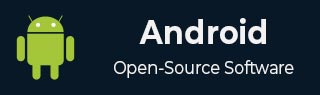
- Android 基础
- Android - 首页
- Android - 概览
- Android - 环境设置
- Android - 架构
- Android - 应用组件
- Android - Hello World 示例
- Android - 资源
- Android - 活动
- Android - 服务
- Android - 广播接收器
- Android - 内容提供程序
- Android - 碎片
- Android - 意图/过滤器
- Android - 用户界面
- Android - UI 布局
- Android - UI 控件
- Android - 事件处理
- Android - 样式和主题
- Android - 自定义组件
- Android 高级概念
- Android - 拖放
- Android - 通知
- 基于位置的服务
- Android - 发送电子邮件
- Android - 发送短信
- Android - 电话呼叫
- 发布 Android 应用
- Android 有用示例
- Android - 警报对话框
- Android - 动画
- Android - 音频捕捉
- Android - 音频管理器
- Android - 自动完成
- Android - 最佳实践
- Android - 蓝牙
- Android - 相机
- Android - 剪贴板
- Android - 自定义字体
- Android - 数据备份
- Android - 开发者工具
- Android - 模拟器
- Android - Facebook 集成
- Android - 手势
- Android - Google 地图
- Android - 图像效果
- Android - ImageSwitcher
- Android - 内部存储
- Android - JetPlayer
- Android - JSON 解析器
- Android - Linkedin 集成
- Android - 加载微调器
- Android - 本地化
- Android - 登录屏幕
- Android - MediaPlayer
- Android - 多点触控
- Android - 导航
- Android - 网络连接
- Android - NFC 指南
- Android - PHP/MySQL
- Android - 进度圆圈
- Android - 进度条
- Android - 推送通知
- Android - RenderScript
- Android - RSS 阅读器
- Android - 屏幕录制
- Android - SDK 管理器
- Android - 传感器
- Android - 会话管理
- Android - 共享首选项
- Android - SIP 协议
- Android - 拼写检查器
- Android - SQLite 数据库
- Android - 支持库
- Android - 测试
- Android - 文本转语音
- Android - TextureView
- Android - Twitter 集成
- Android - UI 设计
- Android - UI 模式
- Android - UI 测试
- Android - WebView 布局
- Android - Wi-Fi
- Android - 小部件
- Android - XML 解析器
- Android 有用资源
- Android - 问答
- Android - 有用资源
- Android - 讨论
Android - 单一碎片
单帧碎片
单帧碎片专为小型屏幕设备(如手持设备(手机))设计,并且应高于 Android 3.0 版本。
示例
此示例将向您解释如何创建自己的碎片。在这里,我们将创建两个碎片,其中一个将在设备处于横向模式时使用,另一个碎片将在纵向模式下使用。因此,让我们按照以下步骤操作,类似于我们在创建Hello World 示例时所遵循的步骤 -
步骤 | 描述 |
---|---|
1 | 您将使用 Android Studio IDE 创建一个 Android 应用程序,并将其命名为MyFragments,位于包com.example.myfragments下,并使用空白 Activity。 |
2 | 修改主活动文件MainActivity.java,如下面的代码所示。在这里,我们将检查设备的方向,并相应地在不同的碎片之间切换。 |
3 | 在包com.example.myfragments下创建两个 Java 文件PM_Fragment.java和LM_Fragement.java,以定义您的碎片和关联方法。 |
4 | 创建布局文件res/layout/lm_fragment.xml和res/layout/pm_fragment.xml,并为这两个碎片定义您的布局。 |
5 | 修改res/layout/activity_main.xml文件的默认内容以包含这两个碎片。 |
6 | 在res/values/strings.xml文件中定义所需的常量 |
7 | 运行应用程序以启动 Android 模拟器并验证对应用程序所做的更改的结果。 |
以下是修改后的主活动文件MainActivity.java的内容 -
package com.example.myfragments; import android.app.Activity; import android.app.FragmentManager; import android.app.FragmentTransaction; import android.content.res.Configuration; import android.os.Bundle; public class MainActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); Configuration config = getResources().getConfiguration(); FragmentManager fragmentManager = getFragmentManager(); FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction(); /** * Check the device orientation and act accordingly */ if (config.orientation == Configuration.ORIENTATION_LANDSCAPE) { /** * Landscape mode of the device */ LM_Fragement ls_fragment = new LM_Fragement(); fragmentTransaction.replace(android.R.id.content, ls_fragment); }else{ /** * Portrait mode of the device */ PM_Fragement pm_fragment = new PM_Fragement(); fragmentTransaction.replace(android.R.id.content, pm_fragment); } fragmentTransaction.commit(); } }
创建两个碎片文件LM_Fragement.java和PM_Fragment.java
以下是LM_Fragement.java文件的内容 -
package com.example.myfragments; import android.app.Fragment; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * Created by TutorialsPoint7 on 8/23/2016. */ public class LM_Fragement extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { /** * Inflate the layout for this fragment */ return inflater.inflate(R.layout.lm_fragment, container, false); } }
以下是PM_Fragement.java文件的内容 -
package com.example.myfragments; import android.app.Fragment; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * Created by TutorialsPoint7 on 8/23/2016. */ public class PM_Fragement extends Fragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { /** * Inflate the layout for this fragment */ return inflater.inflate(R.layout.pm_fragment, container, false); } }
在res/layout目录下创建两个布局文件lm_fragement.xml和pm_fragment.xml。
以下是lm_fragement.xml文件的内容 -
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#7bae16"> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/landscape_message" android:textColor="#000000" android:textSize="20px" /> <!-- More GUI components go here --> </LinearLayout>
以下是pm_fragment.xml文件的内容 -
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#666666"> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/portrait_message" android:textColor="#000000" android:textSize="20px" /> <!-- More GUI components go here --> </LinearLayout>
以下是包含碎片的res/layout/activity_main.xml文件的内容 -
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="horizontal"> <fragment android:name="com.example.fragments" android:id="@+id/lm_fragment" android:layout_weight="1" android:layout_width="0dp" android:layout_height="match_parent" /> <fragment android:name="com.example.fragments" android:id="@+id/pm_fragment" android:layout_weight="2" android:layout_width="0dp" android:layout_height="match_parent" /> </LinearLayout>
确保您具有res/values/strings.xml文件的以下内容 -
<?xml version="1.0" encoding="utf-8"?> <resources> <string name="app_name">My Application</string> <string name="landscape_message">This is Landscape mode fragment</string> <string name="portrait_message">This is Portrait mode fragment></string> </resources>
让我们尝试运行我们刚刚创建的修改后的MyFragments应用程序。我假设您在进行环境设置时已创建了AVD。要从 Android Studio 运行该应用程序,请打开项目中的一个活动文件,然后单击工具栏中的“运行”图标。Android Studio 将应用程序安装到您的 AVD 上并启动它,如果您的设置和应用程序一切正常,它将显示模拟器窗口,您可以在其中单击“菜单”按钮以查看以下窗口。请耐心等待,因为这可能需要一些时间,具体取决于您的计算机速度 -
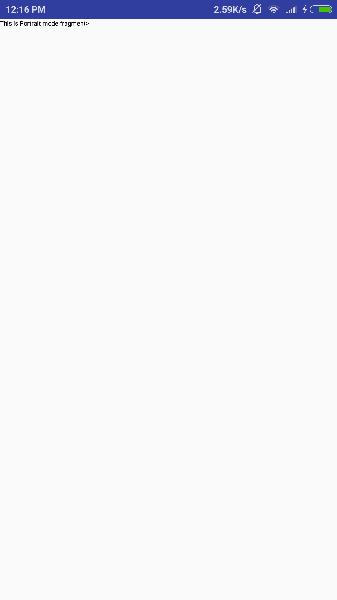
要更改模拟器屏幕的模式,让我们执行以下操作 -
在 Mac 上按fn+control+F11将横向更改为纵向,反之亦然。
在 Windows 上按ctrl+F11。
在 Linux 上按ctrl+F11。
更改模式后,您将能够看到为横向模式实现的 GUI,如下所示 -
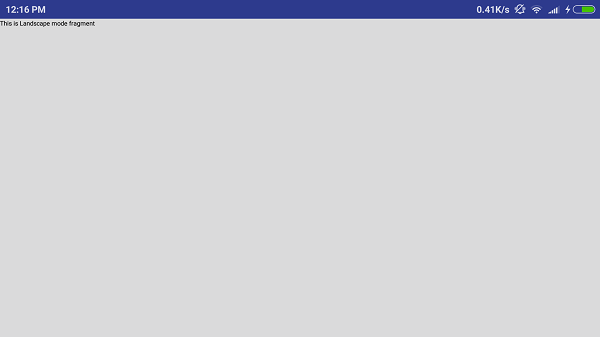
这样,您可以使用相同的活动,但通过不同的碎片使用不同的 GUI。您可以根据需要为不同的 GUI 使用不同类型的 GUI 组件。