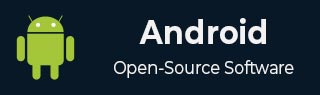
- Android 基础
- Android - 首页
- Android - 概览
- Android - 环境搭建
- Android - 架构
- Android - 应用组件
- Android - Hello World 示例
- Android - 资源
- Android - 活动 (Activity)
- Android - 服务 (Service)
- Android - 广播接收器 (Broadcast Receiver)
- Android - 内容提供器 (Content Provider)
- Android - 碎片 (Fragment)
- Android - 意图/过滤器 (Intents/Filters)
- Android - 用户界面
- Android - UI 布局
- Android - UI 控件
- Android - 事件处理
- Android - 样式和主题
- Android - 自定义组件
- Android 高级概念
- Android - 拖放
- Android - 通知
- 基于位置的服务
- Android - 发送邮件
- Android - 发送短信
- Android - 电话呼叫
- 发布 Android 应用
- Android 实用示例
- Android - 警报对话框
- Android - 动画
- Android - 音频捕获
- Android - 音频管理器
- Android - 自动完成
- Android - 最佳实践
- Android - 蓝牙
- Android - 相机
- Android - 剪贴板
- Android - 自定义字体
- Android - 数据备份
- Android - 开发者工具
- Android - 模拟器
- Android - Facebook 集成
- Android - 手势
- Android - Google 地图
- Android - 图片效果
- Android - ImageSwitcher
- Android - 内部存储
- Android - JetPlayer
- Android - JSON 解析器
- Android - LinkedIn 集成
- Android - 加载动画
- Android - 本地化
- Android - 登录界面
- Android - MediaPlayer
- Android - 多点触控
- Android - 导航
- Android - 网络连接
- Android - NFC 指南
- Android - PHP/MySQL
- Android - 进度圆圈
- Android - 进度条
- Android - 推送通知
- Android - RenderScript
- Android - RSS 阅读器
- Android - 屏幕录制
- Android - SDK 管理器
- Android - 传感器
- Android - 会话管理
- Android - Shared Preferences
- Android - SIP 协议
- Android - 拼写检查器
- Android - SQLite 数据库
- Android - 支持库
- Android - 测试
- Android - 文字转语音
- Android - TextureView
- Android - Twitter 集成
- Android - UI 设计
- Android - UI 模式
- Android - UI 测试
- Android - WebView 布局
- Android - Wi-Fi
- Android - 小部件
- Android - XML 解析器
- Android 实用资源
- Android - 问题解答
- Android - 实用资源
- Android - 讨论
Android - UI 设计
在本章中,我们将了解 Android 屏幕的不同 UI 组件。本章还涵盖了创建更出色 UI 设计的技巧,并解释了如何设计 UI。
UI 屏幕组件
Android 应用的典型用户界面由操作栏和应用内容区域组成。
- 主操作栏
- 视图控制
- 内容区域
- 拆分操作栏
这些组件也已在下图中显示 -
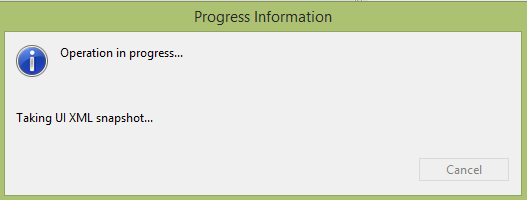
了解屏幕组件
Android 应用的基本单元是活动 (Activity)。UI 在 XML 文件中定义。在编译期间,XML 中的每个元素都会编译成等效的 Android GUI 类,其属性由方法表示。
视图 (View) 和视图组 (ViewGroup)
一个活动 (Activity) 由视图 (View) 组成。视图 (View) 只是出现在屏幕上的一个小部件。它可以是按钮等。一个或多个视图可以组合成一个 ViewGroup。ViewGroup 的示例包括布局。
布局类型
有许多类型的布局。其中一些列在下面 -
- 线性布局 (LinearLayout)
- 绝对布局 (AbsoluteLayout)
- 表格布局 (TableLayout)
- 框架布局 (FrameLayout)
- 相对布局 (RelativeLayout)
线性布局 (LinearLayout)
线性布局进一步细分为水平和垂直布局。这意味着它可以在一列或一行中排列视图。以下是包含文本视图的线性布局(垂直)的代码。
<?xml version=”1.0” encoding=”utf-8”?> <LinearLayout xmlns:android=”http://schemas.android.com/apk/res/android” android:layout_width=”fill_parent” android:layout_height=”fill_parent” android:orientation=”vertical” > <TextView android:layout_width=”fill_parent” android:layout_height=”wrap_content” android:text=”@string/hello” /> </LinearLayout>
绝对布局 (AbsoluteLayout)
AbsoluteLayout 允许您指定其子元素的确切位置。它可以像这样声明。
<AbsoluteLayout android:layout_width=”fill_parent” android:layout_height=”fill_parent” xmlns:android=”http://schemas.android.com/apk/res/android” > <Button android:layout_width=”188dp” android:layout_height=”wrap_content” android:text=”Button” android:layout_x=”126px” android:layout_y=”361px” /> </AbsoluteLayout>
表格布局 (TableLayout)
TableLayout 将视图分组到行和列中。它可以像这样声明。
<TableLayout xmlns:android=”http://schemas.android.com/apk/res/android” android:layout_height=”fill_parent” android:layout_width=”fill_parent” > <TableRow> <TextView android:text=”User Name:” android:width =”120dp” /> <EditText android:id=”@+id/txtUserName” android:width=”200dp” /> </TableRow> </TableLayout>
相对布局 (RelativeLayout)
RelativeLayout 允许您指定子视图相对于彼此的位置。它可以像这样声明。
<RelativeLayout android:id=”@+id/RLayout” android:layout_width=”fill_parent” android:layout_height=”fill_parent” xmlns:android=”http://schemas.android.com/apk/res/android” > </RelativeLayout>
框架布局 (FrameLayout)
FrameLayout 是屏幕上的一个占位符,您可以使用它来显示单个视图。它可以像这样声明。
<?xml version=”1.0” encoding=”utf-8”?> <FrameLayout android:layout_width=”wrap_content” android:layout_height=”wrap_content” android:layout_alignLeft=”@+id/lblComments” android:layout_below=”@+id/lblComments” android:layout_centerHorizontal=”true” > <ImageView android:src = “@drawable/droid” android:layout_width=”wrap_content” android:layout_height=”wrap_content” /> </FrameLayout>
除了这些属性之外,还有其他属性在所有视图和 ViewGroup 中都是通用的。它们列在下面 -
序号 | 视图 & 描述 |
---|---|
1 |
layout_width 指定 View 或 ViewGroup 的宽度 |
2 |
layout_height 指定 View 或 ViewGroup 的高度 |
3 |
layout_marginTop 指定 View 或 ViewGroup 上方额外的空间 |
4 |
layout_marginBottom 指定 View 或 ViewGroup 下方额外的空间 |
5 |
layout_marginLeft 指定 View 或 ViewGroup 左侧额外的空间 |
6 |
layout_marginRight 指定 View 或 ViewGroup 右侧额外的空间 |
7 |
layout_gravity 指定子视图如何定位 |
8 |
layout_weight 指定应分配给 View 的布局中额外空间的多少 |
测量单位
在指定 Android UI 上元素的大小时,您应该记住以下测量单位。
序号 | 单位 & 描述 |
---|---|
1 |
dp 密度无关像素。1 dp 在 160 dpi 屏幕上相当于一个像素。 |
2 |
sp 缩放无关像素。这类似于 dp,建议用于指定字体大小 |
3 |
pt 磅。磅定义为 1/72 英寸,基于物理屏幕尺寸。 |
4 |
px 像素。对应于屏幕上的实际像素 |
屏幕密度
序号 | 密度 & DPI |
---|---|
1 |
低密度 (ldpi) 120 dpi |
2 |
中密度 (mdpi) 160 dpi |
3 |
高密度 (hdpi) 240 dpi |
4 |
超高密度 (xhdpi) 320 dpi |
优化布局
以下是一些创建高效布局的指南。
- 避免不必要的嵌套
- 避免使用太多视图
- 避免深度嵌套