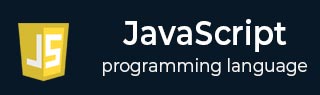
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else
- JavaScript - while 循环
- JavaScript - for 循环
- JavaScript - for...in
- Javascript - for...of
- JavaScript - 循环控制
- JavaScript - break 语句
- JavaScript - continue 语句
- JavaScript - switch case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - String
- JavaScript - Array
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Set
- JavaScript - WeakSet
- JavaScript - Map
- JavaScript - WeakMap
- JavaScript - Iterable
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标签模板
- 面向对象JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理 (Proxies)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 修改 HTML
- JavaScript - 修改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图片地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - ES5
JavaScript 的 ES6 版本于 2015 年发布,标志着 JavaScript 的第二次重大修订。ES6 也被称为 ECMAScript 2015。ES6 中引入的一些重要特性包括箭头函数、let 和 const 关键字、类、rest 参数等。本章将讨论 ES6 版本中所有新增的特性。
ES6 新增特性
以下是添加到 ES6 版本 JavaScript 中的新方法、特性等。
- 箭头函数
- Array find()
- Array findIndex()
- Array from()
- Array keys()
- 类
- const 关键字
- 默认参数
- For/of
- 函数 rest 参数
- JavaScript 模块
- let 关键字
- Map 对象
- 新的全局方法
- 新的 Math 方法
- 新的 Number 方法
- 新的 Number 属性
- Promise
- Set 对象
- String.endsWith()
- String.includes()
- String.startsWith()
- Symbol
- 展开运算符
这里,我们详细解释了每个特性并附带示例。
JavaScript 箭头函数
箭头函数是一种编写更简洁函数代码的方式。箭头函数的概念允许您在不使用 function 关键字、大括号和 return 关键字的情况下定义函数。
示例
在下面的代码中,func() 是一个常规函数,变量 subtracts 存储箭头函数。
<html> <body> <div id = "output">The subtraction of 20 and 10 is: </div> <script> /* Normal function function func(a, b) { return a - b; } */ // Arrow function const subtract = (a, b) => a - b; document.getElementById("output").innerHTML += subtract(20, 10); </script> </body> </html>
输出
The subtraction of 20 and 10 is: 10
JavaScript Array find() 方法
JavaScript array.find() 方法返回第一个符合特定条件的元素。
示例
在下面的代码中,我们使用 array.find() 方法查找长度小于 4 的第一个数组元素。
<html> <body> <div id = "output">The first array element whose length is less than 4 is: </div> <script> const strs = ["Hello", "World", "How", "are", "You"]; function func_len(value, index, array) { return value.length < 4; } document.getElementById("output").innerHTML += strs.find(func_len); </script> </body> </html>
输出
The first array element whose length is less than 4 is: How
JavaScript Array findIndex()
JavaScript array.findIndex() 方法类似于 array.find() 方法,但它返回第一个匹配特定条件的元素的索引。它返回基于 0 的索引。
示例
在下面的代码中,我们使用 array.findIndex() 方法查找长度小于 4 的第一个元素的索引。
<html> <body> <div id = "output">The first array element whose length is less than 4 is: </div> <script> const strs = ["Hello", "World", "How", "are", "You"]; function func_len(value, index, array) { return value.length < 4; } document.getElementById("output").innerHTML += strs.findIndex(func_len); </script> </body> </html>
输出
The first array element whose length is less than 4 is: 2
JavaScript Array from()
JavaScript Array.from() 方法根据作为参数传递的迭代器创建一个数组。
示例
在下面的代码中,我们使用 Array.from() 方法从字符串创建一个数组。但是,您也可以将迭代器作为 Array.from() 方法的参数传递。
<html> <body> <div id = "output">The array from the Hello string is: </div> <script> document.getElementById("output").innerHTML += Array.from("Hello"); </script> </body> </html>
输出
The array from the Hello string is: H,e,l,l,o
JavaScript Array keys()
JavaScript array.keys() 方法返回一个迭代器来迭代键。数组元素的键是数组元素的索引。
示例
在下面的代码中,我们使用 keys() 方法获取 nums[] 数组的键的迭代器。之后,我们使用 for/of 循环遍历数组的键。
<html> <body> <div id = "demo">The keys of the nums array is: <br></div> <script> const output = document.getElementById("demo"); const nums = [45, 67, 89, 342, 123, 12]; const iteratorNums = nums.keys(); for (let key of iteratorNums) { output.innerHTML += key + "<br>"; } </script> </body> </html>
输出
The keys of the nums array is: 0 1 2 3 4 5
JavaScript 类
类在面向对象编程语言中至关重要。它是对象的蓝图。
您可以使用 class 关键字来定义类。您可以向类体中添加构造函数、属性和方法。要访问类属性和方法,您可以使用类实例。
示例
在下面的代码中,我们定义了 animal 类。
构造函数初始化 name 和 isVegetarian 属性的值。getInfo() 方法返回动物信息。
我们创建了动物类的对象,并用它来调用该类的getInfo()方法。
<html> <body> <div id = "output">The animal information is: </div> <script> class animal { constructor(name, isVegetarian) { this.name = name; this.isVegetarian = isVegetarian; } getInfo() { return "Name : " + this.name + ", " + "isVegetarian? : " + this.isVegetarian; } } const lion = new animal("Lion", false); document.getElementById("output").innerHTML += lion.getInfo(); </script> </body> </html>
输出
The animal information is: Name : Lion, isVegetarian? : false
JavaScript const关键字
JavaScript const关键字用于声明常量变量。声明常量变量时需要对其进行初始化。
示例
在下面的代码中,“fruit”是一个常量变量。你不能重新初始化它的值。
<html> <body> <div id = "output">The value of the fruit variable is: </div> <script> const fruit = "Apple"; // fruit = "Banana"; This is Invalid document.getElementById("output").innerHTML += fruit; </script> </body> </html>
输出
The value of the fruit variable is: Apple
JavaScript let关键字
JavaScript let关键字用于定义块作用域变量。
示例
在下面的代码中,我们在“if”块内使用let关键字定义了变量“a”。由于其作用域特性,它无法在“if”块外部访问。
<html> <body> <div id = "output"> </div> <script> if (true) { let a = 20; document.getElementById("output").innerHTML += "The value of a is: " + a; } // You can't access it here. </script> </body> </html>
输出
The value of a is: 20
JavaScript默认参数
默认参数意味着函数参数可以具有默认值。当你没有向函数传递足够的参数时,它将使用默认参数值。
示例
在下面的代码中,division()函数接受两个参数。a的默认值为10,b的默认值为2。
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); function division(a = 10, b = 2) { return a / b; } output.innerHTML += "division(40, 5) => " + division(40, 5) + "<br>"; output.innerHTML += "division(40) => " + division(40) + "<br>"; output.innerHTML += "division() => " + division(); </script> </body> </html>
输出
division(40, 5) => 8 division(40) => 20 division() => 5
JavaScript for…of循环
JavaScript for…of循环遍历可迭代对象,例如数组、字符串、集合、映射等。
示例
在下面的代码中,我们遍历数字数组并在输出中打印数组的每个元素。
<html> <body> <div id = "output">The array elements are: </div> <script> const array = [10, 67, 82, 75, 80]; for (let number of array) { document.getElementById("output").innerHTML += number + ", "; } </script> </body> </html>
输出
The array elements are: 10, 67, 82, 75, 80,
JavaScript函数剩余参数
当你不确定函数参数的数量时,可以使用剩余参数。剩余参数允许你将多个参数收集到单个数组中。
示例
我们在下面的代码中使用剩余参数和sum()函数。剩余参数的名称可以是有效的标识符,它与扩展运算符(…)一起使用。
sum()函数将多个数值相加并返回结果。
<html> <body> <div id = "output">sum(10, 67, 82, 75, 80) = </div> <script> function sum(...restParam) { let res = 0; for (let ele of restParam) { res += ele; } return res; } document.getElementById("output").innerHTML += sum(10, 67, 82, 75, 80); </script> </body> </html>
输出
sum(10, 67, 82, 75, 80) = 314
JavaScript 模块
在JavaScript中,你可以创建不同的模块来编写可重用的代码。之后,你可以将这些模块导入到不同的JavaScript文件中。
默认导出/导入模块
const moduleMath = "This is the default value."; export default moduleMath; // Exporting the module
在其他JavaScript文件中,
// Importing the default module import moduleMath from './filename.js'; console.log(moduleMath);
命名导出/导入模块
你还可以从模块中导出特定的函数或属性,并将它们导入到其他JavaScript文件中。
// Exporting variables export const size = 90; // Exporting function export function add(a, b) { return a + b; }
在其他JavaScript文件中,
// Importing specific properties and functions import { size, add} from './filename.js'; console.log(myVariable); // 90 console.log(add(15, 25)); // 40
JavaScript Map对象
Map用于存储键值对。你可以使用Map()构造函数来定义一个Map。
示例
在下面的示例中,我们使用Map来存储水果的名称和价格。set()方法用于将键值对插入到fruit Map中,get()方法用于从Map中获取特定键的值。
<html> <body> <div id = "output1">The price of the Apple is: </div> <div id = "output2">The price of the Banana is: </div> <script> const fruit = new Map(); fruit.set("Apple", 50); fruit.set("Banana", 60); document.getElementById("output1").innerHTML += fruit.get("Apple") + "<br>"; document.getElementById("output2").innerHTML += fruit.get("Banana"); </script> </body> </html>
输出
The price of the Apple is: 50 The price of the Banana is: 60
新的全局方法
在ES6中,添加了以下两个全局方法。
- isFinite()
- isNaN()
isFinite()
isFinite()方法检查作为参数传递的值是否为有限值。
示例
在下面的代码中,num1变量包含无限值,num2包含有效数值。
我们使用isFinite()方法来检查num1和num2变量的值是否为有限值。
<html> <body> <div id = "output"> </div> <script> const num1 = 6453 / 0; const num2 = 90; document.getElementById("output").innerHTML = "isFinite(6453 / 0): " + isFinite(num1) + "<br>" + "isFinite(90): " + isFinite(num2); </script> </body> </html>
输出
isFinite(6453 / 0): false isFinite(90): true
isNaN()
isNaN()方法检查参数是否为有效数字。对于数字值,它返回false。
示例
在下面的代码中,isNaN()方法对于num1变量返回true,因为它包含字符串,而字符串不是数字。对于num2变量,isNaN()方法返回false,因为它包含数值。
<html> <body> <div id = "output"> </div> <script> const num1 = "Hello"; const num2 = 867; document.getElementById("output").innerHTML = "isNaN(num1): " + isNaN(num1) + "<br>" + "isNaN(num2): " + isNaN(num2); </script> </body> </html>
输出
isNaN(num1): true isNaN(num2): false
新的JavaScript Math方法
在ES6中,向Math对象添加了5个新方法。
- Math.cbrt() − 用于查找给定数字的立方根。
- Math.log2() – 用于查找数字的对数,并使用以2为底。
- Math.log10() – 查找数值以10为底的对数。
- Math.trunc() – 从浮点数中移除小数部分,并将其转换为整数。
- Math.sign() – 根据作为参数传递的数字的符号返回1、0和-1。
示例:Math.cbrt()
下面的代码查找64的立方根。
<html> <body> <div id = "output">The cube root of the 64 is: </div> <script> document.getElementById("output").innerHTML += Math.cbrt(64); </script> </body> </html>
示例:Math.log2()
下面的代码查找以2为底的30的对数。
<html> <body> <div id = "output">The value of logarithm of 30 base 2 is: </div> <script> document.getElementById("output").innerHTML += Math.log2(30); </script> </body> </html>
示例:Math.log10()
下面的代码查找以10为底的10的对数。
<html> <body> <div id = "output">The value of the logarithm of 10 base 10 is: </div> <script> document.getElementById("output").innerHTML += Math.log10(10); </script> </body> </html>
示例:Math.trunc()
下面的代码使用Math.trunc()方法截断浮点数。
<html> <body> <div id = "output">After converting 23.2 to integer is: </div> <script> document.getElementById("output").innerHTML += Math.trunc(23.2); </script> </body> </html>
示例:Math.sign()
<html> <body> <div id="output1">Math.sign(23): </div> <div id="output2">Math.sign(-23): </div> <script> document.getElementById("output1").innerHTML += Math.sign(23); document.getElementById("output2").innerHTML += Math.sign(-23); </script> </body> </html>
新的 Number 方法
在ES6中,添加了两个新的数字方法。
Number.isInteger() − 检查作为参数传递的数字是否为整数。
Number.isSafeInteger() − 检查该数字是否可以表示为64位双精度数。
示例
下面的代码检查10和10.5是否为整数。此外,它还使用Number类的isSafeInteger()方法来检查该数字是否为安全整数。
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); output.innerHTML += "Is 10 integer? " + Number.isInteger(10) + "<br>"; output.innerHTML += "Is 10.5 integer? " + Number.isInteger(10.5) + "<br>"; output.innerHTML += "Is 10000 safe integer? " + Number.isSafeInteger(10000) + "<br>"; output.innerHTML += "Is 10000000000000000000000 safe integer? " + Number.isSafeInteger(10000000000000000000000); </script> </body> </html>
输出
Is 10 integer? true Is 10.5 integer? false Is 10000 safe integer? - true Is 10000000000000000000000 safe integer? - false
新的 Number 属性
在ES6中,添加了三个新的数字属性。
EPSILON − 返回Epsilon的值。
MIN_SAFE_INTEGER − 返回64位数可以表示的最小整数值。
MAX_SAFE_INTEGER − 返回64位可以表示的最大数。
示例
下面的代码显示了JavaScript中Epsilon常数的值、安全整数的最小值和安全整数的最大值。
<html> <body> <div id = "output1">The value of Epsilon is: </div> <div id = "output2">The minimum safe integer is: </div> <div id = "output3">The maximum safe integer is: </div> <script> document.getElementById("output1").innerHTML += Number.EPSILON; document.getElementById("output2").innerHTML += Number.MIN_SAFE_INTEGER; document.getElementById("output3").innerHTML += Number.MAX_SAFE_INTEGER </script> </body> </html>
输出
The value of Epsilon is: 2.220446049250313e-16 The minimum safe integer is: -9007199254740991 The maximum safe integer is: 9007199254740991
JavaScript Promise
在JavaScript中,Promise用于异步处理代码。
它产生和使用代码。
示例
在下面的代码中,我们使用Promise()构造函数创建一个Promise。我们根据使用Math块的random()方法生成的随机值来解决和拒绝Promise。
之后,我们使用then()和catch()块来处理Promise。
<html> <body> <div id = "output"> </div> <script> // Creating a Promise const newPromise = new Promise((res, rej) => { setTimeout(() => { const rand_value = Math.random(); if (rand_value < 0.5) { res("Value is less than 0.5"); // Resolving the promise } else { rej("Value is greater than 0.5"); // Rejecting the promise } }, 1000); // Adding 1 second delay }); // Consuming the Promise newPromise .then((res) => { document.getElementById("output").innerHTML += res; }) .catch((rej) => { document.getElementById("output").innerHTML += rej; }); </script> </body> </html>
输出
Value is greater than 0.5
JavaScript Set对象
Set()构造函数用于创建一个Set。Set只存储不同类型对象的唯一元素。
示例
在下面的代码中,我们创建了一个新的Set,并将包含数字的数组作为Set()构造函数的参数传递。Set只包含唯一元素,你可以在输出中看到这一点。
<html> <body> <div id = "output">The set elements are: </div> <script> const num_set = new Set([10, 20, 20, 42, 12]); for (let num of num_set) { document.getElementById("output").innerHTML += ", " + num; } </script> </body> </html>
输出
The set elements are: , 10, 20, 42, 12
JavaScript新的字符串方法
在ES6中,添加了三个新的字符串方法。
- endsWith() − 检查字符串是否以特定子字符串结尾。
- includes() − 检查字符串是否在任何位置包含子字符串。
- startsWith() − 检查字符串是否以特定子字符串开头。
示例
下面的示例演示了如何使用字符串endsWith()、includes()和startsWith()方法以及字符串“How are you? I'm fine!”。
<html> <body> <div id = "output1">Does string end with 'fine'? </div> <div id = "output2">Does string include 'are'? </div> <div id = "output3">Does string start with 'How'? </div> <script> let str = "How are you? I'm fine!"; document.getElementById("output1").innerHTML += str.endsWith("fine!"); document.getElementById("output2").innerHTML += str.includes("are"); document.getElementById("output3").innerHTML += str.startsWith("How"); </script> </body> </html>
输出
Does string end with 'fine'? true Does string include 'are'? true Does string start with 'How'? true
JavaScript Symbol
JavaScript Symbol是JavaScript中的原始数据类型。在JavaScript中,每个Symbol都是唯一的。你可以使用它来创建唯一的ID。
示例
在下面的代码中,我们定义了两个Symbol,并传递了相同的值作为参数。尽管如此,这两个Symbol仍然是唯一的,你可以在输出中看到这一点。
<html> <body> <div id = "output"> </div> <script> const sym1 = Symbol("a"); const sym2 = Symbol("a"); if (sym1 == sym2) { document.getElementById("output").innerHTML += "sym1 and sym2 are equal. <br>"; } else { document.getElementById("output").innerHTML += "sym1 and sym2 are not equal."; } </script> </body> </html>
输出
sym1 and sym2 are not equal.
JavaScript扩展运算符
JavaScript扩展运算符允许你创建一个可迭代对象的副本,例如数组、字符串等。
示例
下面的代码演示了如何使用扩展运算符从字符串创建字符数组。
<html> <body> <div id = "output">The char array is: </div> <script> let str = "Hello World!"; const char = [...str]; document.getElementById("output").innerHTML += char; </script> </body> </html>
输出
The char array is: H,e,l,l,o, ,W,o,r,l,d,!