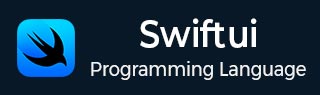
- SwiftUI 教程
- SwiftUI - 首页
- SwiftUI - 概述
- SwiftUI vs UIkit
- SwiftUI 环境
- SwiftUI - 环境设置
- SwiftUI - 基本组件
- SwiftUI - 构建第一个应用程序
- SwiftUI 视图
- SwiftUI - 视图
- SwiftUI - 自定义文本视图
- SwiftUI - 自定义图像视图
- SwiftUI - 堆栈
- SwiftUI 绘制形状
- SwiftUI - 形状
- SwiftUI - 绘制线条
- SwiftUI - 绘制矩形
- SwiftUI - 绘制圆角矩形
- SwiftUI - 绘制三角形
- SwiftUI - 绘制圆形
- SwiftUI - 绘制星形
- SwiftUI - 绘制多边形
- SwiftUI - 绘制饼图
- SwiftUI - 使用内置形状
- SwiftUI - 文本
- SwiftUI - 文本视图
- SwiftUI - 文本输入和输出
- SwiftUI - 颜色
- SwiftUI - 颜色
- SwiftUI - 颜色选择器
- SwiftUI - 渐变
- SwiftUI - 调整颜色
- SwiftUI - 效果
- SwiftUI - 效果
- SwiftUI - 混合效果
- SwiftUI - 模糊效果
- SwiftUI - 阴影效果
- SwiftUI - 悬停效果
- SwiftUI - 动画
- SwiftUI - 动画
- SwiftUI - 创建动画
- SwiftUI - 创建显式动画
- SwiftUI - 多个动画
- SwiftUI - 转场
- SwiftUI - 非对称转场
- SwiftUI - 自定义转场
- SwiftUI - 图像
- SwiftUI - 图像
- SwiftUI - 图像作为背景
- SwiftUI - 旋转图像
- SwiftUI - 媒体
- SwiftUI - 视图布局
- SwiftUI - 视图布局
- SwiftUI - 视图大小
- SwiftUI - 视图间距
- SwiftUI - 视图填充
- SwiftUI - 列表和表格
- SwiftUI - 列表
- SwiftUI - 静态列表
- SwiftUI - 动态列表
- SwiftUI - 自定义列表
- SwiftUI - 表格
- SwiftUI - 表单
- SwiftUI - 表单
- SwiftUI - 将表单拆分为多个部分
- SwiftUI 有用资源
- SwiftUI - 有用资源
- SwiftUI - 讨论
SwiftUI - 绘制圆形
圆形是几何学中一种封闭的图形或形状。它是由一组到平面上的一个固定点的距离相等的点形成的,其中固定距离称为圆的半径,固定点称为圆心。圆上所有点到圆心的距离都相同。因此,在本节中,我们将学习如何在 SwiftUI 中绘制圆形。
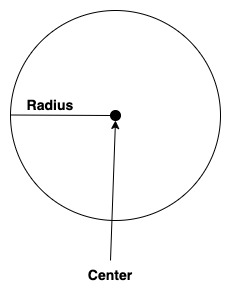
在 SwiftUI 中绘制圆形
在 SwiftUI 中,我们将绘制各种类型的圆形,例如实心圆、无填充或圆形描边的圆以及自定义圆形。为了绘制圆形,SwiftUI 提供了一个名为 addArc() 的内置方法。此方法根据指定的半径和角度计算起点和终点,在这些点之间创建曲线,然后将这些曲线插入路径以创建圆形。
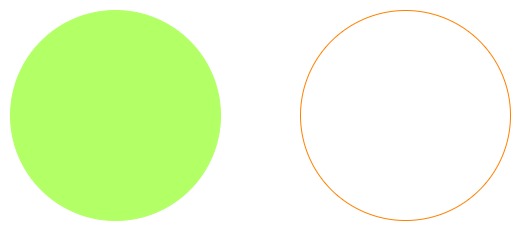
语法
以下是语法:
func addArc( center: CGPoint, radius: CGFloat, startAngle: Angle, endAngle: Angle, clockwise: Bool, transform: CGAffineTransform = .identity )
参数
此函数接受以下参数:
- center: 此参数的坐标表示圆弧的中心。
- radius: 此参数的坐标表示圆弧的半径。
- startAngle: 它表示圆弧起点的角度。它从正 x 轴计算。
- endAngle: 它表示圆弧终点的角度。它从正 x 轴计算。
- clockwise 如果此参数的值设置为 true,则圆弧将按顺时针方向创建。否则,圆弧将按逆时针方向创建。
- transform: 用于在将圆弧添加到路径之前对其应用转换。默认情况下,它设置为恒等转换。
在 SwiftUI 中绘制圆形的步骤
请按照以下步骤在 SwiftUI 中绘制一个简单的实心圆:
步骤 1:初始化路径
要创建圆形,首先我们需要初始化 Path 并提供详细的说明在闭包中。路径允许我们通过指定一系列连接的点和线来创建复杂的图形。
Path() {}
步骤 2:移动到圆形中心
现在我们使用 move(to:) 方法移动到给定画布中圆形的中心。或者我们可以说我们使用 move(to:) 方法到达圆形中心。
Path(){ yPath in yPath.move(to: CGPoint(x: 200, y: 200)) }
步骤 3:绘制实心圆
现在我们调用 addArc() 方法根据初始化的参数绘制圆形,例如圆形的中心应位于坐标 (200, 200) 处,圆形的半径为 100,起始和结束角度为 0.0 度和 360.0 度,圆弧的方向为顺时针方向。
Path(){ yPath in yPath.move(to: CGPoint(x: 200, y: 200)) yPath.addArc(center: CGPoint(x: 200, y:200), radius: 100, startAngle: Angle(degrees: 0.0), endAngle: Angle(degrees: 360.0), clockwise: true) }
步骤 4:填充圆形
我们可以使用 .fill() 修饰符指定圆形的颜色。默认情况下,圆形的颜色为黑色。或者如果我们只想描边,则可以使用 .stroke() 修饰符。
Path(){ yPath in yPath.move(to: CGPoint(x: 200, y: 200)) yPath.addArc(center: CGPoint(x: 200, y:200), radius: 100, startAngle: Angle(degrees: 0.0), endAngle: Angle(degrees: 360.0), clockwise: true) }.fill(Color.cyan)
因此,圆形将如以下图像所示创建。
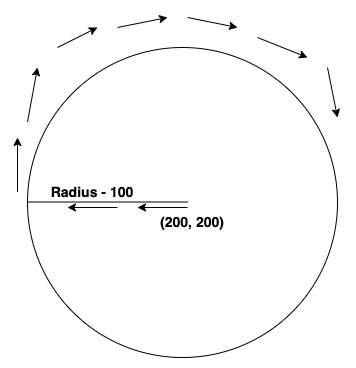
示例 1
以下 SwiftUI 程序用于绘制实心圆。
import SwiftUI struct ContentView: View { var body: some View { Path(){ yPath in yPath.move(to: CGPoint(x: 200, y: 200)) yPath.addArc(center: CGPoint(x: 200, y:200), radius: 100, startAngle: Angle(degrees: 0.0), endAngle: Angle(degrees: 360.0), clockwise: true) }.fill(Color.cyan) } } #Preview { ContentView() }
输出
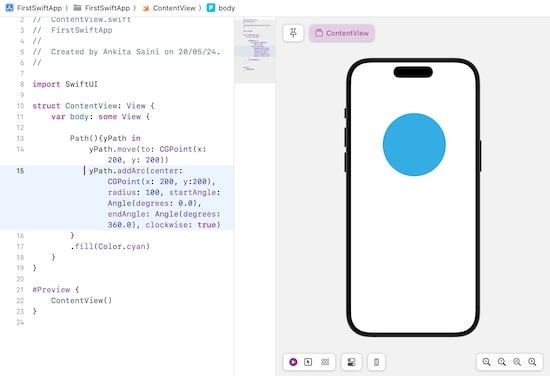
示例 2
以下 SwiftUI 程序用于绘制描边圆或带边界的圆。
import SwiftUI struct ContentView: View { var body: some View { Path(){ yPath in yPath.move(to: CGPoint(x: 200, y: 200)) yPath.addArc(center: CGPoint(x: 200, y:200), radius: 100, startAngle: Angle(degrees: 0.0), endAngle: Angle(degrees: 360.0), clockwise: true) }.stroke(Color.blue, lineWidth: 4) } } #Preview { ContentView() }
输出
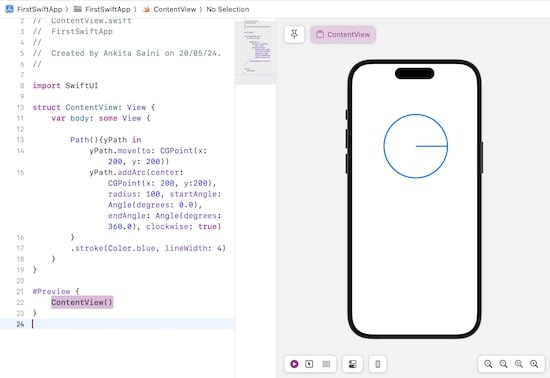
示例 3
以下 SwiftUI 程序用于绘制自定义圆形。其中圆形是根据给定的中心坐标和半径创建的。
import SwiftUI struct Circle : Shape { var radius : CGFloat var center : CGPoint func path(in rect: CGRect) -> Path { var mPath = Path() // Calculating the coordinates of center let Xcenter = center.x - rect.origin.x let Ycenter = center.y - rect.origin.y // For circle mPath.addArc( center: CGPoint(x: Xcenter, y: Ycenter), radius: radius, startAngle: Angle(degrees: 0.0), endAngle: Angle(degrees: 360.0), clockwise: true ) return mPath } } struct ContentView: View { var body: some View { Circle(radius: 50, center: CGPoint(x: 100, y: 200)) .stroke(Color.mint, lineWidth: 4) } } #Preview { ContentView() }
输出
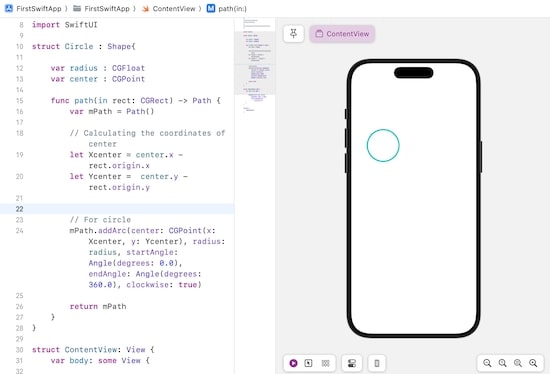
广告