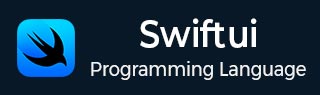
- SwiftUI 教程
- SwiftUI - 首页
- SwiftUI - 概述
- SwiftUI vs UIKit
- SwiftUI 环境
- SwiftUI - 环境设置
- SwiftUI - 基本组件
- SwiftUI - 创建第一个应用程序
- SwiftUI 视图
- SwiftUI - 视图
- SwiftUI - 自定义文本视图
- SwiftUI - 自定义图像视图
- SwiftUI - 堆栈
- SwiftUI 绘制形状
- SwiftUI - 形状
- SwiftUI - 绘制线条
- SwiftUI - 绘制矩形
- SwiftUI - 绘制圆角矩形
- SwiftUI - 绘制三角形
- SwiftUI - 绘制圆形
- SwiftUI - 绘制星形
- SwiftUI - 绘制多边形
- SwiftUI - 绘制饼图
- SwiftUI - 使用内置形状
- SwiftUI - 文本
- SwiftUI - 文本视图
- SwiftUI - 文本输入和输出
- SwiftUI - 颜色
- SwiftUI - 颜色
- SwiftUI - 颜色选择器
- SwiftUI - 渐变
- SwiftUI - 调整颜色
- SwiftUI - 效果
- SwiftUI - 效果
- SwiftUI - 混合效果
- SwiftUI - 模糊效果
- SwiftUI - 阴影效果
- SwiftUI - 悬停效果
- SwiftUI - 动画
- SwiftUI - 动画
- SwiftUI - 创建动画
- SwiftUI - 创建显式动画
- SwiftUI - 多个动画
- SwiftUI - 转场
- SwiftUI - 非对称转场
- SwiftUI - 自定义转场
- SwiftUI - 图片
- SwiftUI - 图片
- SwiftUI - 图片作为背景
- SwiftUI - 旋转图片
- SwiftUI - 媒体
- SwiftUI - 视图布局
- SwiftUI - 视图布局
- SwiftUI - 视图大小
- SwiftUI - 视图间距
- SwiftUI - 视图填充
- SwiftUI - 列表和表格
- SwiftUI - 列表
- SwiftUI - 静态列表
- SwiftUI - 动态列表
- SwiftUI - 自定义列表
- SwiftUI - 表格
- SwiftUI - 表单
- SwiftUI - 表单
- SwiftUI - 将表单分成多个部分
- SwiftUI 有用资源
- SwiftUI - 有用资源
- SwiftUI - 讨论
SwiftUI - 表格
SwiftUI 对表格提供了良好的支持。表格视图用于以表格形式显示数据。它可以创建简单和复杂的表格布局。它允许我们以结构化和多列布局显示数据。
我们还可以自定义表格及其数据,例如标题、表格内容的样式、字体、背景颜色、每列的格式等。日历、产品比较等是 UI 中表格的一些现实生活示例。因此,在本节中,我们将介绍如何创建表格、如何设置样式、如何排序表格数据等等。
在 SwiftUI 中创建表格
在 SwiftUI 中,我们可以借助表格视图创建表格。表格视图以行和列的格式显示数据。它根据可识别的可识别数据集合计算其行。Identifiable 是一个类,其实例持有具有有效身份的实体的值。表格支持水平和垂直滚动,以便在行和列的数量超过视图宽度时调整表格的视图。
语法
以下是语法:
Table(IdentifiableData){ TableColumn(Text, value: \.IdentifiableProperty1) TableColumn(Text, value: \.IdentifiableProperty1 }
示例
以下 SwiftUI 程序用于创建一个简单的表格,其中存储了学生姓名及其喜欢的科目。
import SwiftUI struct Student: Identifiable{ let name: String let id = UUID() let subject: String } struct ContentView: View { @State private var stud = [ Student(name: "Mohina", subject: "Maths"), Student(name: "Rohita", subject: "Science"), Student(name: "Soman", subject: "Maths"), Student(name: "Soha", subject: "Science"), ] var body: some View { VStack{ Text("Class = 1 Data ").font(.title2) // Creating table Table(stud){ TableColumn("Student Names", value: \.name) TableColumn("Favourite Subject", value: \.subject) } } } } #Preview { ContentView() }
输出
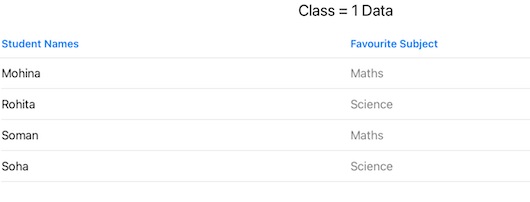
在 SwiftUI 中向表格添加选择功能
我们还可以通过创建 Set 类型的绑定选择变量来选择表格的一行或多行。要创建一个表格数据的单个可选择实例,我们必须为此表格创建一个 id 类型,然后将该变量传递给表格视图。
示例
以下 SwiftUI 程序用于创建一个可选择的表格。
import SwiftUI struct Student: Identifiable{ let name: String let id = UUID() let age: Int let subject: String } struct ContentView: View { @State private var stud = [ Student(name: "Mohina", age: 19, subject: "Maths"), Student(name: "Rohita", age: 18, subject: "Science"), Student(name: "Soman", age: 10, subject: "Maths"), Student(name: "Soha", age: 17, subject: "Science"), ] @State private var select = Set<Student.ID>() var body: some View { VStack{ Text("Original Table ").font(.title2) // Creating table Table(stud, selection: $select){ TableColumn("Student Names", value: \.name) TableColumn("Age"){ stud in Text(String(stud.age)) } TableColumn("Favourite Subject", value: \.subject) } } } } #Preview { ContentView() }
输出
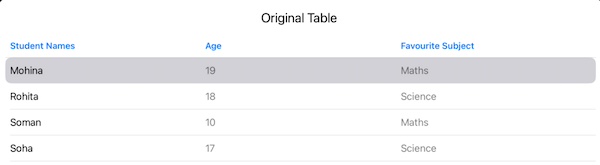
在 SwiftUI 中设置表格样式
在 SwiftUI 中,我们可以使用 `tableStyle()` 修饰符根据设计需要设置表格样式。`.tableStyle()` 修饰符为我们提供了一些预定义的表格样式,使用这些样式可以增强简单表格的外观。
语法
以下是语法:
func tableStyle(_ style: S) -> some View where S : TableStyle
它只接受一个参数,即样式。此参数的值可以是以下任何一个:
.automatic:用于将默认系统样式应用于表格。
inset:用于将内嵌样式应用于表格,这使得其内容从边缘缩进。
示例
以下 SwiftUI 程序用于以内嵌格式设置表格样式。
import SwiftUI struct Student: Identifiable{ let name: String let id = UUID() let age: Int let subject: String } struct ContentView: View { @State private var stud = [ Student(name: "Mohina", age: 19, subject: "Maths"), Student(name: "Rohita", age: 18, subject: "Science"), Student(name: "Soman", age: 10, subject: "Maths"), Student(name: "Soha", age: 17, subject: "Science"), ] var body: some View { VStack{ Text("Original Table ").font(.title2) // Creating table Table(stud){ TableColumn("Student Names", value: \.name) TableColumn("Age"){ stud in Text(String(stud.age)) } TableColumn("Favorite Subject", value: \.subject) }.tableStyle(.inset) } } } #Preview { ContentView() }
输出
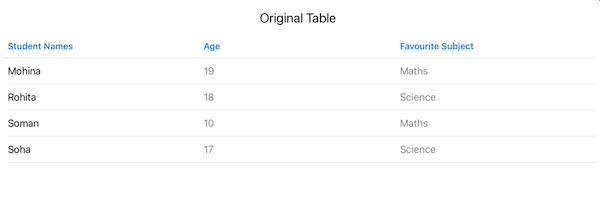
广告