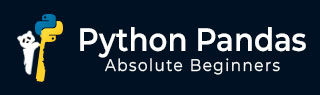
- Python Pandas 教程
- Python Pandas - 首页
- Python Pandas - 简介
- Python Pandas - 环境设置
- Python Pandas - 基础
- Python Pandas - 数据结构介绍
- Python Pandas - 索引对象
- Python Pandas - 面板
- Python Pandas - 基本功能
- Python Pandas - 索引和数据选择
- Python Pandas - Series
- Python Pandas - Series
- Python Pandas - 切片 Series 对象
- Python Pandas - Series 对象的属性
- Python Pandas - Series 对象的算术运算
- Python Pandas - 将 Series 转换为其他对象
- Python Pandas - DataFrame
- Python Pandas - DataFrame
- Python Pandas - 访问 DataFrame
- Python Pandas - 切片 DataFrame 对象
- Python Pandas - 修改 DataFrame
- Python Pandas - 从 DataFrame 中删除行
- Python Pandas - DataFrame 的算术运算
- Python Pandas - I/O 工具
- Python Pandas - I/O 工具
- Python Pandas - 使用 CSV 格式
- Python Pandas - 读取和写入 JSON 文件
- Python Pandas - 从 Excel 文件读取数据
- Python Pandas - 将数据写入 Excel 文件
- Python Pandas - 使用 HTML 数据
- Python Pandas - 剪贴板
- Python Pandas - 使用 HDF5 格式
- Python Pandas - 与 SQL 的比较
- Python Pandas - 数据处理
- Python Pandas - 排序
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 连接
- Python Pandas - 统计函数
- Python Pandas - 描述性统计
- Python Pandas - 处理文本数据
- Python Pandas - 函数应用
- Python Pandas - 选项和自定义
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 合并/连接
- Python Pandas - 多级索引
- Python Pandas - 多级索引的基础
- Python Pandas - 使用多级索引进行索引
- Python Pandas - 使用多级索引的高级重新索引
- Python Pandas - 重命名多级索引标签
- Python Pandas - 对多级索引进行排序
- Python Pandas - 二元运算
- Python Pandas - 二元比较运算
- Python Pandas - 布尔索引
- Python Pandas - 布尔掩码
- Python Pandas - 数据重塑和透视
- Python Pandas - 透视表
- Python Pandas - 堆叠和解堆叠
- Python Pandas - 熔化
- Python Pandas - 计算虚拟变量
- Python Pandas - 类别数据
- Python Pandas - 类别数据
- Python Pandas - 类别数据的排序和分类
- Python Pandas - 类别数据的比较
- Python Pandas - 处理缺失数据
- Python Pandas - 缺失数据
- Python Pandas - 填充缺失数据
- Python Pandas - 缺失值的插值
- Python Pandas - 删除缺失数据
- Python Pandas - 使用缺失数据进行计算
- Python Pandas - 处理重复项
- Python Pandas - 重复数据
- Python Pandas - 计数和检索唯一元素
- Python Pandas - 重复标签
- Python Pandas - 分组和聚合
- Python Pandas - GroupBy
- Python Pandas - 时间序列数据
- Python Pandas - 日期功能
- Python Pandas - 时间差
- Python Pandas - 稀疏数据结构
- Python Pandas - 稀疏数据
- Python Pandas - 可视化
- Python Pandas - 可视化
- Python Pandas - 其他概念
- Python Pandas - 注意事项和陷阱
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用资源
- Python Pandas - 讨论
Python Pandas - 填充缺失数据
填充缺失数据是一个用有意义的替代方案替换缺失 (NaN) 值的过程。无论您是想用常量值替换缺失值,还是向前或向后传播值,Pandas 都内置了函数来实现这一点。
在本教程中,我们将学习在 Pandas 中填充缺失数据的不同方法,包括:
用标量替换缺失值。
向前和向后填充。
使用指定的限制进行填充。
使用 replace() 方法替换数据。
使用正则表达式替换值。
用标量值填充缺失数据
Pandas 中的 fillna() 方法用于用标量值(例如任何特定数字)填充缺失值 (NA 或 NaN)。
示例
以下演示了如何使用 fillna() 方法用标量值(“NaN” 为 “5”)填充缺失值 NaN。
import pandas as pd import numpy as np # Create DataFrame with missing values data = {"Col1": [3, np.nan, np.nan, 2], "Col2": [1.0, pd.NA, pd.NA, 2.0]} df = pd.DataFrame(data) # Display the original DataFrame with missing values print("Original DataFrame:\n",df) # Fill missing values with 5 df_filled = df.fillna(5) print("\nResultant DataFrame after NaN replaced with '5':\n", df_filled)
其输出如下:
Original DataFrame:
Col1 | Col2 | |
---|---|---|
0 | 3.0 | 1.0 |
1 | NaN | <NA> |
2 | NaN | <NA> |
3 | 2.0 | 2.0 |
Col1 | Col2 | |
---|---|---|
0 | 3.0 | 1.0 |
1 | 5.0 | 5.0 |
2 | 5.0 | 5.0 |
3 | 2.0 | 2.0 |
向前或向后填充缺失值
您还可以分别使用 ffill() 和 bfill() 方法传播最后一个有效观测值以向前或向后填充间隙。
序号 | 方法和操作 |
---|---|
1 |
ffill() 此方法用前一个有效值填充缺失值。 |
2 |
bfill() 此方法用下一个有效值填充缺失值。 |
示例:向前填充
此示例使用向前填充 ffill() 方法替换缺失值。
import pandas as pd import numpy as np # Create DataFrame with missing values df = pd.DataFrame([[9, -3, -2], [-5, 1, 8], [6, 4, -8]], index=['a', 'c', 'd'], columns=['one', 'two', 'three']) df = df.reindex(['a', 'b', 'c', 'd', 'e']) # Display the original DataFrame with missing values print("Original DataFrame:\n",df) # Forward Fill the missing values result = df.ffill() print("\nResultant DataFrame after Forward fill:\n", result)
其输出如下:
Original DataFrame:
one | two | three | |
---|---|---|---|
a | 9.0 | -3.0 | -2.0 |
b | NaN | NaN | NaN |
c | -5.0 | 1.0 | 8.0 |
d | 6.0 | 4.0 | -8.0 |
e | NaN | NaN | NaN |
one | two | three | |
---|---|---|---|
a | 9.0 | -3.0 | -2.0 |
b | 9.0 | -3.0 | -2.0 |
c | -5.0 | 1.0 | 8.0 |
d | 6.0 | 4.0 | -8.0 |
e | 6.0 | 4.0 | -8.0 |
示例:向后填充
此示例使用向后填充 bfill() 方法替换缺失值。
import pandas as pd import numpy as np # Create DataFrame with missing values df = pd.DataFrame([[9, -3, -2], [-5, 1, 8], [6, 4, -8]], index=['a', 'c', 'd'], columns=['one', 'two', 'three']) df = df.reindex(['a', 'b', 'c', 'd', 'e']) # Display the original DataFrame with missing values print("Original DataFrame:\n",df) # Backward Fill the missing values result = df.bfill() print("\nResultant DataFrame after Backward fill:\n", result)
其输出如下:
Original DataFrame:
one | two | three | |
---|---|---|---|
a | 9.0 | -3.0 | -2.0 |
b | NaN | NaN | NaN |
c | -5.0 | 1.0 | 8.0 |
d | 6.0 | 4.0 | -8.0 |
e | NaN | NaN | NaN |
one | two | three | |
---|---|---|---|
a | 9.0 | -3.0 | -2.0 |
b | -5.0 | 1.0 | 8.0 |
c | -5.0 | 1.0 | 8.0 |
d | 6.0 | 4.0 | -8.0 |
e | NaN | NaN | NaN |
限制填充次数
您还可以通过指定 limit 参数来控制填充多少个连续缺失值的限制。
示例
以下示例演示了如何使用 limit 参数使用 ffill() 方法设置填充缺失值的限制。
import pandas as pd import numpy as np # Create DataFrame with missing values df = pd.DataFrame([[9, -3, -2], [-5, 1, 8], [6, 4, -8]], index=['a', 'c', 'd'], columns=['one', 'two', 'three']) df = df.reindex(['a', 'b', 'd', 'e', 'f']) # Display the original DataFrame with missing values print("Original DataFrame:\n",df) # Forward Fill the missing values with limit result = df.ffill(limit=1) print("\nResultant DataFrame after Forward fill:\n", result)
以下是上述代码的输出:
Original DataFrame:
one | two | three | |
---|---|---|---|
a | 9.0 | -3.0 | -2.0 |
b | NaN | NaN | NaN |
d | 6.0 | 4.0 | -8.0 |
e | NaN | NaN | NaN |
f | NaN | NaN | NaN |
one | two | three | |
---|---|---|---|
a | 9.0 | -3.0 | -2.0 |
b | 9.0 | -3.0 | -2.0 |
d | 6.0 | 4.0 | -8.0 |
e | 6.0 | 4.0 | -8.0 |
f | NaN | NaN | NaN |
使用 replace() 方法替换数据
很多时候,我们必须用一些特定值替换通用值。我们可以通过应用 replace() 方法来实现。
用标量值替换 NA 等效于 fillna() 函数的行为。
示例
以下是使用 replace() 方法替换通用值的示例。
import pandas as pd import numpy as np # Create DataFrame df = pd.DataFrame({'one':[10,20,30,40,50,2000], 'two':[1000,0,30,40,50,60]}) # Replace the generic values print(df.replace({1000:10,2000:60}))
其输出如下:
one | two | |
---|---|---|
0 | 10 | 10 |
1 | 20 | 0 |
2 | 30 | 30 |
3 | 40 | 40 |
4 | 50 | 50 |
5 | 60 | 60 |
使用正则表达式替换缺失数据
您还可以使用正则表达式模式用 replace() 方法替换数据中的缺失值。
示例
以下是使用正则表达式用 replace() 方法替换特定数据的示例。
import pandas as pd import numpy as np # Create DataFrame with missing values df = pd.DataFrame({"a": list(range(4)), "b": list("ab.."), "c": ["a", "b", np.nan, "d"]}) # Display the original DataFrame with missing values print("Original DataFrame:\n",df) # Replace the missing values with regular exp result = df.replace(r"\.", 10, regex=True) print("\nResultant DataFrame after filling the missing values using regex:\n", result)
其输出如下:
Original DataFrame:
a | b | c | |
---|---|---|---|
0 | 0 | a | a |
1 | 1 | b | b |
2 | 2 | . | NaN |
3 | 3 | . | d |
a | b | c | |
---|---|---|---|
0 | 0 | a | a |
1 | 1 | b | b |
2 | 2 | 10 | NaN |
3 | 3 | 10 | d |
广告