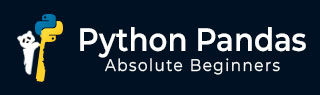
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 处理 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引进行高级重索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视
- Python Pandas - 堆叠和取消堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 分类数据
- Python Pandas - 分类数据
- Python Pandas - 分类数据的排序和分类
- Python Pandas - 分类数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复数据
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 迭代
在数据操作中,遍历 pandas 对象是一项基本任务,遍历的行为取决于您正在处理的对象类型。本教程解释了 pandas 中的迭代是如何工作的,特别关注 Series 和 DataFrame 对象。
pandas 中的迭代行为在 Series 和 DataFrame 对象之间有所不同 -
Series:遍历 Series 对象会直接产生值,使其类似于数组结构。
DataFrame:遍历 DataFrame 遵循类似字典的约定,其中迭代会产生列标签(即键)。
遍历 DataFrame 中的行
要遍历 DataFrame 的行,我们可以使用以下方法 -
items():遍历 (键,值) 对
iterrows():将行迭代为 (索引,系列) 对
itertuples():将行迭代为命名元组
遍历列对
items() 方法允许您将每一列迭代为键值对,其中标签作为键,列值作为 Series 对象。此方法与 DataFrame 的类似字典的接口一致。
示例
以下示例使用 items() 方法迭代 DataFrame 行。在此示例中,每列都作为 Series 中的键值对单独迭代。
import pandas as pd import numpy as np df = pd.DataFrame(np.random.randn(4,3),columns=['col1','col2','col3']) print("Original DataFrame:\n", df) # Iterate Through DataFrame rows print("Iterated Output:") for key,value in df.items(): print(key,value)
其输出如下 -
Original DataFrame: col1 col2 col3 0 0.422561 0.094621 -0.214307 1 0.430612 -0.334812 -0.010867 2 0.350962 -0.145470 0.988463 3 1.466426 -1.258297 -0.824569 Iterated Output: col1 0 0.422561 1 0.430612 2 0.350962 3 1.466426 Name: col1, dtype: float64 col2 0 0.094621 1 -0.334812 2 -0.145470 3 -1.258297 Name: col2, dtype: float64 col3 0 -0.214307 1 -0.010867 2 0.988463 3 -0.824569 Name: col3, dtype: float64
观察,每列都单独迭代,其中键是列名,值是相应的 Series 对象。
将 DataFrame 迭代为 Series 对
iterrows() 方法返回一个迭代器,该迭代器会产生索引和行对,其中每一行都表示为 Series 对象,其中包含每一行中的数据。
示例
以下示例使用 iterrows() 方法迭代 DataFrame 行。
import pandas as pd import numpy as np df = pd.DataFrame(np.random.randn(4,3),columns = ['col1','col2','col3']) print("Original DataFrame:\n", df) # Iterate Through DataFrame rows print("Iterated Output:") for row_index,row in df.iterrows(): print(row_index,row)
其输出如下 -
Original DataFrame: col1 col2 col3 0 0.468160 -0.634193 -0.603612 1 1.231840 0.090565 -0.449989 2 -1.645371 0.032578 -0.165950 3 1.956370 -0.261995 2.168167 Iterated Output: 0 col1 0.468160 col2 -0.634193 col3 -0.603612 Name: 0, dtype: float64 1 col1 1.231840 col2 0.090565 col3 -0.449989 Name: 1, dtype: float64 2 col1 -1.645371 col2 0.032578 col3 -0.165950 Name: 2, dtype: float64 3 col1 1.956370 col2 -0.261995 col3 2.168167 Name: 3, dtype: float64
注意:因为 iterrows() 遍历行,所以它不会在行之间保留数据类型。0、1、2 是行索引,col1、col2、col3 是列索引。
将 DataFrame 迭代为命名元组
itertuples() 方法将返回一个迭代器,为 DataFrame 中的每一行生成一个命名元组。元组的第一个元素将是行的对应索引值,其余值为行值。此方法通常比 iterrows() 快,并保留行元素的数据类型。
示例
以下示例使用 itertuples() 方法将 DataFrame 行循环为命名元组
import pandas as pd import numpy as np df = pd.DataFrame(np.random.randn(4,3),columns = ['col1','col2','col3']) print("Original DataFrame:\n", df) # Iterate Through DataFrame rows print("Iterated Output:") for row in df.itertuples(): print(row)
其输出如下 -
Original DataFrame: col1 col2 col3 0 0.501238 -0.353269 -0.058190 1 -0.426044 -0.012733 -0.532594 2 -0.704042 2.201186 -1.960429 3 0.514151 -0.844160 0.508056 Iterated Output: Pandas(Index=0, col1=0.5012381423628608, col2=-0.3532690739340918, col3=-0.058189913290578134) Pandas(Index=1, col1=-0.42604395958954777, col2=-0.012733326002509393, col3=-0.5325942971498149) Pandas(Index=2, col1=-0.7040424042099052, col2=2.201186165472291, col3=-1.9604285032438307) Pandas(Index=3, col1=0.5141508750506754, col2=-0.8441600001815068, col3=0.5080555294913854)
遍历 DataFrame 列
当您遍历 DataFrame 时,它只会返回列名。
示例
让我们考虑以下示例来了解 DataFrame 列的遍历。
import pandas as pd import numpy as np N = 5 df = pd.DataFrame({ 'A': pd.date_range(start='2016-01-01', periods=N, freq='D'), 'x': np.linspace(0, stop=N-1, num=N), 'y': np.random.rand(N), 'C': np.random.choice(['Low', 'Medium', 'High'], N).tolist(), 'D': np.random.normal(100, 10, size=N).tolist() }) print("Original DataFrame:\n", df) # Iterate Through DataFrame Columns print("Output:") for col in df: print(col)
其输出如下 -
Original DataFrame: A x y C D 0 2016-01-01 0.0 0.990949 Low 114.143838 1 2016-01-02 1.0 0.314517 High 95.559640 2 2016-01-03 2.0 0.180237 Low 121.134817 3 2016-01-04 3.0 0.170095 Low 95.643132 4 2016-01-05 4.0 0.920718 Low 96.379692 Output: A x y C D
示例
在遍历 DataFrame 时,您不应该修改任何对象。迭代旨在用于读取,迭代器返回原始对象的副本(视图),这意味着更改不会反映在原始对象上。以下示例演示了上述语句。
import pandas as pd import numpy as np df = pd.DataFrame(np.random.randn(4,3),columns = ['col1','col2','col3']) for index, row in df.iterrows(): row['a'] = 10 print(df)
其输出如下 -
col1 col2 col3 0 -1.739815 0.735595 -0.295589 1 0.635485 0.106803 1.527922 2 -0.939064 0.547095 0.038585 3 -1.016509 -0.116580 -0.523158
如您所见,DataFrame 中没有反映任何更改,因为迭代仅提供数据的视图。