0.308136 -0.592368 -0.183193 1.354921 2 -0.963498 -1.768054 -0.395023 -2.454112 Results: Data types: A float64 B float64 C float64 D float64 dtype: object Index: RangeIndex(start=0, stop=3, step=1) Columns: Index(['A', 'B', 'C', 'D'], dtype='object') Values: [[ 2.16120893 -1.67180742 -1.02042138 -0.28706468] [ 0.30813618 -0.59236786 -0.18319262 1.35492058] [-0.96349817 -1.76805364 -0.3950226 -2.45411245]] Shape: (3, 4) Number of dimensions: 2 Size: 12 Is empty: False
探索 Pandas 中的基本方法
Pandas 在这两种数据结构中都提供了多种基本方法,这使得轻松快速查看和理解数据变得容易。这些方法帮助您获得摘要并探索详细信息,而无需花费太多精力。
Series 和 DataFrame 方法
序号 | 方法和描述 |
---|---|
1 |
head(n) 返回对象的前 n 行。n 的默认值为 5。 |
2 |
tail(n) 返回对象的后 n 行。n 的默认值为 5。 |
3 |
info() 提供 DataFrame 的简洁摘要,包括索引 dtype 和列 dtype、非空值以及内存使用情况。 |
4 |
describe() 生成 DataFrame 或 Series 的描述性统计信息,例如计数、均值、标准差、最小值和最大值。 |
示例
现在让我们创建一个 Series 并查看 Series 基本方法的工作原理。
import pandas as pd import numpy as np # Create a Series with random numbers s = pd.Series(np.random.randn(10)) print("Series:") print(s) # Using basic methods print("First 5 elements of the Series:\n", s.head()) print("\nLast 3 elements of the Series:\n", s.tail(3)) print("\nDescriptive statistics of the Series:\n", s.describe())
其输出如下:
Series: 0 -0.295898 1 -0.786081 2 -1.189834 3 -0.410830 4 -0.997866 5 0.084868 6 0.736541 7 0.133949 8 1.023674 9 0.669520 dtype: float64 First 5 elements of the Series: 0 -0.295898 1 -0.786081 2 -1.189834 3 -0.410830 4 -0.997866 dtype: float64 Last 3 elements of the Series: 7 0.133949 8 1.023674 9 0.669520 dtype: float64 Descriptive statistics of the Series: count 10.000000 mean -0.103196 std 0.763254 min -1.189834 25% -0.692268 50% -0.105515 75% 0.535627 max 1.023674 dtype: float64
示例
现在看看下面的示例,并了解基本方法在 DataFrame 对象上的工作原理。
import pandas as pd import numpy as np #Create a Dictionary of series data = {'Name':pd.Series(['Tom','James','Ricky','Vin','Steve','Smith','Jack']), 'Age':pd.Series([25,26,25,23,30,29,23]), 'Rating':pd.Series([4.23,3.24,3.98,2.56,3.20,4.6,3.8])} #Create a DataFrame df = pd.DataFrame(data) print("Our data frame is:\n") print(df) # Using basic methods print("\nFirst 5 rows of the DataFrame:\n", df.head()) print("\nLast 3 rows of the DataFrame:\n", df.tail(3)) print("\nInfo of the DataFrame:") df.info() print("\nDescriptive statistics of the DataFrame:\n", df.describe())
执行上述代码后,您将获得以下输出:
Our data frame is: Name Age Rating 0 Tom 25 4.23 1 James 26 3.24 2 Ricky 25 3.98 3 Vin 23 2.56 4 Steve 30 3.20 5 Smith 29 4.60 6 Jack 23 3.80 First 5 rows of the DataFrame: Name Age Rating 0 Tom 25 4.23 1 James 26 3.24 2 Ricky 25 3.98 3 Vin 23 2.56 4 Steve 30 3.20 Last 3 rows of the DataFrame: Name Age Rating 4 Steve 30 3.2 5 Smith 29 4.6 6 Jack 23 3.8 Info of the DataFrame: <class 'pandas.core.frame.DataFrame'> RangeIndex: 7 entries, 0 to 6 Data columns (total 3 columns): # Column Non-Null Count Dtype --- ------ -------------- ----- 0 Name 7 non-null object 1 Age 7 non-null int64 2 Rating 7 non-null float64 dtypes: float64(1), int64(1), object(1) memory usage: 296.0+ bytes Descriptive statistics of the DataFrame: Age Rating count 7.000000 7.000000 mean 25.857143 3.658571 std 2.734262 0.698628 min 23.000000 2.560000 25% 24.000000 3.220000 50% 25.000000 3.800000 75% 27.500000 4.105000 max 30.000000 4.600000