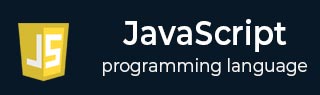
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else
- JavaScript - while 循环
- JavaScript - for 循环
- JavaScript - for...in
- Javascript - for...of
- JavaScript - 循环控制
- JavaScript - break 语句
- JavaScript - continue 语句
- JavaScript - switch case
- JavaScript - 用户自定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - String
- JavaScript - Array
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Set
- JavaScript - WeakSet
- JavaScript - Map
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标签模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxy
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步编程
- JavaScript - 异步编程
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 修改 HTML
- JavaScript - 修改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图片地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 风格指南
JavaScript - 严格模式
JavaScript中的严格模式
在 JavaScript 中,**严格模式**是在 ES5(ECMAScript 2009)中引入的。引入“严格模式”的目的是使 JavaScript 代码更安全。
“**use strict**”字面量表达式用于在 JavaScript 代码中添加严格模式。它消除了代码中的静默错误,例如,你不能在未声明的情况下使用变量,不能修改对象的只读属性等。
启用严格模式
要启用严格模式,应将以下字面量表达式写入代码的顶部:
'use strict';
“use strict”指令用于启用 JavaScript 的严格模式。
为什么要使用严格模式?
这里列出了一些使用严格 JavaScript 模式的原因:
- **错误预防** - 严格模式可以防止开发人员在编写 JavaScript 代码时常犯的一些错误,例如在未声明的情况下初始化变量或使用保留关键字作为标识符。
- **更安全的代码** - 严格模式可以防止意外创建全局变量。此外,它不允许使用“with”之类的语句,这些语句可能会导致代码漏洞。
- **未来兼容性** - 通过使用严格模式,你可以使你的代码与 JavaScript 的未来版本保持一致。例如,当前版本的 JavaScript 不包含像“public”这样的关键字,但它为将来版本保留了这些关键字。因此,严格模式从现在开始将不允许你将其用作标识符。
全局作用域中的严格模式
当你在 JavaScript 代码顶部添加“**use strict**”时;它将对整个代码使用严格模式。
示例
在下面的示例中,我们定义了变量“y”并将其初始化为 50。代码在输出中打印“y”的值。
此外,我们在未声明的情况下初始化了变量“x”。因此,它会在控制台中给出**错误**,并且不会打印输出。
简而言之,严格模式不允许你在未声明的情况下使用变量。
<html> <head> <title> Using the strict mode gloablly </title> </head> <body> <script> "use strict"; let y = 50; // This is valid document.write("The value of the X is: " + y); x = 100; // This is not valid document.write("The value of the X is: " + x); </script> </body> </html>
局部作用域中的严格模式
你也可以在特定的函数内使用“严格模式”。因此,它只会在函数作用域内应用。让我们通过一个例子来理解它。
示例
在下面的示例中,我们只在 test() 函数内部使用了“use strict”字面量。因此,它只消除了函数中的异常错误。
下面的代码允许你在函数外部在未声明的情况下初始化变量,但在函数内部则不允许。
<html> <head> <title> Using the strict mode gloablly </title> </head> <body> <script> x = 100; // This is valid document.write("The value of the X is - " + x); function test() { "use strict"; y = 50; // This is not valid document.write("The value of the y is: " + x); } test(); </script> </body> </html>
在严格模式下不应该犯的错误
1. 你不能在未声明的情况下用值初始化变量。
<script> 'use strict'; num = 70.90; // This is invalid </script>
2. 同样,你不能在未声明的情况下使用对象。
<script> 'use strict'; numObj = {a: 89, b: 10.23}; // This is invalid </script>
3. 你不能使用 delete 关键字删除对象。
<script> 'use strict'; let women = { name: "Aasha", age: 29 }; delete women; // This is invalid </script>
4. 在严格模式下,你不能删除对象原型。
<script> 'use strict'; let women = { name: "Aasha", age: 29 }; delete women.prototype; // This is invalid </script>
5. 不允许使用 delete 运算符删除函数。
<script> 'use strict'; function func() { } delete func; // This is invalid </script>
6. 你不能使用重复的参数值的函数。
<script> 'use strict'; function func(param1, param1, param2) { // Function with 2 param1 is not allowed! } </script>
7. 你不能将八进制数字赋值给变量。
<script> 'use strict'; let octal = 010; // Throws an error </script>
8. 你不能使用转义字符。
<script> 'use strict'; let octal = \010; // Throws an error </script>
9. 你不能使用保留关键字(如 eval、arguments、public 等)作为标识符。
<script> 'use strict'; let public = 100; // Throws an error </script>
10. 你不能写入对象的只读属性。
<script> 'use strict'; let person = {}; Object.defineProperty(person, 'name', { value: "abc", writable: false }); obj1.name = "JavaScript"; // throws an error </script>
11. 你不能为 getter 函数赋值。
<script> 'use strict'; let person = { get name() { return "JavaScript"; } }; obj1.name = "JavaScript"; // throws an error </script>
12. 在严格模式下,当你在函数内部使用“this”关键字时,它指的是通过其调用函数的引用对象。如果未指定引用对象,则它指的是 undefined 值。
<script> 'use strict'; function test() { console.log(this); // Undefined } test(); </script>
13. 你不能在严格模式下使用“with”语句。
<script> 'use strict'; with (Math) {x = sin(2)}; // This will throw an error </script>
14. 出于安全原因,你不能使用 eval() 函数声明变量。
<script> 'use strict'; eval("a = 8") </script>
15. 你不能使用为将来保留的关键字作为标识符。以下关键字为将来保留:
- implements
- interface
- package
- private
- protected