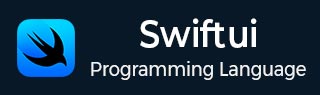
- SwiftUI 教程
- SwiftUI - 首页
- SwiftUI - 概述
- SwiftUI vs UIkit
- SwiftUI 环境
- SwiftUI - 环境设置
- SwiftUI - 基本组件
- SwiftUI - 构建第一个应用程序
- SwiftUI 视图
- SwiftUI - 视图
- SwiftUI - 自定义文本视图
- SwiftUI - 自定义图像视图
- SwiftUI - 堆叠
- SwiftUI 绘制形状
- SwiftUI - 形状
- SwiftUI - 绘制线条
- SwiftUI - 绘制矩形
- SwiftUI - 绘制圆角矩形
- SwiftUI - 绘制三角形
- SwiftUI - 绘制圆形
- SwiftUI - 绘制星形
- SwiftUI - 绘制多边形
- SwiftUI - 绘制饼图
- SwiftUI - 使用内置形状
- SwiftUI - 文本
- SwiftUI - 文本视图
- SwiftUI - 文本输入和输出
- SwiftUI - 颜色
- SwiftUI - 颜色
- SwiftUI - 颜色选择器
- SwiftUI - 渐变
- SwiftUI - 调整颜色
- SwiftUI - 效果
- SwiftUI - 效果
- SwiftUI - 混合效果
- SwiftUI - 模糊效果
- SwiftUI - 阴影效果
- SwiftUI - 悬停效果
- SwiftUI - 动画
- SwiftUI - 动画
- SwiftUI - 创建动画
- SwiftUI - 创建显式动画
- SwiftUI - 多个动画
- SwiftUI - 过渡
- SwiftUI - 非对称过渡
- SwiftUI - 自定义过渡
- SwiftUI - 图像
- SwiftUI - 图像
- SwiftUI - 图像作为背景
- SwiftUI - 旋转图像
- SwiftUI - 媒体
- SwiftUI - 视图布局
- SwiftUI - 视图布局
- SwiftUI - 视图大小
- SwiftUI - 视图间距
- SwiftUI - 视图填充
- SwiftUI - 列表和表格
- SwiftUI - 列表
- SwiftUI - 静态列表
- SwiftUI - 动态列表
- SwiftUI - 自定义列表
- SwiftUI - 表格
- SwiftUI - 表单
- SwiftUI - 表单
- SwiftUI - 将表单拆分为多个部分
- SwiftUI 有用资源
- SwiftUI - 有用资源
- SwiftUI - 讨论
SwiftUI - 绘制圆角矩形
圆角矩形是一个矩形,但具有圆角。此形状通过定义其角的曲率半径来创建。在 UI 中,圆角矩形用于设计字段、按钮、图标等,因为与矩形相比,它在视觉上更具吸引力。SwiftUI 提供了一个内置函数来创建圆角矩形,但在这里我们将创建一个没有任何内置函数的圆角矩形。
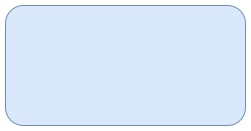
在 SwiftUI 中绘制圆角矩形
在 SwiftUI 中,我们可以使用 addLine(to:) 方法创建线段和线段。此方法从当前点到给定点(终点)添加一条直线段。此函数采用两个坐标 (CGPoint(x:, y:)),它们是当前点和线段终点的长度,线段将在此处结束。
语法
以下是语法 -
addLine(to end: CGPoint)
此函数仅接受一个参数“end”。它表示线段终点在用户空间坐标系中的位置。
在 SwiftUI 中绘制圆角矩形的步骤
按照以下步骤在 SwiftUI 中绘制圆角矩形 -
步骤 1:定义自定义形状
要绘制自定义圆角矩形,我们需要创建一个符合 Shape 协议的结构体。在此结构体中,我们将定义如何创建圆角矩形。或者我们可以说定义 path(in:) 方法
struct RoundedRectangle: Shape { var cRadius: CGFloat var corners: UIRectCorner func path(in rRect: CGRect) -> Path { let xpath = UIBezierPath( roundedRect: rRect, byRoundingCorners: corners, cornerRadii: CGSize(width: cRadius, height: cRadius) ) return Path(xpath.cgPath) } }
步骤 2:创建视图
现在我们在 SwiftUI 视图中使用自定义圆角矩形。它将显示自定义圆角矩形的外观。
struct ContentView: View { var body: some View { RoundedRectangle(cRadius: 30, corners: [.topLeft, .topRight, .bottomLeft, .bottomRight]) } }
步骤 3:添加其他自定义
我们还可以包含其他自定义,例如描边、填充、阴影、叠加文本等,在圆角矩形中。
struct ContentView: View { var body: some View { RoundedRectangle(cRadius: 30, corners: [.topLeft, .topRight, .bottomLeft, .bottomRight]) .fill(Color.blue) .frame(width: 200, height: 100) .padding() } }
示例 1
以下 SwiftUI 程序用于创建实心的自定义圆角矩形。
import SwiftUI struct RoundedRectangle: Shape { var cRadius: CGFloat var corners: UIRectCorner func path(in rRect: CGRect) -> Path { let xpath = UIBezierPath( roundedRect: rRect, byRoundingCorners: corners, cornerRadii: CGSize(width: cRadius, height: cRadius) ) return Path(xpath.cgPath) } } struct ContentView: View { var body: some View { RoundedRectangle(cRadius: 30, corners: [.topLeft, .topRight, .bottomLeft, .bottomRight]) .fill(Color.blue) .frame(width: 200, height: 100) .padding() } } #Preview { ContentView() }
输出
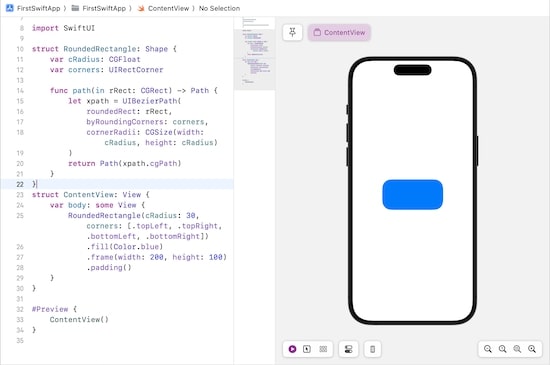
示例 2
以下 SwiftUI 程序用于绘制自定义圆角矩形。在这里,我们将创建圆角矩形的每条线和每个角。
import SwiftUI struct RoundedRectangle: Shape { var topLeftRadius: CGFloat var topRightRadius: CGFloat var bottomLeftRadius: CGFloat var bottomRightRadius: CGFloat func path(in rectangle: CGRect) -> Path { var xpath = Path() // Define the starting point of the rectangle let sPoint = CGPoint(x: rectangle.minX + topLeftRadius, y: rectangle.minY) // Move to the starting point xpath.move(to: sPoint) // Top Right Side xpath.addLine(to: CGPoint(x: rectangle.maxX - topRightRadius, y: rectangle.minY)) xpath.addArc( center: CGPoint(x: rectangle.maxX - topRightRadius, y: rectangle.minY + topRightRadius), radius: topRightRadius, startAngle: Angle(degrees: -90), endAngle: Angle(degrees: 0), clockwise: false ) // Right Bottom Side xpath.addLine(to: CGPoint(x: rectangle.maxX, y: rectangle.maxY - bottomRightRadius)) xpath.addArc( center: CGPoint(x: rectangle.maxX - bottomRightRadius, y: rectangle.maxY - bottomRightRadius), radius: bottomRightRadius, startAngle: Angle(degrees: 0), endAngle: Angle(degrees: 90), clockwise: false ) // Bottom Left Side xpath.addLine(to: CGPoint(x: rectangle.minX + bottomLeftRadius, y: rectangle.maxY)) xpath.addArc(center: CGPoint(x: rectangle.minX + bottomLeftRadius, y: rectangle.maxY - bottomLeftRadius), radius: bottomLeftRadius, startAngle: Angle(degrees: 90), endAngle: Angle(degrees: 180), clockwise: false) // Top Left side xpath.addLine(to: CGPoint(x: rectangle.minX, y: rectangle.minY + topLeftRadius)) xpath.addArc(center: CGPoint(x: rectangle.minX + topLeftRadius, y: rectangle.minY + topLeftRadius), radius: topLeftRadius, startAngle: Angle(degrees: 180), endAngle: Angle(degrees: 270), clockwise: false) return xpath } } struct ContentView: View { var body: some View { RoundedRectangle(topLeftRadius: 20, topRightRadius: 20, bottomLeftRadius: 20, bottomRightRadius: 20) .fill(Color.purple) .frame(width: 300, height: 200) } }
输出
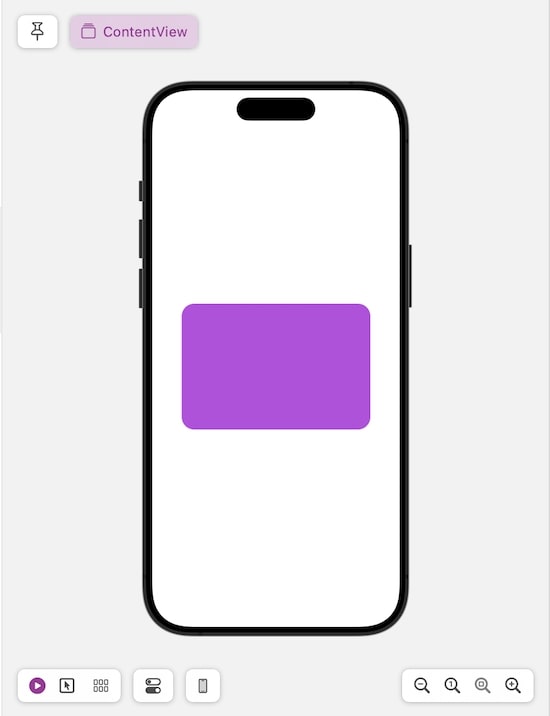
要创建描边圆角矩形,我们将使用 .stroke 修饰符。在这里,我们只会对视图部分进行更改,其余代码与上面相同
struct ContentView: View { var body: some View { RoundedRectangle(topLeftRadius: 20, topRightRadius: 20, bottomLeftRadius: 20, bottomRightRadius: 20) .stroke(Color.red, lineWidth: 3) .frame(width: 300, height: 200) } }
输出
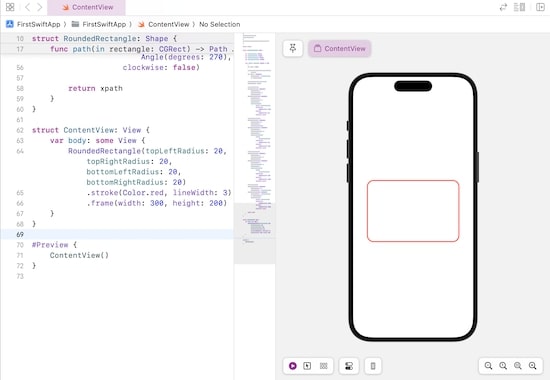
使用以上代码,我们可以根据需要自定义圆角矩形。在这里,我们更改每个角的大小,它将创建一个不均匀的圆角矩形。
struct ContentView: View { var body: some View { RoundedRectangle(topLeftRadius: 50, topRightRadius: 20, bottomLeftRadius: 20, bottomRightRadius: 50) .stroke(Color.red, lineWidth: 3) .frame(width: 300, height: 200) } }
输出
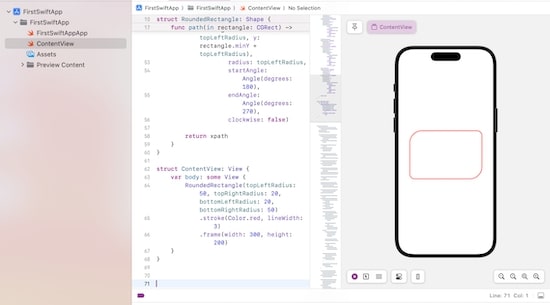
广告