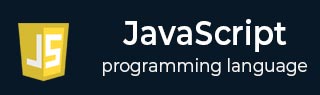
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 放置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - If...Else
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in
- Javascript - For...of
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 带标签的模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promises
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promisification
- JavaScript - Promises 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图像地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - Clickjacking 攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - 数组 .reduce() 方法
在 JavaScript 中,Array.reduce() 方法用于操作数组。此方法对数组中的每个元素(从左到右)执行一个 reducer 函数,并返回一个“单个值”作为结果。
它接受一个名为'initialValue' 的可选参数。如果我们没有将此参数传递给该方法,它将把 arr[0] 的值视为初始值。此外,它将在传递的 initialValue 参数上执行回调函数。
此方法不会对空数组元素执行 reducer 函数。此外,它不会修改原始数组。
注意 - 如果当前数组为空或不包含任何 initialValue,此方法将抛出'TypeError' 异常。
语法
以下是 JavaScript Array.reduce() 方法的语法 -
reduce(callbackFn(accumulator, currentValue, currentIndex, array), initialValue)
参数
此方法接受两个参数。下面描述了相同的内容 -
- callbackFn - 这是要在数组中的每个元素上执行的函数。此函数接受四个参数
- accumulator - 这是 initialValue,或函数先前返回的值。
- currentValue - 这是数组中正在处理的当前元素。如果指定了 initialValue,则其值为 arr[0],否则其值为 arr[1]。
- currentIndex (可选) - 这是数组中正在处理的当前元素的索引。
- array (可选) - 这是调用 reduce() 方法的数组。
- initialValue (可选) - 第一次调用回调函数时,累加器参数初始化到的值。
返回值
此方法返回单个值,该值是在减少数组后得到的结果。
示例
示例 1
在以下示例中,我们使用 JavaScript Array.reduce() 方法来对提供的数组中存在的所有元素求和。
<html> <body> <script> const numbers = [10, 20, 30, 40, 50]; const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0); document.write(sum); </script> </body> </html>
累加器从 0 开始,对于数组中的每个元素,它将当前元素添加到累加器中。累加器的最终结果 (150) 是所有元素的总和。
输出
150
示例 2
在此示例中,我们计算指定数组中所有元素的乘积 -
<html> <body> <script> const numbers = [10, 20, 30, 40, 50]; const product = numbers.reduce((accumulator, currentValue) => accumulator * currentValue, 1); document.write(product); </script> </body> </html>
累加器从 1 开始,对于数组中的每个元素,它将当前元素乘以累加器。累加器的最终结果 (12000000) 是所有元素的乘积。
输出
12000000
示例 3
在下面的示例中,我们将数组的数组 (nesetedArray) 扁平化成一个单一的、一维数组 (flattenedArray) -
<html> <body> <script> const nestedArray = [[1, 2], [3, 4], [5, 6]]; const flattenedArray = nestedArray.reduce((accumulator, currentValue) => accumulator.concat(currentValue), []); document.write(flattenedArray); </script> </body> </html>
输出
1,2,3,4,5,6
示例 4
如果当前数组不包含任何元素(没有可用的初始值),则 reduce() 方法将抛出“TypeError”异常 -
<html> <body> <script> const numbers = []; try { numbers.reduce((accumulator, currentValue) => accumulator * currentValue); } catch (error) { document.write(error); } </script> </body> </html>
输出
TypeError: Reduce of empty array with no initial value
广告