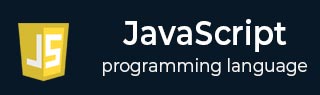
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - 删除运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - 展开运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - If...Else
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in
- Javascript - For...of
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 带标签的模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookies
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图像地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - Clickjacking 攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - typeof 运算符
typeof 运算符
在 JavaScript 中,typeof 运算符是一个一元运算符,用于获取特定变量的数据类型。它放在其单个操作数之前,该操作数可以是任何类型。它返回一个字符串值,指示其操作数的数据类型。JavaScript 包含基本数据类型和非基本数据类型。
JavaScript 中有七种基本数据类型 - 数字、字符串、布尔值、未定义、空、符号和大整数。还有一个称为对象的复合数据类型。对象数据类型包含三个子数据类型 - 对象、数组和日期。
语法
以下是 typeof 运算符的语法:
typeof (operand);
我们可以如下所示编写不带括号的操作数:
typeof operand;
参数
操作数 - 它可以是表示对象或基本类型的值、变量或表达式。在 JavaScript 中,基本类型是不具有方法或属性的对象的数据。
返回值
它返回一个字符串值,表示操作数的数据类型。
typeof 运算符返回的数据类型
以下是typeof运算符的返回值列表。
类型 | typeof 返回的字符串 |
---|---|
数字 | "number" |
字符串 | "string" |
布尔值 | "boolean" |
对象 | "object" |
函数 | "function" |
未定义 | "undefined" |
空 | "object" |
符号 | "symbol" |
大整数 | "bigint" |
JavaScript 中有七种基本数据类型 - 数字、字符串、布尔值、大整数、未定义、空和符号。typeof 运算符用于识别这些基本数据类型。
typeof 运算符返回所有基本类型的值的相同数据类型,除了空值。它为空值返回“object”。
对于对象、日期和数组,它返回“object”作为数据类型。
对于函数和类,它返回“function”作为数据类型。
让我们使用 typeof 运算符逐一识别这些数据类型。
typeof 10; // returns 'number' typeof 'Hello World'; // returns 'string' typeof true; // returns 'boolean' typeof {name:"Tutorialspoint"}; // returns 'object' typeof function foo(){};// returns 'function' typeof undefined; // returns 'undefined' typeof null; // returns 'object' typeof Symbol(); // returns 'symbol' typeof 10n; // returns 'bigint'
JavaScript typeof 运算符检查数字类型
在 JavaScript 中,数字类型表示数值。JavaScript 对所有数字使用浮点表示法。JavaScript typeof 运算符对所有类型的数字(如整数、浮点数、零、Infinity、NaN 等)返回“number”。
typeof 10; //returns "number"; typeof -10; //returns "number"; typeof 0; //returns "number"; typeof 10.20; //returns "number"; typeof Math.LN10; //returns "number"; typeof Infinity; //returns "number"; typeof NaN; //returns "number"; typeof Number('1'); //returns "number"; typeof Number('hello'); //returns "number";
示例
以下示例演示了如何使用 typeof 运算符检查数字数据类型。
<html> <body> <p> Using typeof operator to check number data type </p> <div id="output"></div> <script> let num = 42; document.getElementById("output").innerHTML = typeof num; </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
Using typeof operator to check number data type number Set the variable to different value and then try...
JavaScript typeof 运算符检查字符串类型
字符串表示字符序列。typeof 运算符有助于识别字符串变量。JavaScript 的 typeof 运算符对于所有类型的字符串都返回 "string",例如空字符串、字符字符串、单词字符串、多行字符串等。
typeof "10"; //returns "string"; typeof ""; //returns "string"; typeof "Hello World"; //returns "string"; typeof String(10); //returns "string"; typeof typeof 2; //returns "string";
示例
在下面的示例中,我们使用 typeof 运算符来检查字符串数据类型。
<html> <body> <div id="output"></div> <script> let str = "Hello World"; document.getElementById("output").innerHTML = typeof str; </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
string Set the variable to different value and then try...
JavaScript typeof 运算符检查布尔类型
布尔值表示真或假。tyepof 操作数对布尔变量返回布尔值。
typeof true; //returns "boolean"; typeof false; //returns "boolean"; typeof Boolean(10); //returns "boolean";
示例
在下面的示例中,我们使用 typeof 运算符来检查布尔数据类型。
<html> <body> <div id="output"></div> <script> let bool = true; document.getElementById("output").innerHTML = typeof bool; </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
boolean Set the variable to different value and then try...
JavaScript typeof 运算符检查 Symbol 类型
Symbol 在 ES6 中引入,提供了一种创建唯一标识符的方法。使用 typeof 运算符与 Symbol 一起使用时,会返回 "symbol"。
typeof Symbol(); //returns "symbol"; typeof Symbol("unique values"); //returns "symbol";
示例
在下面的示例中,我们使用 typeof 运算符来检查 Symbol 数据类型。
<html> <body> <div id="output"></div> <script> let sym = Symbol("Hello"); document.getElementById("output").innerHTML = typeof sym; </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
symbol Set the variable to different value and then try...
JavaScript typeof 运算符检查 Undefined 和 Null
"undefined" 类型表示缺少值。"null" 类型表示没有任何对象值。当使用 typeof 运算符与未定义变量一起使用时,它会返回 'undefined'。令人惊讶的是,使用 typeof 运算符与 null 一起使用也会返回 "object",这是 JavaScript 中已知的怪癖。
typeof undefined; //returns "undefined"; typeof null; //returns "object";
请注意,typeof 运算符将对未声明的变量和已声明但未赋值的变量都返回 "undefined"。
示例
在下面的示例中,我们使用 typeof 运算符来检查 undefined 数据类型。
<html> <body> <div id="output"></div> <script> let x; document.getElementById("output").innerHTML = typeof x; </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
undefined Set the variable to different value and then try...
JavaScript typeof 运算符检查对象类型
对于所有类型的对象,例如 JavaScript 对象、数组、日期、正则表达式等,JavaScript typeof 运算符都返回 "object"。
const obj = {age: 23}; typeof obj; //returns "object"; const arr = [1,2,3,4]; typeof arr; //returns "object"; typeof new Date(); //returns "object"; typeof new String("Hello World"); //returns "object";
示例
在下面的示例中,我们使用 typeof 运算符来检查对象数据类型。
<html> <body> <div id="output"></div> <script> const person = {name: "John", age: 34}; document.getElementById("output").innerHTML = typeof person; </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
object Set the variable to different value and then try...
JavaScript typeof 运算符检查函数类型
函数在 JavaScript 中是头等公民。JavaScript typeof 运算符对所有类型的函数都返回 "function"。有趣的是,它对类也返回 "function"。
const myFunc = function(){return "Hello world"}; typeof myFunc; //returns "function"; const func = new Function(); typeof func; //returns "function"; class myClass {constructor() { }} typeof myClass; // returns "function";
示例
在下面的示例中,我们使用 typeof 运算符来检查函数数据类型。
<html> <body> <div id="output"></div> <script> const myFunc = function(){return "Hello world"}; document.getElementById("output").innerHTML = typeof myFunc; </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
function Set the variable to different value and then try...
JavaScript typeof 运算符检查 BigInt 类型
typeof 运算符对 BigInt 数字返回 "bigint"。BigInt 值是数值,其大小超过了 number 原语所能表示的范围。
typeof 100n; // returns "bigint"
JavaScript typeof 运算符在实时开发中的应用
例如,开发人员从 API 获取数据。如果只有一个字符串,API 返回字符串响应,对于多个字符串,API 返回字符串数组。在这种情况下,开发人员需要检查响应的类型是字符串还是数组,如果是数组,则需要遍历数组的每个字符串。
示例
在下面的示例中,我们检查 'response' 变量的类型并相应地打印其值。
<html> <body> <script> const response = ["Hello", "World!", "How", "are", "you?"]; if (typeof response == "string") { document.write("The response is - ", response); } else { document.write("The response values are : "); // Traversing the array for (let val of response) { document.write(val, " "); } } </script> </body> </html>
输出
The response values are : Hello World! How are you?
JavaScript 数组和 typeof 运算符
尽管数组在 JavaScript 中是一种对象类型,但它们在使用 typeof 运算符时具有不同的行为。
let numbers = [1, 2, 3]; typeof numbers; // Output: 'object'
使用 typeof 运算符时,数组返回 "object",因此为了精确检测数组,通常最好使用 Array.isArray()。
示例
<html> <body> <div id="output"></div> <script> let numbers = [1, 2, 3]; document.getElementById("output").innerHTML = Array.isArray(numbers); </script> <p>Set the variable to different value and then try...</p> </body> </html>
输出
true Set the variable to different value and then try..