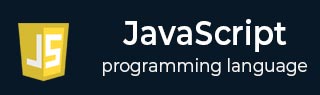
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else
- JavaScript - while 循环
- JavaScript - for 循环
- JavaScript - for...in
- Javascript - for...of
- JavaScript - 循环控制
- JavaScript - break 语句
- JavaScript - continue 语句
- JavaScript - switch case
- JavaScript - 用户自定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - String
- JavaScript - Array
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Set
- JavaScript - WeakSet
- JavaScript - Map
- JavaScript - WeakMap
- JavaScript - Iterable
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标签模板
- 面向对象的 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxy
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步编程
- JavaScript - 异步编程
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promisification
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 修改 HTML
- JavaScript - 修改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图片地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - ECMAScript 2017
JavaScript 的 ECMAScript 2017 版本于 2017 年发布。ECMAScript 2017 为该语言引入了一些新特性。其中最显著的特性是 async/await 语法,它允许我们以更同步的风格编写异步操作。它提供了共享内存和原子操作,增强了对并发编程的支持。
在本章中,我们将讨论 ECMAScript 2017 中添加的所有新特性。
ECMAScript 2017 中添加的新特性
以下是添加到 ECMAScript 2017 版本 JavaScript 中的新方法、特性等。
padStart() 和 padEnd() 方法
Object.entries() 方法
Object.values() 方法
JavaScript async 和 await
Object getOwnPropertyDescriptors() 方法
JavaScript 共享内存
这里,我们详细解释了每个特性。
字符串填充:padStart() 和 padEnd() 方法
ECMAScript 2017 引入了两种字符串方法,padStart() 和 padEnd() 方法,允许您通过添加特定字符来在字符串的开头和结尾添加填充。这些方法用于扩展字符串并达到所需的长度。
示例
在下面的代码中,我们将所需的字符串长度作为 padStart() 和 padEnd() 方法的第一个参数,并将字符作为第二个参数传递。
但是,您也可以将字符串作为第二个参数传递。
<html> <body> <div id = "output1">After padding at the start: </div> <div id = "output2">After padding at the end: </div> <script> let base = "TurorialsPoint"; let start_updated = base.padStart(18, "@"); // Padding at the start let end_updated = base.padEnd(18, "*"); // Padding at the end document.getElementById("output1").innerHTML += start_updated; document.getElementById("output2").innerHTML += end_updated; </script> </body> </html>
输出
After padding at the start: @@@@TurorialsPoint After padding at the end: TurorialsPoint****
Object.entries() 方法
ECMAScript 2017 为对象添加了 Object.entries() 方法。Object.entries() 方法返回一个迭代器,用于遍历对象的键值对。
示例
在下面的代码中,employee 对象包含三个属性。我们使用 Object entries() 方法来获取对象的迭代器。
之后,我们使用 for...of 循环使用迭代器遍历对象属性。
<html> <body> <div id = "demo"> </div> <script> const output = document.getElementById("demo"); const employee = { Company: "TutorialsPoint", Ex_company: "TCS", Experience: 4, } const emp_iterator = Object.entries(employee); for (let pair of emp_iterator) { output.innerHTML += pair + "<br>"; } </script> </body> </html>
输出
Company,TutorialsPoint Ex_company,TCS Experience,4
Object.values() 方法
ECMAScript 2017 为对象引入了 Object.values() 方法。JavaScript Object.values() 方法用于获取对象属性值的数组。
示例
在下面的代码中,我们使用 Object.values() 方法获取数组中所有对象的 value。
<html> <body> <div id = "output">Object values are: </div> <script> const wall = { color: "blue", size: "15x12", paintBrand: "Asiant paints" } document.getElementById("output").innerHTML += " " + Object.values(wall); </script> </body> </html>
输出
Object values are: blue,15x12,Asiant paints
JavaScript async 和 await
async 和 await 关键字在 ECMAScript 2017 中添加到语言中。async/await 关键字用于创建异步函数。async 关键字用于创建异步函数,await 关键字用于处理操作。
示例
在下面的代码中,printMessage() 函数是异步的。我们在函数内部定义了一个新的 promise 并将其存储在 getData 变量中。
承诺需要 0.5 秒才能解析。`await` 关键字处理承诺,并在承诺解析之前阻塞函数代码执行。
<html> <body> <div id = "output"> </div> <script> async function printMessage() { let getData = new Promise(function (res, rej) { setTimeout(() => { res("Promise resolved !!"); }, 500); }); document.getElementById("output").innerHTML = await getData; } printMessage(); </script> </body> </html>
输出
Promise resolved !!
Object.getOwnPropertyDescriptors() 方法
Object.getOwnPropertyDescriptor() 方法返回每个属性的属性描述符,例如 writable、enumerable、configurable、value 等。它是对象属性的元数据。
示例
在下面的代码中,我们使用 getOwnPrpopertyDescriptors() 方法获取对象每个属性的属性描述符。(注意:原文此处拼写错误,应为 getOwnPropertyDescriptors)
<html> <body> <div id = "output">The Property descriptors of the wall object is: <br></div> <script> const wall = { color: "blue", thickness: 10, } document.getElementById("output").innerHTML += JSON.stringify(Object.getOwnPropertyDescriptors(wall)); </script> </body> </html>
输出
The Property descriptors of the wall object is: {"color":{"value":"blue","writable":true,"enumerable":true,"configurable":true},"thickness":{"value":10,"writable":true,"enumerable":true,"configurable":true}}
JavaScript 共享内存和原子操作
在 JavaScript 中,共享内存允许多个线程共享内存,从而实现多个线程之间的高效通信。
JavaScript 是一种单线程编程语言。但是,您可以使用 Web Workers 在不同的线程中运行 JavaScript 代码。
2018 年,引入了 SharedArrayBuffer 来共享内存并通过共享数据执行原子操作。