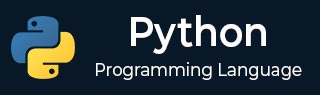
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 文件重命名和删除
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 连接线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部结构
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python Requests put() 方法
Python Requests 的`put()`方法用于向指定的URL发送PUT请求。此方法用于使用提供的数据更新或替换给定URL处的资源。
`put()`方法类似于`requests.post()`,但专门用于替换现有资源,而不是创建新资源。它接受URL、数据、报头、文件、身份验证、超时等参数来定制请求。
此方法返回一个响应对象,其中包含有关请求状态、报头和内容的信息,可以根据应用程序的需求进一步处理。
语法
以下是Python Requests `put()`方法的语法和参数:
requests.get(url, params=None, **kwargs)
参数
以下是Python Requests `put()`方法的参数:
- url: url是要发送PUT请求的资源的URL。
- data(可选): 这是要与PUT请求一起发送的数据。
- json(可选): 要在主体中发送的JSON数据。
- kwargs(可选): 可以传递的可选参数,包括headers、cookies、auth、timeout等。
返回值
此方法返回一个Response对象。
示例1
以下是使用python requests `put()`方法的基本PUT请求的基本示例:
import requests # Define the URL url = 'https://httpbin.org/put' # Define the data to be sent in the request body data = {'key1': 'value1', 'key2': 'value2'} # Send the PUT request with the data response = requests.put(url, json=data) # Print the response status code print('Status Code:', response.status_code) # Print the response content print('Response Content:', response.text)
输出
Status Code: 200 Response Content: { "args": {}, ---------------- ---------------- ---------------- }, "origin": "110.226.149.205", "url": "https://httpbin.org/put" }
示例2
当使用Python中的requests模块发送PUT请求时,我们可以处理请求过程中可能发生的错误。以下示例处理put请求中的错误:
import requests try: response = requests.put('https://api.example.com/nonexistent', timeout=5) response.raise_for_status() # Raises an HTTPError if the status code is 4xx, 5xx except requests.exceptions.HTTPError as err: print(f'HTTP error occurred: {err}') except requests.exceptions.RequestException as err: print(f'Request error occurred: {err}')
输出
Request error occurred: HTTPSConnectionPool(host='api.example.com', port=443): Max retries exceeded with url: /nonexistent (Caused by NameResolutionError("<urllib3.connection.HTTPSConnection object at 0x000001BC03605890>: Failed to resolve 'api.example.com' ([Errno 11001] getaddrinfo failed)"))
示例3
这是一个使用python requests `put()`方法设置超时的PUT请求示例:
import requests # Define the URL url = 'https://httpbin.org/put' # Define the data to be sent in the request body data = {'key1': 'value1', 'key2': 'value2'} # Set the timeout for the request (in seconds) timeout = 5 try: # Send the PUT request with the data and timeout response = requests.put(url, json=data, timeout=timeout) # Print the response status code print('Status Code:', response.status_code) # Print the response content print('Response Content:', response.text) except requests.Timeout: # Handle timeout error print('Timeout Error: Request timed out.') except requests.RequestException as e: # Handle other request exceptions print('Request Exception:', e)
输出
Status Code: 200 Response Content: { "args": {}, ------------------- ------------------- ------------------- }, "origin": "110.226.149.205", "url": "https://httpbin.org/put" }
python_modules.htm
广告