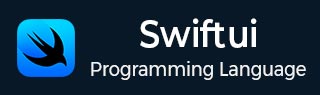
- SwiftUI 教程
- SwiftUI - 首页
- SwiftUI - 概述
- SwiftUI vs UIKit
- SwiftUI 环境
- SwiftUI - 环境设置
- SwiftUI - 基本组件
- SwiftUI - 构建第一个应用程序
- SwiftUI 视图
- SwiftUI - 视图
- SwiftUI - 自定义文本视图
- SwiftUI - 自定义图像视图
- SwiftUI - 堆栈
- SwiftUI 绘制形状
- SwiftUI - 形状
- SwiftUI - 绘制线条
- SwiftUI - 绘制矩形
- SwiftUI - 绘制圆角矩形
- SwiftUI - 绘制三角形
- SwiftUI - 绘制圆形
- SwiftUI - 绘制星形
- SwiftUI - 绘制多边形
- SwiftUI - 绘制饼图
- SwiftUI - 使用内置形状
- SwiftUI - 文本
- SwiftUI - 文本视图
- SwiftUI - 文本输入和输出
- SwiftUI - 颜色
- SwiftUI - 颜色
- SwiftUI - 颜色选择器
- SwiftUI - 渐变
- SwiftUI - 调整颜色
- SwiftUI - 效果
- SwiftUI - 效果
- SwiftUI - 混合效果
- SwiftUI - 模糊效果
- SwiftUI - 阴影效果
- SwiftUI - 悬停效果
- SwiftUI - 动画
- SwiftUI - 动画
- SwiftUI - 创建动画
- SwiftUI - 创建显式动画
- SwiftUI - 多个动画
- SwiftUI - 转场
- SwiftUI - 非对称转场
- SwiftUI - 自定义转场
- SwiftUI - 图片
- SwiftUI - 图片
- SwiftUI - 图片作为背景
- SwiftUI - 旋转图片
- SwiftUI - 媒体
- SwiftUI - 视图布局
- SwiftUI - 视图布局
- SwiftUI - 视图大小
- SwiftUI - 视图间距
- SwiftUI - 视图填充
- SwiftUI - 列表和表格
- SwiftUI - 列表
- SwiftUI - 静态列表
- SwiftUI - 动态列表
- SwiftUI - 自定义列表
- SwiftUI - 表格
- SwiftUI - 表单
- SwiftUI - 表单
- SwiftUI - 将表单分成多个部分
- SwiftUI 有用资源
- SwiftUI - 有用资源
- SwiftUI - 讨论
SwiftUI - 绘制饼图
饼图是一种圆形图表,用于以圆形形式显示数据。此图表被分成多个扇区,每个扇区代表一类数据,扇区的大小代表该类数据的数量或百分比。饼图通常用于以非常简单的形式显示数据,以便用户可以轻松比较整个图表中的不同部分。饼图包含以下组件:
- 扇区:它代表一类数据。
- 标签:它用百分比值和类别名称标记每个扇区。
- 图例:它解释每个扇区代表什么。
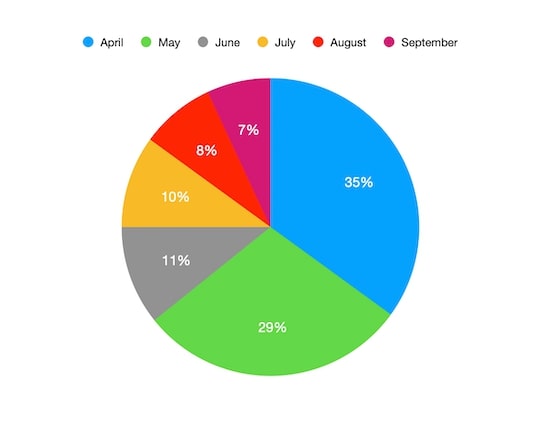
SwiftUI 没有提供任何直接创建饼图的内置方法。因此,我们将使用addArc()方法和Zstack创建饼图。其中 Zstack 用于创建不同颜色的扇区,addArc() 方法用于根据指定的半径和角度计算起始点和结束点,然后它将在这些点之间创建曲线,然后将曲线插入路径以创建所需的形状。
语法
以下是语法:
func addArc(center: CGPoint, radius: CGFloat, startAngle: Angle, endAngle: Angle, clockwise: Bool, transform: CGAffineTransform = .identity)
参数
此函数采用以下参数:
- center: 此参数的坐标表示圆弧的中心。
- radius: 此参数的坐标表示圆弧的半径。
- startAngle: 它表示圆弧起点的角度。它从正 x 轴计算。
- endAngle: 它表示圆弧终点的角度。它从正 x 轴计算。
- clockwise: 如果此参数的值设置为 true,则圆弧将顺时针创建。否则,圆弧将逆时针创建。
- transform: 它用于在添加到路径之前将变换应用于圆弧。默认情况下,它设置为恒等变换。
绘制饼图
现在我们将开始绘制饼图。为此,我们将使用以下问题陈述。假设 Mohan 对 100 人进行了关于最喜欢的蔬菜的调查,结果如下:
- 土豆:40%
- 豌豆:30%
- 西红柿:20%
- 青椒:10%
现在我们将创建此调查的饼图。根据数据,饼图将包含四个不同颜色的四个扇区,每种颜色代表一种蔬菜,例如土豆代表橙色,豌豆代表绿色,西红柿代表红色,青椒代表蓝色。因此,以下 SwiftUI 程序将使用 addArch() 方法和 Zstack 创建饼图。
import SwiftUI struct ContentView: View { var body: some View { ZStack { Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: CGPoint(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: -90.0), endAngle: Angle(degrees: 54.0), clockwise: false) }.fill(Color.orange) Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: CGPoint(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 54.0), endAngle: Angle(degrees: 162.0), clockwise: false) }.fill(Color.green) Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: CGPoint(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 162.0), endAngle: Angle(degrees: 234.0), clockwise: false) }.fill(Color.blue) Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: CGPoint(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 234.0), endAngle: Angle(degrees: 270.0), clockwise: false) }.fill(Color.red) } } } #Preview { ContentView() }
输出
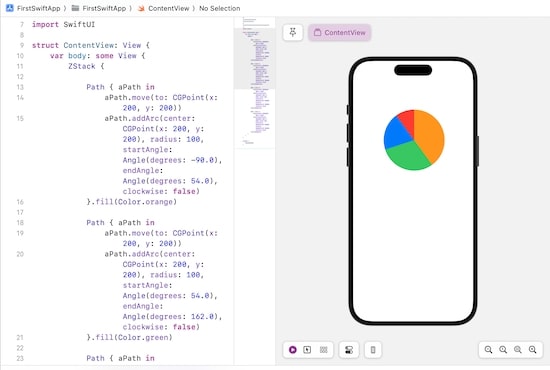
我们可以自定义饼图以使其更具吸引力。因此,我们在每个扇区上添加边框和阴影。还在扇区中添加标签。
import SwiftUI struct ContentView: View { var body: some View { ZStack { Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: -90), endAngle: Angle(degrees: 54), clockwise: false) }.fill(Color.orange).overlay( Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: -90), endAngle: Angle(degrees: 54), clockwise: false) }.stroke(Color.black, lineWidth: 2).overlay(Text("40%") .bold().foregroundColor(.white).offset(x: 50, y: -190))) Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 54), endAngle: Angle(degrees: 162), clockwise: false) }.fill(Color.green).overlay( Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 54), endAngle: Angle(degrees: 162), clockwise: false) }.stroke(Color.black, lineWidth: 2) ) Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 162), endAngle: Angle(degrees: 234), clockwise: false) } .fill(Color.blue) .overlay( Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 162), endAngle: Angle(degrees: 234), clockwise: false) }.stroke(Color.black, lineWidth: 2)) Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 234), endAngle: Angle(degrees: 270), clockwise: false) }.fill(Color.red).overlay( Path { aPath in aPath.move(to: CGPoint(x: 200, y: 200)) aPath.addArc(center: .init(x: 200, y: 200), radius: 100, startAngle: Angle(degrees: 234), endAngle: Angle(degrees: 270), clockwise: false) }.stroke(Color.black, lineWidth: 2)) }.shadow(radius: 10) } } #Preview { ContentView() }
输出
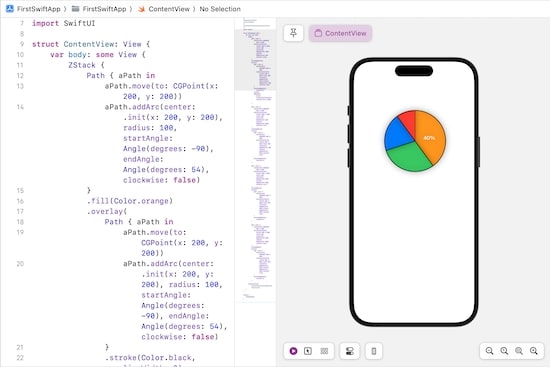
广告