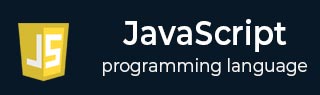
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 放置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - 删除运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - 展开运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - If...Else
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in
- Javascript - For...of
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标记模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookies
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图像地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - 鼠标事件
JavaScript 鼠标事件允许用户使用鼠标控制和交互网页。这些事件根据用户点击、滚动、拖动和其他鼠标移动触发特定的函数或操作。
要在 JavaScript 中处理鼠标事件,可以使用 addEventListener() 方法。addEventListener() 方法接受两个参数:事件类型和事件处理程序函数。事件类型是要处理的事件的名称,事件处理程序函数是在事件发生时将调用的函数。
在 Web 开发中,JavaScript 提供了一种强大的机制,可以通过一组事件来响应用户与鼠标的交互。这些事件使开发人员能够通过捕获和处理各种鼠标相关操作来创建动态和交互式的 Web 应用程序。
常见的鼠标事件
以下是 JavaScript 最常见的鼠标事件
鼠标事件 | 描述 |
---|---|
点击 | 当元素经历鼠标按钮按下时,它会触发 click 事件。 |
双击 | dblclick 事件在快速双击鼠标按钮时触发。 |
鼠标按下和鼠标抬起 | 鼠标点击的开始触发“mousedown”事件,而点击的完成导致“mouseup”事件发生。 |
鼠标移动 | 当鼠标指针移动到某个元素上时,它会触发“mousemove”事件;此事件为开发人员提供有关鼠标位置的信息。这些数据使他们能够设计以动态鼠标移动为基础的响应式界面。 |
上下文菜单 | 当用户尝试打开上下文菜单(通常通过右键单击)时,他们会触发 contextmenu 事件。此事件允许开发人员自定义或禁止上下文菜单的默认行为。 |
滚轮 | 当鼠标滚轮旋转时,它会触发“wheel event”;此特定事件通常体现在实现功能中,尤其是缩放或滚动。 |
拖放 | 拖放功能与 dragstart、dragend、dragover、dragenter、dragleave 和 drop 等事件相关。它们允许开发人员创建用于在网页内拖动元素的交互式界面。 |
示例:点击事件
在此示例中,我们演示了 click 事件。当单击按钮时,它会向控制台消息打印相应的消息,即“Clicked!”。此事件通常在提交表单时使用。
<!DOCTYPE html> <html> <head> <title>Click Event Example</title> </head> <body> <button id="clickButton">Click me!</button> <p id = "output"></p> <script> const clickButton = document.getElementById('clickButton'); const outputDiv = document.getElementById("output"); clickButton.addEventListener('click', function(event) { outputDiv.innerHTML += 'Clicked!'+ JSON.stringify(event) + "<br>"; }); </script> </body> </html>
示例:双击事件
此示例中使用 dblclick 事件,在双击指定按钮时触发。我们将事件侦听器附加到 id 为“doubleClickButton”的元素。用户双击该按钮会提示一个函数,该函数记录控制台消息,确认他们与该按钮的交互。
<!DOCTYPE html> <html> <head> <title>Double Click Event Example</title> </head> <body> <button id="doubleClickButton">Double-click me!</button> <p id = "output"></p> <script> const doubleClickButton = document.getElementById('doubleClickButton'); const outputDiv = document.getElementById("output"); doubleClickButton.addEventListener('dblclick', function(event) { outputDiv.innerHTML += 'Double-clicked!' + JSON.stringify(event) + "<br>"; }); </script> </body> </html>
示例:鼠标按下和鼠标抬起事件
此场景举例说明了 mousedown 和 mouseup 事件的使用:这两个事件都应用于一个 <div> 元素,该元素的 id 为“mouseUpDownDiv”。建立了两个不同的事件侦听器;一个响应鼠标按钮的下压动作,另一个在释放或向上移动该按钮时做出反应。按下指定的 div(mousedown)时,一条指示用户已按下鼠标按钮的消息将显示在您的控制台日志中。当用户释放鼠标按钮(mouseup)时,我们还会记录另一条消息以指示鼠标按钮已抬起。
<!DOCTYPE html> <html> <head> <title>Mouse Down and Mouse Up Events Example</title> </head> <body> <div id="mouseUpDownDiv" style="width: 600px; height: 100px; background-color: lightblue;"> Please perform mouse down and up event any where in this DIV. </div> <p id = "output"></p> <script> const mouseUpDownDiv = document.getElementById('mouseUpDownDiv'); const outputDiv = document.getElementById("output"); mouseUpDownDiv.addEventListener('mousedown', function(event) { outputDiv.innerHTML += 'Mouse button down!' + JSON.stringify(event) + "<br>"; }); mouseUpDownDiv.addEventListener('mouseup', function(event) { outputDiv.innerHTML += 'Mouse button up!' + JSON.stringify(event) + "<br>"; }); </script> </body> </html>
示例:鼠标移动事件
在本例中,我们使用 mousemove 事件来监视鼠标指针在指定 <div> 元素(id 为“mouseMoveDiv”)上的移动。处理程序函数从表示指针 X-Y 坐标的事件对象中提取 clientX 和 clientY 属性。随后,这些属性将记录到控制台中;从而提供有关用户在指定 div 区域内确切位置的实时反馈。
<!DOCTYPE html> <html> <head> <title>Mouse Move Event Example</title> </head> <body> <div id="mouseMoveDiv" style="width: 600px; height: 200px; background-color: lightgreen;"> Please move you mouse inside this DIV.</div> <p id = "output"></p> <script> const mouseMoveDiv = document.getElementById('mouseMoveDiv'); const outputDiv = document.getElementById("output"); mouseMoveDiv.addEventListener('mousemove', function(event) { const x = event.clientX; const y = event.clientY; outputDiv.innerHTML += `Mouse moved to (${x}, ${y})` + JSON.stringify(event) + "<br>"; }); </script> </body> </html>
示例:滚轮事件
此示例展示了 wheel 事件,在鼠标滚轮旋转时激活。事件侦听器附加到 id 为“wheelDiv”的 <div> 元素。当用户在该 div 上旋转鼠标滚轮时,关联的函数会向控制台记录一条消息,指示鼠标滚轮已旋转。
<!DOCTYPE html> <html> <head> <title>Wheel Event Example</title> </head> <body> <div id="wheelDiv" style="width: 600px; height: 200px; background-color: palevioletred;"> Please bring the curser inside this DIV and rotate the wheel of mouse.</div> <p id = "output"></p> <script> const wheelDiv = document.getElementById('wheelDiv'); const outputDiv = document.getElementById("output"); wheelDiv.addEventListener('wheel', function(event) { outputDiv.innerHTML += 'Mouse wheel rotated!'+ event + "<br>"; }); </script> </body> </html>