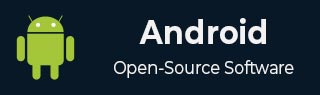
- Android 基础
- Android - 首页
- Android - 概述
- Android - 环境搭建
- Android - 架构
- Android - 应用组件
- Android - Hello World 示例
- Android - 资源
- Android - 活动 (Activities)
- Android - 服务 (Services)
- Android - 广播接收器 (Broadcast Receivers)
- Android - 内容提供器 (Content Providers)
- Android - 碎片 (Fragments)
- Android - 意图/过滤器 (Intents/Filters)
- Android - 用户界面
- Android - UI 布局
- Android - UI 控件
- Android - 事件处理
- Android - 样式和主题
- Android - 自定义组件
- Android 高级概念
- Android - 拖放
- Android - 通知
- 基于位置的服务
- Android - 发送邮件
- Android - 发送短信
- Android - 打电话
- 发布 Android 应用
- Android 实用示例
- Android - 警报对话框
- Android - 动画
- Android - 音频采集
- Android - AudioManager
- Android - 自动完成
- Android - 最佳实践
- Android - 蓝牙
- Android - 相机
- Android - 剪贴板
- Android - 自定义字体
- Android - 数据备份
- Android - 开发者工具
- Android - 模拟器
- Android - Facebook 集成
- Android - 手势
- Android - Google 地图
- Android - 图片特效
- Android - ImageSwitcher
- Android - 内部存储
- Android - JetPlayer
- Android - JSON 解析器
- Android - Linkedin 集成
- Android - 加载动画 (Loading Spinner)
- Android - 本地化
- Android - 登录界面
- Android - MediaPlayer
- Android - 多点触控
- Android - 导航
- Android - 网络连接
- Android - NFC 指南
- Android - PHP/MySQL
- Android - 进度圆圈
- Android - ProgressBar
- Android - 推送通知
- Android - RenderScript
- Android - RSS 阅读器
- Android - 屏幕录制
- Android - SDK 管理器
- Android - 传感器
- Android - 会话管理
- Android - 共享首选项
- Android - SIP 协议
- Android - 拼写检查器
- Android - SQLite 数据库
- Android - 支持库
- Android - 测试
- Android - 文字转语音
- Android - TextureView
- Android - Twitter 集成
- Android - UI 设计
- Android - UI 模式
- Android - UI 测试
- Android - WebView 布局
- Android - Wi-Fi
- Android - 小部件 (Widgets)
- Android - XML 解析器
- Android 实用资源
- Android - 问答
- Android - 实用资源
- Android - 讨论
Android - 文字转语音
Android 允许您将文本转换为语音。您不仅可以转换文本,还可以用多种不同的语言朗读文本。
Android 为此提供了 **TextToSpeech** 类。要使用此类,您需要实例化此类的对象并指定 **initListener**。其语法如下:
private EditText write; ttobj=new TextToSpeech(getApplicationContext(), new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { } });
在此监听器中,您需要指定 TextToSpeech 对象的属性,例如其语言、音调等。语言可以通过调用 **setLanguage()** 方法设置。其语法如下:
ttobj.setLanguage(Locale.UK);
setLanguage 方法采用 Locale 对象作为参数。以下是部分可用语言环境:
序号 | 语言环境 |
---|---|
1 | 美国 (US) |
2 | 加拿大法语 (CANADA_FRENCH) |
3 | 德国 (GERMANY) |
4 | 意大利 (ITALY) |
5 | 日本 (JAPAN) |
6 | 中国 (CHINA) |
设置语言后,您可以调用该类的 **speak** 方法来朗读文本。其语法如下:
ttobj.speak(toSpeak, TextToSpeech.QUEUE_FLUSH, null);
除了 speak 方法外,TextToSpeech 类中还提供了一些其他方法。列举如下:
序号 | 方法及描述 |
---|---|
1 |
addSpeech(String text, String filename) 此方法在文本字符串和声音文件之间添加映射。 |
2 |
getLanguage() 此方法返回一个描述语言的 Locale 实例。 |
3 |
isSpeaking() 此方法检查 TextToSpeech 引擎是否正在忙于朗读。 |
4 |
setPitch(float pitch) 此方法设置 TextToSpeech 引擎的语音音调。 |
5 |
setSpeechRate(float speechRate) 此方法设置语音速率。 |
6 |
shutdown() 此方法释放 TextToSpeech 引擎使用的资源。 |
7 |
stop() 此方法停止朗读。 |
示例
以下示例演示了 TextToSpeech 类的用法。它创建了一个基本的应用程序,允许您设置写入文本并朗读它。
要尝试此示例,您需要在实际设备上运行。
步骤 | 描述 |
---|---|
1 | 您将使用 Android Studio 在 com.example.sairamkrishna.myapplication 包下创建一个 Android 应用程序。 |
2 | 修改 src/MainActivity.java 文件以添加 TextToSpeech 代码。 |
3 | 修改布局 XML 文件 res/layout/activity_main.xml,如有需要,添加任何 GUI 组件。 |
4 | 运行应用程序,选择正在运行的 Android 设备,将应用程序安装到设备上并验证结果。 |
以下是 **src/MainActivity.java** 的内容。
package com.example.sairamkrishna.myapplication; import android.app.Activity; import android.os.Bundle; import android.speech.tts.TextToSpeech; import android.view.View; import android.widget.Button; import android.widget.EditText; import java.util.Locale; import android.widget.Toast; public class MainActivity extends Activity { TextToSpeech t1; EditText ed1; Button b1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ed1=(EditText)findViewById(R.id.editText); b1=(Button)findViewById(R.id.button); t1=new TextToSpeech(getApplicationContext(), new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if(status != TextToSpeech.ERROR) { t1.setLanguage(Locale.UK); } } }); b1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { String toSpeak = ed1.getText().toString(); Toast.makeText(getApplicationContext(), toSpeak,Toast.LENGTH_SHORT).show(); t1.speak(toSpeak, TextToSpeech.QUEUE_FLUSH, null); } }); } public void onPause(){ if(t1 !=null){ t1.stop(); t1.shutdown(); } super.onPause(); } }
以下是 **activity_main.xml** 的内容。
在以下代码中,**abc** 表示 tutorialspoint.com 的徽标。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:transitionGroup="true"> <TextView android:text="Text to Speech" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textview" android:textSize="35dp" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Tutorials point" android:id="@+id/textView" android:layout_below="@+id/textview" android:layout_centerHorizontal="true" android:textColor="#ff7aff24" android:textSize="35dp" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/imageView" android:src="@drawable/abc" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:theme="@style/Base.TextAppearance.AppCompat" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/editText" android:layout_below="@+id/imageView" android:layout_marginTop="46dp" android:hint="Enter Text" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:textColor="#ff7aff10" android:textColorHint="#ffff23d1" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Text to Speech" android:id="@+id/button" android:layout_below="@+id/editText" android:layout_centerHorizontal="true" android:layout_marginTop="46dp" /> </RelativeLayout>
以下是 **Strings.xml** 的内容。
<resources> <string name="app_name">My Application</string> </resources>
以下是 **AndroidManifest.xml** 的内容。
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.sairamkrishna.myapplication" > <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" > <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
让我们尝试运行您的应用程序。我假设您已将实际的 Android 移动设备连接到计算机。要从 Android Studio 运行应用程序,请打开项目的某个活动文件,然后单击工具栏中的运行 图标。在启动应用程序之前,Android Studio 将显示以下窗口,让您选择要在其中运行 Android 应用程序的位置。
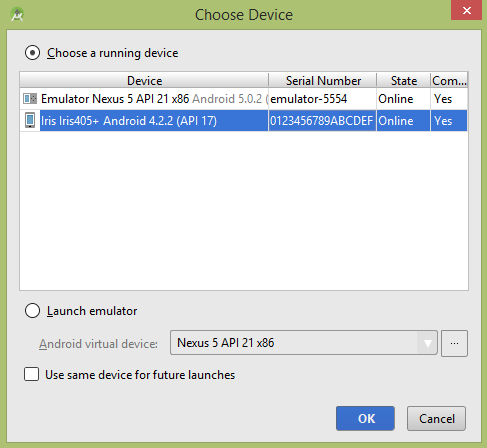
选择您的移动设备作为选项,然后检查您的移动设备,它将显示以下屏幕。
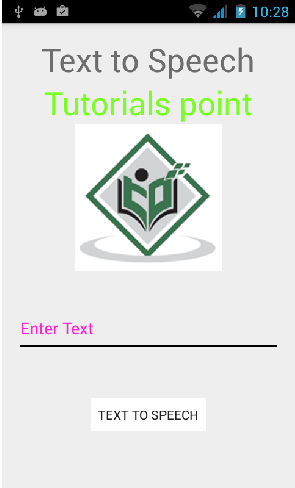
现在只需在字段中键入一些文本,然后单击下面的文字转语音按钮。将会出现通知,并朗读文本。如下图所示:
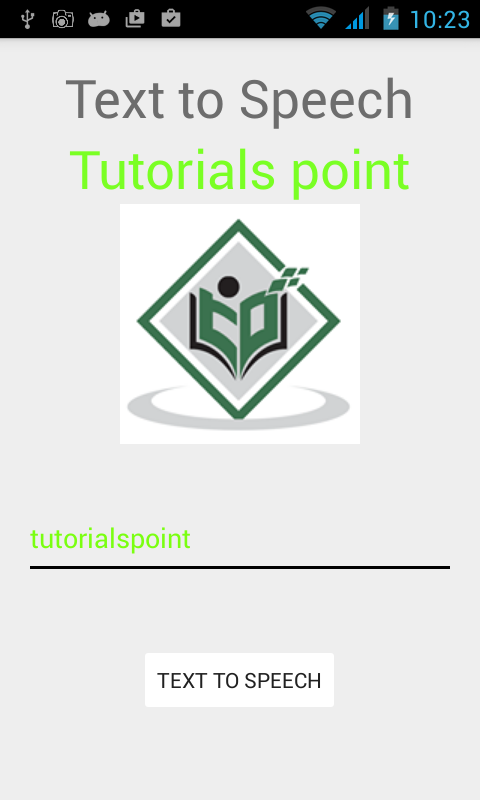
现在键入其他内容,并使用不同的语言环境重复此步骤。您将再次听到声音。如下图所示:
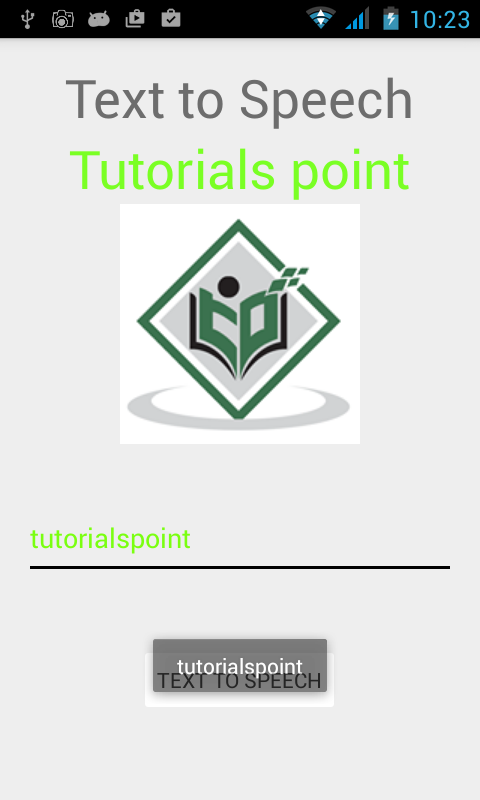