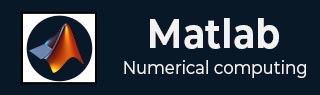
- Matlab 教程
- MATLAB - 首页
- MATLAB - 概述
- MATLAB - 特性
- MATLAB - 环境设置
- MATLAB - 编辑器
- MATLAB - 在线
- MATLAB - 工作区
- MATLAB - 语法
- MATLAB - 变量
- MATLAB - 命令
- MATLAB - 数据类型
- MATLAB - 运算符
- MATLAB - 日期和时间
- MATLAB - 数字
- MATLAB - 随机数
- MATLAB - 字符串和字符
- MATLAB - 文本格式化
- MATLAB - 时间表
- MATLAB - M 文件
- MATLAB - 冒号表示法
- MATLAB - 数据导入
- MATLAB - 数据输出
- MATLAB - 数据归一化
- MATLAB - 预定义变量
- MATLAB - 决策制定
- MATLAB - 决策
- MATLAB - If End 语句
- MATLAB - If Else 语句
- MATLAB - If…Elseif Else 语句
- MATLAB - 嵌套 If 语句
- MATLAB - Switch 语句
- MATLAB - 嵌套 Switch
- MATLAB - 循环
- MATLAB - 循环
- MATLAB - For 循环
- MATLAB - While 循环
- MATLAB - 嵌套循环
- MATLAB - Break 语句
- MATLAB - Continue 语句
- MATLAB - End 语句
- MATLAB - 数组
- MATLAB - 数组
- MATLAB - 向量
- MATLAB - 转置运算符
- MATLAB - 数组索引
- MATLAB - 多维数组
- MATLAB - 兼容数组
- MATLAB - 分类数组
- MATLAB - 元胞数组
- MATLAB - 矩阵
- MATLAB - 稀疏矩阵
- MATLAB - 表格
- MATLAB - 结构体
- MATLAB - 数组乘法
- MATLAB - 数组除法
- MATLAB - 数组函数
- MATLAB - 函数
- MATLAB - 函数
- MATLAB - 函数参数
- MATLAB - 匿名函数
- MATLAB - 嵌套函数
- MATLAB - 返回语句
- MATLAB - 空函数
- MATLAB - 局部函数
- MATLAB - 全局变量
- MATLAB - 函数句柄
- MATLAB - 滤波器函数
- MATLAB - 阶乘
- MATLAB - 私有函数
- MATLAB - 子函数
- MATLAB - 递归函数
- MATLAB - 函数优先级顺序
- MATLAB - Map 函数
- MATLAB - Mean 函数
- MATLAB - End 函数
- MATLAB - 错误处理
- MATLAB - 错误处理
- MATLAB - Try...Catch 语句
- MATLAB - 调试
- MATLAB - 绘图
- MATLAB - 绘图
- MATLAB - 绘制数组
- MATLAB - 绘制向量
- MATLAB - 条形图
- MATLAB - 直方图
- MATLAB - 图形
- MATLAB - 2D 线性图
- MATLAB - 3D 图
- MATLAB - 格式化绘图
- MATLAB - 对数坐标轴绘图
- MATLAB - 绘制误差条
- MATLAB - 绘制 3D 等值线
- MATLAB - 极坐标图
- MATLAB - 散点图
- MATLAB - 绘制表达式或函数
- MATLAB - 绘制矩形
- MATLAB - 绘制频谱图
- MATLAB - 绘制网格曲面
- MATLAB - 绘制正弦波
- MATLAB - 插值
- MATLAB - 插值
- MATLAB - 线性插值
- MATLAB - 2D 数组插值
- MATLAB - 3D 数组插值
- MATLAB - 多项式
- MATLAB - 多项式
- MATLAB - 多项式加法
- MATLAB - 多项式乘法
- MATLAB - 多项式除法
- MATLAB - 多项式的导数
- MATLAB - 变换
- MATLAB - 变换
- MATLAB - 拉普拉斯变换
- MATLAB - 拉普拉斯滤波器
- MATLAB - 高斯-拉普拉斯滤波器
- MATLAB - 逆傅里叶变换
- MATLAB - 傅里叶变换
- MATLAB - 快速傅里叶变换
- MATLAB - 2D 逆余弦变换
- MATLAB - 向坐标轴添加图例
- MATLAB - 面向对象
- MATLAB - 面向对象编程
- MATLAB - 类和对象
- MATLAB - 函数重载
- MATLAB - 运算符重载
- MATLAB - 用户自定义类
- MATLAB - 复制对象
- MATLAB - 代数
- MATLAB - 线性代数
- MATLAB - 高斯消元法
- MATLAB - 高斯-约旦消元法
- MATLAB - 降阶行阶梯形式
- MATLAB - 特征值和特征向量
- MATLAB - 积分
- MATLAB - 积分
- MATLAB - 二重积分
- MATLAB - 梯形法则
- MATLAB - 辛普森法则
- MATLAB - 其他
- MATLAB - 微积分
- MATLAB - 微分
- MATLAB - 矩阵的逆
- MATLAB - GNU Octave
- MATLAB - Simulink
- MATLAB - 有用资源
- MATLAB - 快速指南
- MATLAB - 有用资源
- MATLAB - 讨论
MATLAB - 用户自定义类
MATLAB 传统上以其强大的矩阵运算和内置函数而闻名,但它也支持面向对象编程 (OOP)。MATLAB 中的用户自定义类允许您创建自定义数据类型,这些数据类型封装了数据和对该数据进行操作的函数(方法)。这种方法可以帮助组织代码,提高可重用性,并更自然地对复杂系统进行建模。
什么是类?
类是创建对象(实例)的蓝图。它定义了对象将具有的属性(数据)和方法(函数)。
定义类
在 MATLAB 中,您可以使用 classdef 关键字定义类。以下是一个简单的示例:
classdef MyClass properties Property1 end methods function obj = MyClass(val) if nargin > 0 obj.Property1 = val; end end function output = myMethod(obj, input) output = obj.Property1 + input; end end end
示例的分解
1. 类定义
classdef MyClass
此行声明了一个名为 MyClass 的新类。
2. 属性块
properties Property1 end
属性是保存类对象的变量。在此示例中,MyClass 有一个属性 Property1。
3. 方法块
methods
方法是定义类行为的函数。它们对类的对象进行操作。
4. 构造函数方法
function obj = MyClass(val) if nargin > 0 obj.Property1 = val; end end
构造函数方法是 MATLAB 在创建新对象时调用的特殊函数。构造函数方法的名称与类名称相同。在此,如果提供了输入值 val,则构造函数会初始化 Property1。
5. 其他方法
function output = myMethod(obj, input) output = obj.Property1 + input; end
这是一个名为 myMethod 的常规方法。它接受两个输入:obj(对象本身)和 input。它返回 Property1 和 input 的总和。
创建对象
要创建 MyClass 的对象,您可以使用构造函数方法
obj = MyClass(10); disp(obj.Property1); % Here Output: 10
使用方法
您可以使用点表示法在对象上调用方法
result = obj.myMethod(5); disp(result); % Here Output: 15
使用类的优势
- 封装 - 将相关的数据和函数组合在一起,使代码更模块化,更易于管理。
- 可重用性 - 定义类后,可以在项目的不同部分或不同项目中重用它。
- 抽象 - 隐藏内部实现细节,仅公开必要的接口。
- 继承 - 创建继承现有类的属性和方法的新类,从而促进代码重用和层次结构类结构。
示例 1:点类
以下是一个更实用的示例,定义一个类来表示二维点。
classdef Point properties X Y end methods function obj = Point(x, y) if nargin > 0 obj.X = x; obj.Y = y; end end function obj = set.X(obj, x) if x >= 0 obj.X = x; else error('X must be non-negative'); end end function obj = set.Y(obj, y) if y >= 0 obj.Y = y; else error('Y must be non-negative'); end end function distance = distanceToOrigin(obj) distance = sqrt(obj.X^2 + obj.Y^2); end end end
使用点类
p = Point(3, 4); disp(p.X); % Output: 3 disp(p.Y); % Output: 4 dist = p.distanceToOrigin(); disp(dist); % Output: 5
在此示例中 -
- 点类具有 X 和 Y 属性来存储坐标。
- 构造函数初始化这些属性。
- 设置方法(set.X 和 set.Y)验证坐标是否为非负数。
- distanceToOrigin 方法计算从原点到点的欧几里得距离。
示例 2:圆形类
此类将包括圆的半径和中心的属性,以及计算面积、周长以及检查点是否在圆内的的方法。
圆形类定义
classdef Circle properties Radius Center end methods % Constructor function obj = Circle(radius, center) if nargin > 0 obj.Radius = radius; obj.Center = center; end end % Method to calculate area function area = area(obj) area = pi * obj.Radius^2; end % Method to calculate circumference function circumference = circumference(obj) circumference = 2 * pi * obj.Radius; end % Method to check if a point is inside the circle function isIn = isPointInside(obj, point) distance = sqrt((point(1) - obj.Center(1))^2 + (point(2) - obj.Center(2))^2); isIn = distance <= obj.Radius; end end end
圆形类的分解
1. 类定义
classdef Circle
2. 属性
properties Radius Center end
圆形类有两个属性:Radius(圆的半径)和 Center(一个 2 元素向量,表示圆中心的 x 和 y 坐标)。
3. 构造函数方法
function obj = Circle(radius, center) if nargin > 0 obj.Radius = radius; obj.Center = center; end end
如果提供了输入参数,则构造函数会初始化 Radius 和 Center 属性。
4. 面积方法
function area = area(obj) area = pi * obj.Radius^2; end
此方法使用公式 𝜋 x Radius2 计算圆的面积。
5. 周长方法
function circumference = circumference(obj) circumference = 2 * pi * obj.Radius; end
此方法使用公式 2 x x Radius 计算圆的周长。
6. 点在圆内检查方法。
function isIn = isPointInside(obj, point) distance = sqrt((point(1) - obj.Center(1))^2 + (point(2) - obj.Center(2))^2); isIn = distance <= obj.Radius; end
此方法检查给定点是否在圆内。它计算点到圆中心的距离,并检查此距离是否小于或等于半径。
使用圆形类
要创建圆形对象并使用其方法,您可以执行以下操作。
% Create a Circle object with radius 5 and center at (0, 0) c = Circle(5, [0, 0]); % Calculate the area a = c.area(); disp(['Area: ', num2str(a)]); % Output: Area: 78.5398 % Calculate the circumference circ = c.circumference(); disp(['Circumference: ', num2str(circ)]); % Output: Circumference: 31.4159 % Check if a point is inside the circle point = [3, 4]; isInside = c.isPointInside(point); disp(['Is the point inside the circle? ', num2str(isInside)]); % Output: Is the point inside the circle? 1 % Check if another point is inside the circle point = [6, 0]; isInside = c.isPointInside(point); disp(['Is the point inside the circle? ', num2str(isInside)]); % Output: Is the point inside the circle? 0