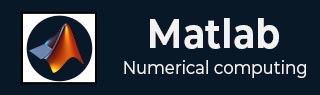
- Matlab 教程
- MATLAB - 首页
- MATLAB - 概述
- MATLAB - 功能
- MATLAB - 环境设置
- MATLAB - 编辑器
- MATLAB - 在线版
- MATLAB - 工作区
- MATLAB - 语法
- MATLAB - 变量
- MATLAB - 命令
- MATLAB - 数据类型
- MATLAB - 运算符
- MATLAB - 日期和时间
- MATLAB - 数字
- MATLAB - 随机数
- MATLAB - 字符串和字符
- MATLAB - 文本格式化
- MATLAB - 时间表
- MATLAB - M 文件
- MATLAB - 冒号表示法
- MATLAB - 数据导入
- MATLAB - 数据导出
- MATLAB - 数据归一化
- MATLAB - 预定义变量
- MATLAB - 决策
- MATLAB - 决策语句
- MATLAB - if 语句
- MATLAB - if else 语句
- MATLAB - if…elseif else 语句
- MATLAB - 嵌套 if 语句
- MATLAB - switch 语句
- MATLAB - 嵌套 switch
- MATLAB - 循环
- MATLAB - 循环
- MATLAB - for 循环
- MATLAB - while 循环
- MATLAB - 嵌套循环
- MATLAB - break 语句
- MATLAB - continue 语句
- MATLAB - end 语句
- MATLAB - 数组
- MATLAB - 数组
- MATLAB - 向量
- MATLAB - 转置运算符
- MATLAB - 数组索引
- MATLAB - 多维数组
- MATLAB - 兼容数组
- MATLAB - 分类数组
- MATLAB - 元胞数组
- MATLAB - 矩阵
- MATLAB - 稀疏矩阵
- MATLAB - 表格
- MATLAB - 结构体
- MATLAB - 数组乘法
- MATLAB - 数组除法
- MATLAB - 数组函数
- MATLAB - 函数
- MATLAB - 函数
- MATLAB - 函数参数
- MATLAB - 匿名函数
- MATLAB - 嵌套函数
- MATLAB - return 语句
- MATLAB - 无返回值函数
- MATLAB - 局部函数
- MATLAB - 全局变量
- MATLAB - 函数句柄
- MATLAB - 滤波器函数
- MATLAB - 阶乘
- MATLAB - 私有函数
- MATLAB - 子函数
- MATLAB - 递归函数
- MATLAB - 函数优先级顺序
- MATLAB - map 函数
- MATLAB - mean 函数
- MATLAB - end 函数
- MATLAB - 错误处理
- MATLAB - 错误处理
- MATLAB - try…catch 语句
- MATLAB - 调试
- MATLAB - 绘图
- MATLAB - 绘图
- MATLAB - 绘制数组
- MATLAB - 绘制向量
- MATLAB - 条形图
- MATLAB - 直方图
- MATLAB - 图形
- MATLAB - 二维线图
- MATLAB - 三维图
- MATLAB - 格式化绘图
- MATLAB - 对数坐标轴绘图
- MATLAB - 绘制误差条
- MATLAB - 绘制三维等值线图
- MATLAB - 极坐标图
- MATLAB - 散点图
- MATLAB - 绘制表达式或函数
- MATLAB - 绘制矩形
- MATLAB - 绘制频谱图
- MATLAB - 绘制网格曲面
- MATLAB - 绘制正弦波
- MATLAB - 插值
- MATLAB - 插值
- MATLAB - 线性插值
- MATLAB - 二维数组插值
- MATLAB - 三维数组插值
- MATLAB - 多项式
- MATLAB - 多项式
- MATLAB - 多项式加法
- MATLAB - 多项式乘法
- MATLAB - 多项式除法
- MATLAB - 多项式的导数
- MATLAB - 变换
- MATLAB - 变换
- MATLAB - 拉普拉斯变换
- MATLAB - 拉普拉斯滤波器
- MATLAB - 高斯-拉普拉斯滤波器
- MATLAB - 逆傅里叶变换
- MATLAB - 傅里叶变换
- MATLAB - 快速傅里叶变换
- MATLAB - 二维逆余弦变换
- MATLAB - 向坐标轴添加图例
- MATLAB - 面向对象
- MATLAB - 面向对象编程
- MATLAB - 类和对象
- MATLAB - 函数重载
- MATLAB - 运算符重载
- MATLAB - 用户自定义类
- MATLAB - 复制对象
- MATLAB - 代数
- MATLAB - 线性代数
- MATLAB - 高斯消元法
- MATLAB - 高斯-约当消元法
- MATLAB - 简化行阶梯形
- MATLAB - 特征值和特征向量
- MATLAB - 积分
- MATLAB - 积分
- MATLAB - 二重积分
- MATLAB - 梯形法则
- MATLAB - 辛普森法则
- MATLAB - 其他
- MATLAB - 微积分
- MATLAB - 微分
- MATLAB - 矩阵的逆
- MATLAB - GNU Octave
- MATLAB - Simulink
- MATLAB - 有用资源
- MATLAB - 快速指南
- MATLAB - 有用资源
- MATLAB - 讨论
MATLAB - 多项式除法
多项式除法是将一个多项式除以另一个多项式的过程。在 MATLAB 中,可以使用 `deconv` 函数执行多项式除法,该函数计算两个多项式的解卷积。由于多项式除法本质上是多项式乘法的逆运算,因此 `deconv` 函数可用于多项式除法。
在 Matlab 中使用 deconv() 函数
MATLAB 中的 `deconv()` 函数可以帮助你将一个多项式除以另一个多项式。
语法
[x,r] = deconv(y,h)
假设你有两个多项式 P(x) 和 Q(x)。当你将 P(x) 除以 Q(x) 时,你会得到商 X(x) 和余数 R(x)。Matlab 中的 `deconv()` 函数会为你完成这个除法。
例如,如果你有 P(x) = 6x³ + 5x² + 4x + 3 和 Q(x) = 2x + 1,使用 `deconv()` 将得到商 X(x) = 3x² + 2x + 1 和余数 R(x) = 0。
在 Matlab 中,你使用 `deconv(y, h)`,其中 y 是 P(x) 的系数,h 是 Q(x) 的系数。该函数返回 X(x) 的系数作为商和 R(x) 作为余数。
示例 1:多项式除法
让我们考虑以下两个多项式:
P(x) = 6x3 + 5x2 + 4x + 3 Q(x) = 2x + 1
上述多项式的系数如下:
P = [ 6 5 4 3 ]; Q = [2 1]
这是一个 Matlab 代码:
% Define the coefficients of the polynomials P = [6 5 4 3]; % Coefficients of P(x) = 6x^3 + 5x^2 + 4x + 3 Q = [2 1]; % Coefficients of Q(x) = 2x + 1 % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder);
**在上面的示例中** - 将 P(x) 除以 Q(x) 的结果,即除法产生的多项式。无法被 Q(x) 进一步除的多项式。
商 X(x) 将是一个 2 次多项式,因为 P(x) 是 3 次多项式,而 Q(x) 是 1 次多项式。
余数 R(x) 将是一个常数项,因为它表示除法后的剩余部分。
代码执行后,我们将得到以下输出:
>> % Define the coefficients of the polynomials P = [6 5 4 3]; % Coefficients of P(x) = 6x^3 + 5x^2 + 4x + 3 Q = [2 1]; % Coefficients of Q(x) = 2x + 1 % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder); Quotient coefficients: 3.0000 1.0000 1.5000 Remainder coefficients: 0 0 0 1.5000 >>
示例 2:高次多项式
让我们除以两个高次多项式。
Polynomials: P(x) = 4x5 + 3x4 + 2x3 + x2 + 5x + 6 Q(x) = 2x2 + x + 1 Coefficients: P = [ 4 3 2 1 5 6 ] Q = [ 2 1 1 ]
为了解决上述多项式除法,我们使用的代码是:
% Define the coefficients of the polynomials P = [4 3 2 1 5 6]; % Coefficients of P(x) = 4x^5 + 3x^4 + 2x^3 + x^2 + 5x + 6 Q = [2 1 1]; % Coefficients of Q(x) = 2x^2 + x + 1 % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder);
在上面的代码中,我们有:
- P(x) 是 5 次多项式。
- Q(x) 是 2 次多项式。
- 商将是一个 3 次多项式,余数将是一个 1 次多项式。
在 Matlab 命令窗口中执行代码后,我们将得到以下输出:
>> % Define the coefficients of the polynomials P = [4 3 2 1 5 6]; % Coefficients of P(x) = 4x^5 + 3x^4 + 2x^3 + x^2 + 5x + 6 Q = [2 1 1]; % Coefficients of Q(x) = 2x^2 + x + 1 % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder); Quotient coefficients: 2.0000 0.5000 -0.2500 0.3750 Remainder coefficients: 0 0 0 0 4.8750 5.6250 >>
示例 3:余数为零的除法
考虑两个多项式,其中除法结果的余数为零。
Polynomials: P(x) = x4 + 4x3 + 6x2 + 4x + 1 (which is the expansion of (x+1)4) Q(x) = x + 1 Coefficients: P = [ 1 4 6 4 1 ] Q = [ 1 1 ]
我们的 Matlab 代码是:
% Define the coefficients of the polynomials P = [1 4 6 4 1]; % Coefficients of P(x) = x^4 + 4x^3 + 6x^2 + 4x + 1 Q = [1 1]; % Coefficients of Q(x) = x + 1 % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder);
在上面的代码中:
- 由于 P(x) 被 Q(x) 整除,因此余数将为零。
- 商将是 P(x) 除以 Q(x) 的结果,即 (x+1)³
在 Matlab 命令窗口中执行代码后,输出为:
>> % Define the coefficients of the polynomials P = [1 4 6 4 1]; % Coefficients of P(x) = x^4 + 4x^3 + 6x^2 + 4x + 1 Q = [1 1]; % Coefficients of Q(x) = x + 1 % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder); Quotient coefficients: 1 3 3 1 Remainder coefficients: 0 0 0 0 0 >>
示例 4:多项式除以常数
如果将多项式除以常数(0 次多项式),则商将只是原始多项式除以该常数。
Polynomials: P(x) = 3x3 + 6x2 + 9x + 12 Q(x) = 3 (constant) Coefficients: P = [ 3 6 9 12 ] Q = [ 3 ]
我们的 Matlab 代码是:
% Define the coefficients of the polynomials P = [3 6 9 12]; % Coefficients of P(x) = 3x^3 + 6x^2 + 9x + 12 Q = [3]; % Coefficients of Q(x) = 3 (constant) % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder);
在 Matlab 命令窗口中执行代码后,输出为:
>> % Define the coefficients of the polynomials P = [3 6 9 12]; % Coefficients of P(x) = 3x^3 + 6x^2 + 9x + 12 Q = [3]; % Coefficients of Q(x) = 3 (constant) % Perform the polynomial division [quotient, remainder] = deconv(P, Q); % Display the results disp('Quotient coefficients:'); disp(quotient); disp('Remainder coefficients:'); disp(remainder); Quotient coefficients: 1 2 3 4 Remainder coefficients: 0 0 0 0 >>