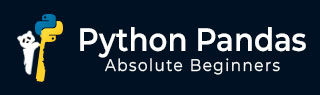
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - Panel
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片Series对象
- Python Pandas - Series对象的属性
- Python Pandas - Series对象的算术运算
- Python Pandas - 将Series转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问DataFrame
- Python Pandas - 切片DataFrame对象
- Python Pandas - 修改DataFrame
- Python Pandas - 从DataFrame中删除行
- Python Pandas - DataFrame的算术运算
- Python Pandas - I/O工具
- Python Pandas - I/O工具
- Python Pandas - 使用CSV格式
- Python Pandas - 读取和写入JSON文件
- Python Pandas - 从Excel文件读取数据
- Python Pandas - 将数据写入Excel文件
- Python Pandas - 使用HTML数据
- Python Pandas - 剪贴板
- Python Pandas - 使用HDF5格式
- Python Pandas - 与SQL的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 级联
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 使用文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引的高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和分类
- Python Pandas - 比较分类数据
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 附加概念
- Python Pandas - 警告和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 读取和写入JSON文件
Pandas库提供了强大的函数,用于以各种格式读取和写入数据。其中一种格式是JSON(JavaScript对象表示法),它是一种轻量级的数据交换格式,易于人工读取和写入。JSON广泛用于在服务器和Web应用程序之间传输数据。
JSON文件以结构化格式存储数据,类似于Python中的字典或列表。JSON文件扩展名为.json。下面您可以看到JSON文件中数据的样式:
[ { "Name": "Braund", "Gender": "Male", "Age": 30 }, { "Name": "Cumings", "Gender": "Female", "Age": 25 }, { "Name": "Heikkinen", "Gender": "female", "Age": 35 } ]
在本教程中,我们将学习如何使用Pandas处理JSON文件,包括读取和写入JSON文件以及一些常见的配置。
读取JSON文件
pandas.read_json()函数用于将JSON数据读取到Pandas DataFrame中。此函数可以接受文件路径、URL或JSON字符串作为输入。
示例
以下示例演示了如何使用pandas.read_json()函数读取JSON数据。这里我们使用StringIO将JSON字符串加载到类似文件的对象中。
import pandas as pd from io import StringIO # Create a string representing JSON data data = """[ {"Name": "Braund", "Gender": "Male", "Age": 30}, {"Name": "Cumings", "Gender": "Female", "Age": 25}, {"Name": "Heikkinen", "Gender": "Female", "Age": 35} ]""" # Use StringIO to convert the JSON formatted string data into a file-like object obj = StringIO(data) # Read JSON into a Pandas DataFrame df = pd.read_json(obj) print(df)
以下是上述代码的输出:
Name | Gender | Age | |
---|---|---|---|
0 | Braund | Male | 30 |
1 | Cumings | Female | 25 |
2 | Heikkinen | Female | 35 |
写入JSON文件
Pandas提供.to_json()函数,可以使用Pandas DataFrame或Series对象中的数据来写入或创建JSON文件。此函数用于将Pandas数据结构对象转换为JSON字符串,并允许您使用多个参数自定义JSON输出的格式。
示例:写入JSON文件的基本示例
这是一个演示如何将Pandas DataFrame写入JSON文件的示例。
import pandas as pd # Create a DataFrame from the above dictionary df = pd.DataFrame({"Name":["Braund", "Cumings", "Heikkinen"], "Gender": ["Male", "Female", "Female"], "Age": [30, 25, 25]}) print("Original DataFrame:\n", df) # Write DataFrame to a JSON file df.to_json("output_written_json_file.json", orient='records', lines=True) print("The output JSON file has been written successfully.")
以下是上述代码的输出:
Original DataFrame:
Name | Gender | Age | |
---|---|---|---|
0 | Braund | Male | 30 |
1 | Cumings | Female | 25 |
2 | Heikkinen | Female | 35 |
执行上述代码后,您可以在工作目录中找到名为output_written_json_file.json的已创建JSON文件。
示例:使用split方向写入JSON文件
以下示例使用split方向将简单的DataFrame对象写入JSON。
import pandas as pd from json import loads, dumps # Create a DataFrame df = pd.DataFrame( [["x", "y"], ["z", "w"]], index=["row_1", "row_2"], columns=["col_1", "col_2"], ) # Convert DataFrame to JSON with 'split' orientation result = df.to_json(orient="split") parsed = loads(result) # Display the JSON output print("JSON Output (split orientation):") print(dumps(parsed, indent=4))
以下是上述代码的输出:
JSON Output (split orientation): { "columns": [ "col_1", "col_2" ], "index": [ "row_1", "row_2" ], "data": [ [ "x", "y" ], [ "z", "w" ] ] }
广告