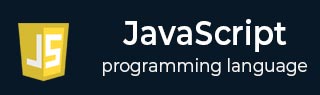
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else
- JavaScript - while 循环
- JavaScript - for 循环
- JavaScript - for...in
- Javascript - for...of
- JavaScript - 循环控制
- JavaScript - break 语句
- JavaScript - continue 语句
- JavaScript - switch case
- JavaScript - 用户自定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - String
- JavaScript - Array
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Set
- JavaScript - WeakSet
- JavaScript - Map
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标记模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxy
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步编程
- JavaScript - 异步编程
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promisification
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 修改 HTML
- JavaScript - 修改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面跳转
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图片地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - Fetch API
什么是 Fetch API?
JavaScript Fetch API 是一个 Web API,允许 Web 浏览器向 Web 服务器发出 HTTP 请求。在 JavaScript 中,Fetch API 在 ES6 版本中引入。它是 XMLHttpRequest (XHR) 对象的替代方案,用于向服务器发出 'GET'、'POST'、'PUT' 或 'DELETE' 请求。
浏览器的 window 对象默认包含 Fetch API。
Fetch API 提供了 fetch() 方法,可用于异步访问网络上的资源。
fetch() 方法允许你向服务器发出请求,并返回一个 Promise。之后,你需要解析 Promise 以获取从服务器接收的响应。
语法
你可以按照以下语法在 JavaScript 中使用 fetch() API:
window.fetch(URL, [options]); OR fetch(URL, [options]);
参数
fetch() 方法接受两个参数。
URL − 你需要发出请求的 API 端点。
[options] − 这是一个可选参数。它是一个对象,包含方法、标头等作为键。
返回值
它返回一个 Promise,你可以使用 'then...catch' 块或异步方式来解决。
使用 'then...catch' 块处理 Fetch() API 响应
JavaScript fetch() API 返回一个 Promise,你可以使用 'then...catch' 块来处理它。
按照以下语法使用带有 'then...catch' 块的 fetch() API。
fetch(URL) .then(data => { // Handle data }) .catch(err=> { // Handle error })
在上面的语法中,你可以在 'then' 块中处理 'data',在 'catch' 块中处理错误。
示例
在下面的代码中,我们使用 fetch() API 从给定的 URL 获取数据。它返回一个 Promise,我们使用 'then' 块来处理。
首先,我们将数据转换为 JSON 格式。之后,我们将数据转换为字符串并在网页上打印出来。
<html> <body> <div id = "output"> </div> <script> const output = document.getElementById('output'); const URL = 'https://jsonplaceholder.typicode.com/todos/5'; fetch(URL) .then(res => res.json()) .then(data => { output.innerHTML += "The data from the API is: " + "<br>"; output.innerHTML += JSON.stringify(data); }); </script> </body> </html>
输出
The data from the API is: {"userId":1,"id":5,"title":"laboriosam mollitia et enim quasi adipisci quia provident illum","completed":false}
异步处理 Fetch() API 响应
你也可以使用 async/await 关键字异步地解决 fetch() API 返回的 Promise。
语法
用户可以按照以下语法使用 async/await 关键字和 fetch() API。
let data = await fetch(URL); data = await data.json();
在上面的语法中,我们使用 'await' 关键字来停止代码执行,直到 Promise 完成。之后,我们再次使用带有 data.json() 的 'await' 关键字来停止函数的执行,直到它将数据转换为 JSON 格式。
示例
在下面的代码中,我们定义了 getData() 异步函数,使用 fetch() API 从给定的 URL 获取待办事项列表数据。之后,我们将数据转换为 JSON 并将输出打印到网页上。
<html> <body> <div id = "output"> </div> <script> async function getData() { let output = document.getElementById('output'); let URL = 'https://jsonplaceholder.typicode.com/todos/6'; let data = await fetch(URL); data = await data.json(); output.innerHTML += "The data from the API is: " + "<br>"; output.innerHTML += JSON.stringify(data); } getData(); </script> </body> </html>
输出
The data from the API is: {"userId":1,"id":6,"title":"qui ullam ratione quibusdam voluptatem quia omnis","completed":false}
Fetch() API 的选项
你也可以将包含选项作为键值对的对象作为第二个参数传递。
语法
按照以下语法将选项传递给 Fetch() API。
fetch(URL, { method: "GET", body: JSON.stringify(data), mode: "no-cors", cache: "no-cache", credentials: "same-origin", headers: { "Content-Type": "application/json", }, redirect: "follow", })
选项
这里,我们提供了一些要传递给 fetch() API 的选项。
method − 它根据你想要发出哪种类型的请求,取值为 'GET'、'POST'、'PUT' 和 'DELETE' 方法。
body − 用于以字符串格式传递数据。
mode − 出于安全原因,它取值为 'cors'、'no-cors'、'same-origin' 等。
cache − 它取值为 '*default'、'no-cache'、'reload' 等。
credentials − 它取值为 'same-origin'、'omit' 等。
headers − 你可以使用此属性将标头传递到请求中。
redirect − 如果你希望用户在请求完成之后重定向到其他网页,可以使用 redirect 属性。
示例:发出 GET 请求
在下面的代码中,我们将“GET”方法作为选项传递给了 fetch() API。
Fetch() API 从给定的 API 端点获取数据。
<html> <body> <div id = "output"> </div> <script> let output = document.getElementById("output"); let options = { method: 'GET', } let URL = "https://dummy.restapiexample.com/api/v1/employee/2"; fetch(URL, options) .then(res => res.json()) .then(res => { output.innerHTML += "The status of the response is - " + res.status + "<br>"; output.innerHTML += "The message returned from the API is - " + res.message + "<br>"; output.innerHTML += "The data returned from the API is - " + JSON.stringify(res.data); }) .catch(err => { output.innerHTML += "The error returned from the API is - " + JSON.stringify(err); }) </script> </body> </html>
输出
The status of the response is - success The message returned from the API is - Successfully! Record has been fetched. The data returned from the API is - {"id":2,"employee_name":"Garrett Winters","employee_salary":170750,"employee_age":63,"profile_image":""}
示例(发出 POST 请求)
在下面的代码中,我们创建了一个包含 emp_name 和 emp_age 属性的 employee 对象。
此外,我们创建了一个包含 method、headers 和 body 属性的 options 对象。我们将 'POST' 用作 method 的值,并将 employee 对象转换为字符串后用作 body 值。
我们使用 fetch() API 向 API 端点发出 POST 请求以插入数据。发出 POST 请求后,您可以在网页上看到响应。
<html> <body> <div id = "output"> </div> <script> let output = document.getElementById("output"); let employee = { "emp_name": "Sachin", "emp_age": "30" } let options = { method: 'POST', // To make a post request headers: { 'Content-Type': 'application/json;charset=utf-8' }, body: JSON.stringify(employee) } let URL = "https://dummy.restapiexample.com/api/v1/create"; fetch(URL, options) .then(res => res.json()) // Getting response .then(res => { output.innerHTML += "The status of the request is : " + res.status + "<br>"; output.innerHTML += "The message returned from the API is : " + res.message + "<br>"; output.innerHTML += "The data added is : " + JSON.stringify(res.data); }) .catch(err => { output.innerHTML += "The error returned from the API is : " + JSON.stringify(err); }) </script> </body> </html>
输出
The status of the request is : success The message returned from the API is : Successfully! Record has been added. The data added is : {"emp_name":"Sachin","emp_age":"30","id":7425}
示例(发出 PUT 请求)
在下面的代码中,我们创建了 'animal' 对象。
此外,我们创建了 options 对象。在 options 对象中,我们添加了 'PUT' 方法、headers 和 animal 对象作为 body。
之后,我们使用 fetch() API 更新给定 URL 上的数据。您可以看到成功更新数据的输出响应。
<html> <body> <div id = "output"> </div> <script> let output = document.getElementById("output"); let animal = { "name": "Lion", "color": "Yellow", "age": 10 } let options = { method: 'PUT', // To Update the record headers: { 'Content-Type': 'application/json;charset=utf-8' }, body: JSON.stringify(animal) } let URL = "https://dummy.restapiexample.com/api/v1/update/3"; fetch(URL, options) .then(res => res.json()) // Getting response .then(res => { console.log(res); output.innerHTML += "The status of the request is : " + res.status + "<br>"; output.innerHTML += "The message returned from the API is : " + res.message + "<br>"; output.innerHTML += "The data added is : " + JSON.stringify(res.data); }) .catch(err => { output.innerHTML += "The error returned from the API is : " + JSON.stringify(err); }) </script> </body> </html>
输出
The status of the request is : success The message returned from the API is : Successfully! Record has been updated. The data added is : {"name":"Lion","color":"Yellow","age":10}
示例(发出 DELETE 请求)
在下面的代码中,我们向给定的 URL 发出 'DELETE' 请求,以使用 fetch() API 删除特定数据。它返回包含成功消息的响应。
<html> <body> <div id = "output"> </div> <script> const output = document.getElementById("output"); let options = { method: 'DELETE', // To Delete the record } let URL = " https://dummy.restapiexample.com/api/v1/delete/2"; fetch(URL, options) .then(res => res.json()) // After deleting data, getting response .then(res => { output.innerHTML += "The status of the request is : " + res.status + "<br>"; output.innerHTML += "The message returned from the API is : " + res.message + "<br>"; output.innerHTML += "The data added is - " + JSON.stringify(res.data); }) .catch(err => { output.innerHTML += "The error returned from the API is : " + JSON.stringify(err); }) </script> </body> </html>
输出
The status of the request is : success The message returned from the API is : Successfully! Record has been deleted The data added is - "2"
使用 Fetch() API 的优势
以下是使用 fetch() API 与第三方软件或 Web 服务器交互的一些好处。
简单的语法 − 它提供了一种简单的语法来向服务器发出 API 请求。
基于 Promise − 它返回 Promise,您可以使用 'then...catch' 块或 'async/await' 关键字异步地解决它。
JSON 处理 − 它具有将字符串响应数据转换为 JSON 数据的内置功能。
选项 − 您可以使用 fetch() API 向请求传递多个选项。